Prompt
Answer
1. Clarifying the User's Requirement:
The user wants to create SQL queries to perform the following tasks:
- Validate that an SQL query calculates the number of words in each row of a table.
- Find all words that occur in the "Texts" table more than 10 times.
2. Identifying Key Elements:
To accomplish these tasks, we need to:
- Extract words from text (considering words are alphanumeric strings separated by spaces).
- Count the occurrences of each word in the "Texts" table.
- Filter out words that occur more than 10 times.
3. Constructing the Regular Expression:
SQL Query to Calculate the Number of Words in Each Row:
SELECT
id,
text_column,
(LENGTH(text_column) - LENGTH(REPLACE(text_column, ' ', '')) + 1) as word_count
FROM table_name;
SQL Query to Find Words Occurring More Than 10 Times:
SELECT word
FROM (
SELECT word, COUNT(*) as word_count
FROM (
SELECT
REGEXP_SUBSTR(text_column, '\w+', 1, level) as word
FROM table_name
CONNECT BY REGEXP_SUBSTR(text_column, '\w+', 1, level) IS NOT NULL
)
GROUP BY word
)
WHERE word_count > 10;
4. Ensuring Flexibility and Efficiency:
- The regex used for extracting words (\w+) covers alphanumeric characters and underscores.
- The queries are structured to efficiently calculate word counts and filter words occurring more than 10 times.
5. Testing and Validation:
- Test the queries on sample data to ensure they accurately calculate word counts and identify words occurring more than 10 times.
6. Providing a Clear Explanation:
- The first query uses a combination of LENGTH and REPLACE functions to count the number of words in each row.
- The second query employs REGEXP_SUBSTR to extract words, and nested queries to count and filter words occurring more than 10 times.
7. Offering Usage Guidance:
- Substitute "table_name" with the actual table name containing the text data.
- Adjust the column names ("text_column", "id") in the queries based on the schema of the "Texts" table.
Description
Learn to construct SQL queries to calculate word counts in text data, and find words occurring more than 10 times in a specific table.
More Regex Generators
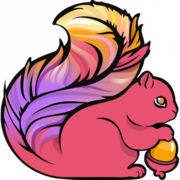
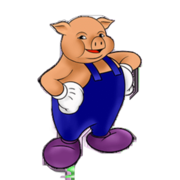
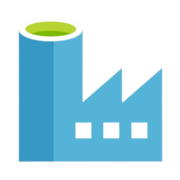
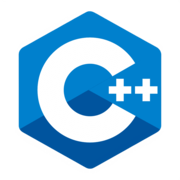
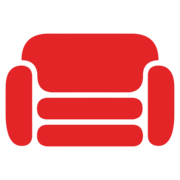
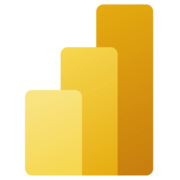
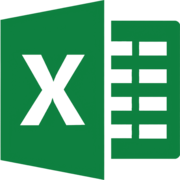
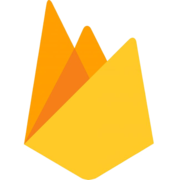
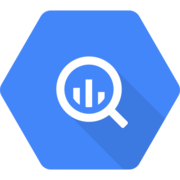
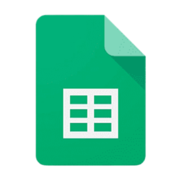
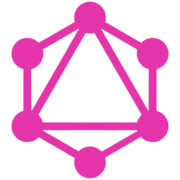
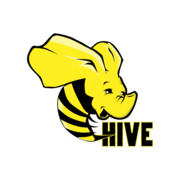
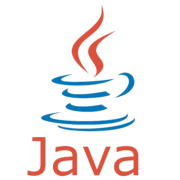
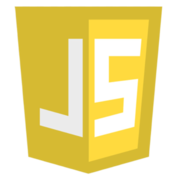
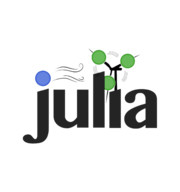
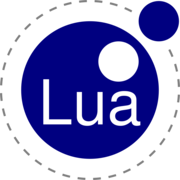
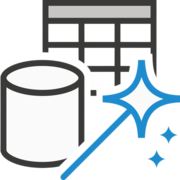
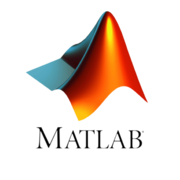
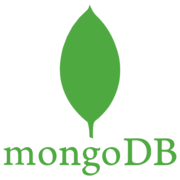
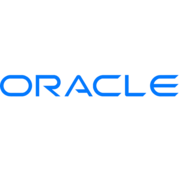
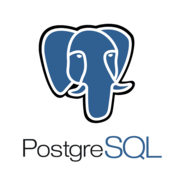
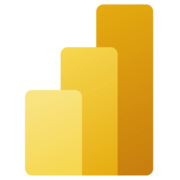
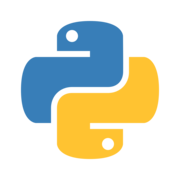
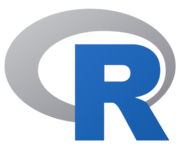
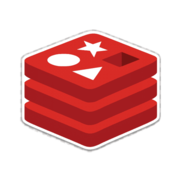
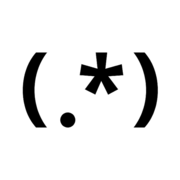
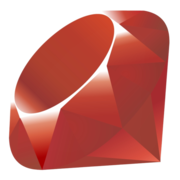
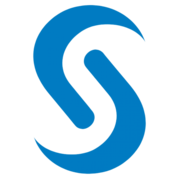
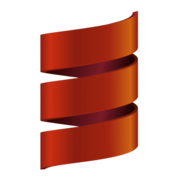
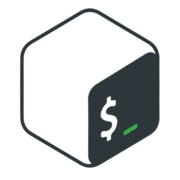
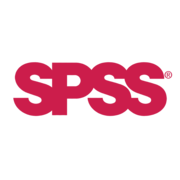
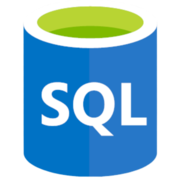
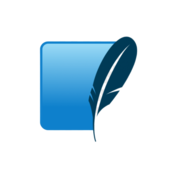
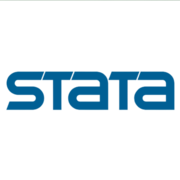
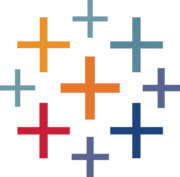
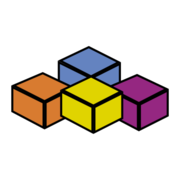