Variable Namer
Naming Strategy for JavaScript Class Interacting with Flask Server
This guide provides naming suggestions for a JavaScript class that interacts with a Flask server, including class name, method name, and variable names. The example code snippet demonstrates the suggested names in action, promoting code

Prompt
Answer
Naming Strategy for JavaScript Class Interacting with Flask Server
Class Name
The class handles data fetching from a Flask server. Hence, a name that indicates this purpose is appropriate.
Suggested Name:
ServerDataFetcher
Method Name
The method is responsible for making fetch requests to the Flask server. A name that reflects this specific action is essential.
Suggested Name:
fetchData
Variable Names
Within the method, variables should clearly indicate their role or the data they hold.
Suggested Variables:
url
: Represents the endpoint URL to which the request is made.requestOptions
: Defines options like method, headers, and body for the fetch request.response
: Holds the response received from the server.data
: Contains the parsed JSON data from the response.
Example Code Snippet
// JavaScript Class for fetching data from a Flask server
class ServerDataFetcher {
/**
* Method to fetch data from the Flask server
* @param {string} endpoint - The API endpoint to send the request to
* @returns {Promise
Explanation
ServerDataFetcher
: Clearly indicates the class is designed for fetching data from a server.fetchData
: Represents the action of fetching data, suitable for a method performing this task.url
: Clearly defines the target endpoint for the request.requestOptions
: Self-explanatory, encapsulates options for the fetch request.response
: The returned result from the server, ensuring readability.data
: Represents the processed response, easily understood in context.
Contextual Alignment
- The names follow conventions for JavaScript, making the functionality clear and maintainable.
- Aligned with typical RESTful structure, facilitating understanding across teams.
For further learning on improving code readability and maintainability, the Enterprise DNA Platform offers comprehensive courses.
Output
This naming strategy aims to enhance clarity, making the code base easier to understand and work with for future developers.
Description
This guide provides naming suggestions for a JavaScript class that interacts with a Flask server, including class name, method name, and variable names. The example code snippet demonstrates the suggested names in action, promoting code readability and maintainability.
More Variable Namers
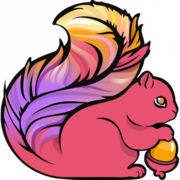
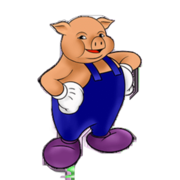
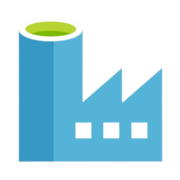
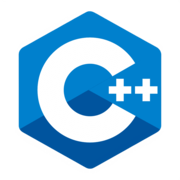
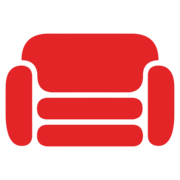
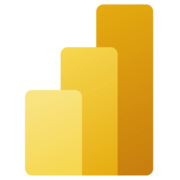
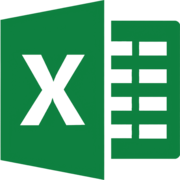
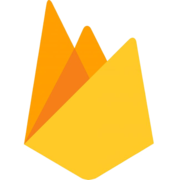
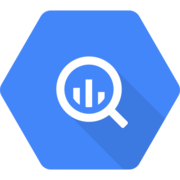
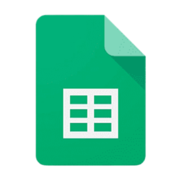
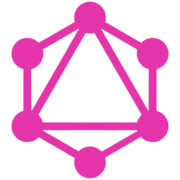
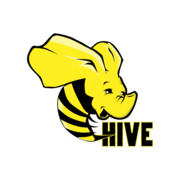
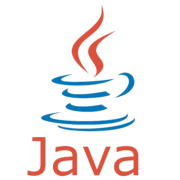
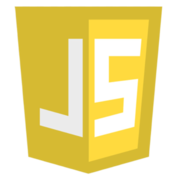
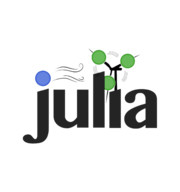
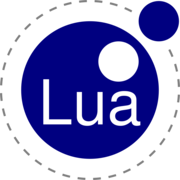
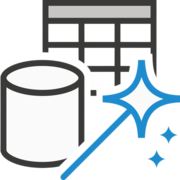
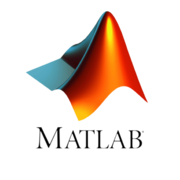
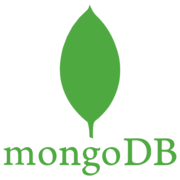
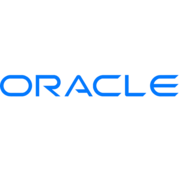
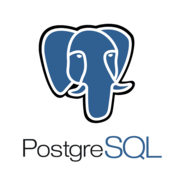
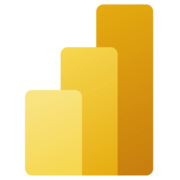
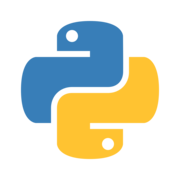
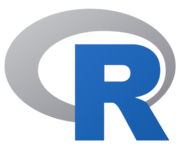
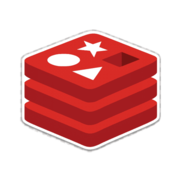
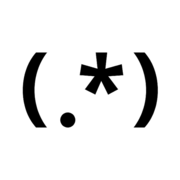
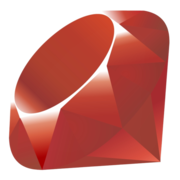
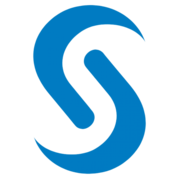
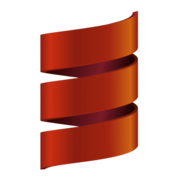
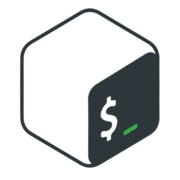
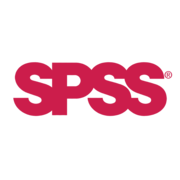
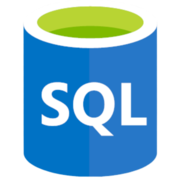
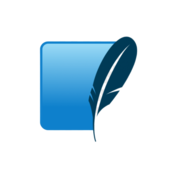
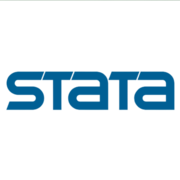
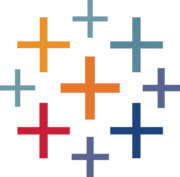
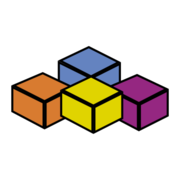