Prompt
Answer
Problem Analysis
The provided code implements the merge sort algorithm to sort an array in ascending order. The algorithm divides the array into two halves, recursively sorts each half, and then merges the two sorted halves. The current implementation modifies the original array in place.
Data Structure Selection
For efficient handling of sorting operations with minimal modification of the original array, a suitable data structure would be an auxiliary array. This auxiliary array would be used to store intermediate results during the merging process, allowing for a more controlled and predictable sorting process without directly modifying the input array.
Template Generation
Below is a language-agnostic template for implementing the merge sort algorithm using an auxiliary array:
1. Initialize an auxiliary array of the same size as the input array.
2. Implement the merge_sort function with the following steps:
a. Divide the array into two halves.
b. Recursively call merge_sort on each half.
c. Merge the sorted halves into the auxiliary array.
d. Copy the merged results back to the original array.
3. Implement the merge function to merge two sorted halves efficiently.
Pseudocode:
function merge_sort(arr, aux_arr, low, high):
if low < high:
mid = low + (high - low) / 2
merge_sort(arr, aux_arr, low, mid)
merge_sort(arr, aux_arr, mid + 1, high)
merge(arr, aux_arr, low, mid, high)
function merge(arr, aux_arr, low, mid, high):
Copy arr[low:high+1] into aux_arr[low:high+1]
Initialize pointers i, j, k
Merge the two halves back into arr
arr = [12, 11, 13, 5, 6, 7]
aux_arr = [0]*len(arr)
merge_sort(arr, aux_arr, 0, len(arr)-1)
print("Given array is", arr)
print("Sorted array is", arr)
Description and Documentation
- Auxiliary Array: An auxiliary array is utilized to store intermediate results during the merging process. This helps in preserving the original array and ensures a stable sorting process.
- Merge Sort Algorithm: The merge sort algorithm is optimal for sorting large arrays efficiently with a time complexity of O(n log n) in the average and worst cases.
- Pseudocode Explanation: The provided pseudocode outlines a structured approach to implementing merge sort with an auxiliary array, making it easier to adapt to different programming languages while preserving the original array.
Description
Implementing merge sort algorithm using an auxiliary array to efficiently sort an array while minimizing modifications to the original array. Provides structured pseudocode template for implementation.
More Data Structure Designers
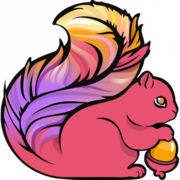
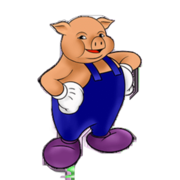
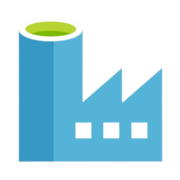
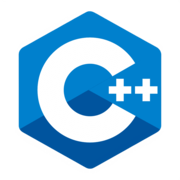
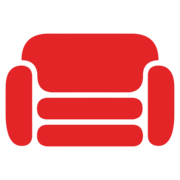
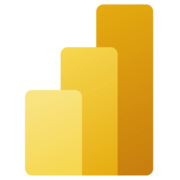
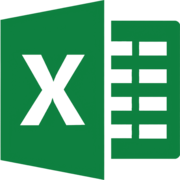
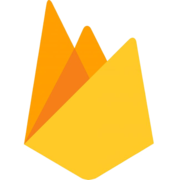
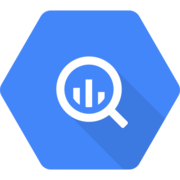
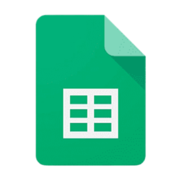
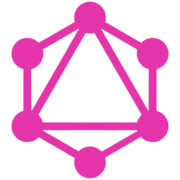
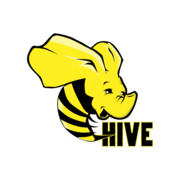
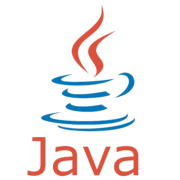
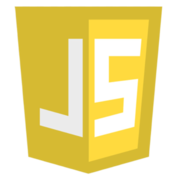
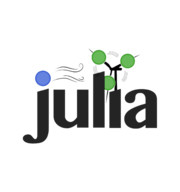
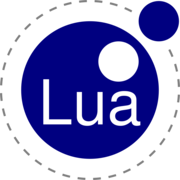
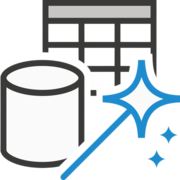
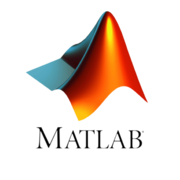
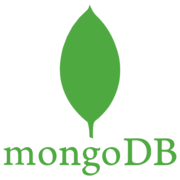
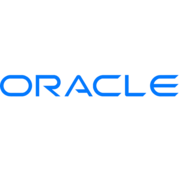
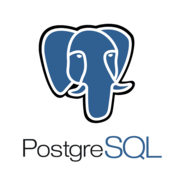
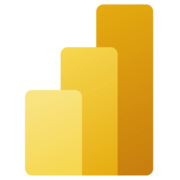
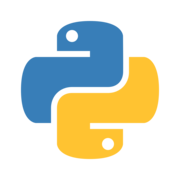
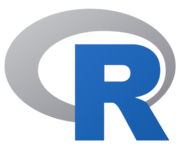
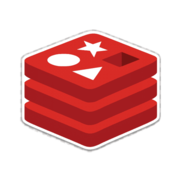
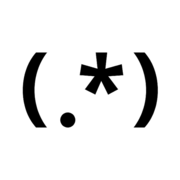
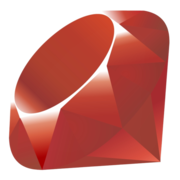
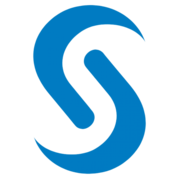
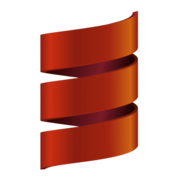
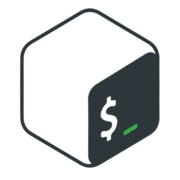
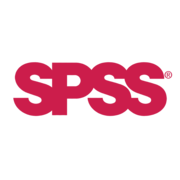
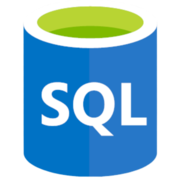
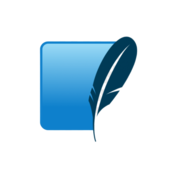
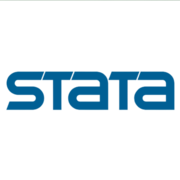
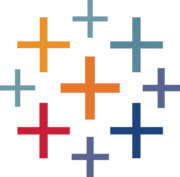
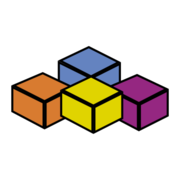