This Query related with thread "C Integer Processing Program Overview "
Prompt
Answer
Documentation for Function: write_into_file
Function Signature
int write_into_file(char *filepath, int *ps, int *pe);
Purpose
The write_into_file
function is designed to write data into a specific file. The data to be written is provided through pointers to two integer values (ps
and pe
).
Parameters
filepath
(char*
):- Description: A string representing the path to the file where data should be written.
- Expected Value: A null-terminated string.
- Usage: Used to open the file at the specified path for writing data.
ps
(int*
):- Description: Pointer to the start integer value.
- Expected Value: A valid pointer to an integer.
- Usage: The integer value pointed to by
ps
is part of the data written into the file.
pe
(int*
):- Description: Pointer to the end integer value.
- Expected Value: A valid pointer to an integer.
- Usage: The integer value pointed to by
pe
is part of the data written into the file.
Return Type
int
- Returns
0
on successful write operation. - Returns
-1
if there is an error during the file operation (such as failure to open the file or write data).
- Returns
Description
- This function attempts to open the file specified by
filepath
in write mode. - It writes the integer values pointed to by
ps
andpe
into the file. - If any step in this process fails (such as the file cannot be opened), the function returns
-1
. - If successful, the function returns
0
.
Example Usage
int main() {
char *filepath = "output.txt";
int start_value = 10;
int end_value = 20;
int result = write_into_file(filepath, &start_value, &end_value);
if (result == 0) {
printf("Data written successfully.\n");
} else {
printf("Failed to write data.\n");
}
return 0;
}
Inline Comments
Below is the function with detailed inline comments explaining the key steps:
#include
int write_into_file(char *filepath, int *ps, int *pe) {
FILE *file;
// Attempt to open the file in write mode
file = fopen(filepath, "w");
if (file == NULL) {
// Return -1 if file cannot be opened
return -1;
}
// Write the start value to the file
if (fprintf(file, "%d\n", *ps) < 0) {
// Close the file if writing failed
fclose(file);
return -1;
}
// Write the end value to the file
if (fprintf(file, "%d\n", *pe) < 0) {
// Close the file if writing failed
fclose(file);
return -1;
}
// Close the file after writing
fclose(file);
// Return 0 to indicate success
return 0;
}
Detailed Explanation
Opening the File:
- The function uses
fopen
to open the file specified byfilepath
in write mode ("w"
). - If
fopen
returnsNULL
, the file could not be opened (possibly due to permissions or a non-existent path), and the function returns-1
.
- The function uses
Writing Data:
- The function uses
fprintf
to write the integer values pointed to byps
andpe
into the file. - Each
fprintf
call writes an integer followed by a newline character (\n
). - If
fprintf
fails (returns a negative value), the file is closed usingfclose
, and the function returns-1
.
- The function uses
Closing the File:
- After successfully writing the data, the function closes the file using
fclose
. - The function returns
0
to indicate that the operation was successful.
- After successfully writing the data, the function closes the file using
Description
The write_into_file
function saves two integer values to a specified file path. It accepts the file path and pointers to the integers, returning a success or error status based on the outcome of the file operations.
More Documentation Generators
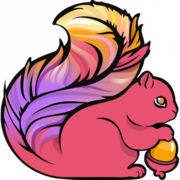
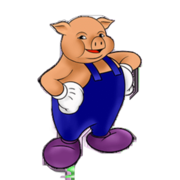
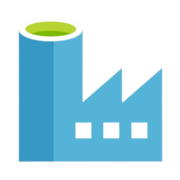
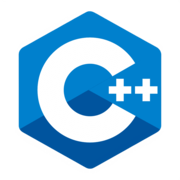
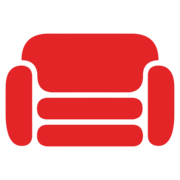
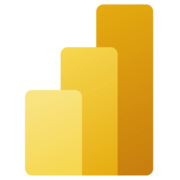
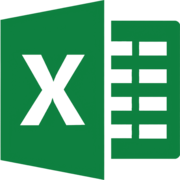
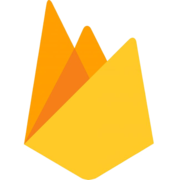
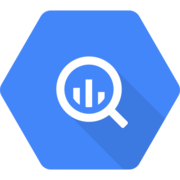
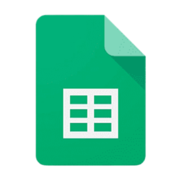
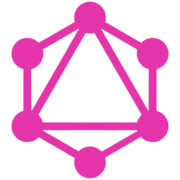
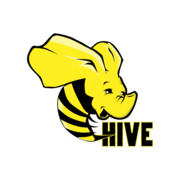
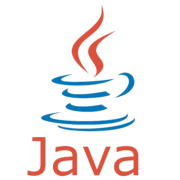
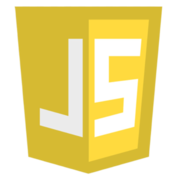
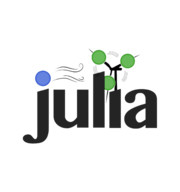
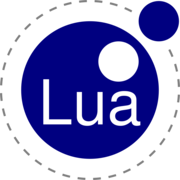
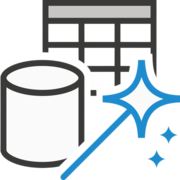
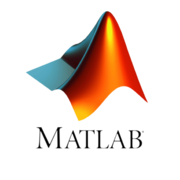
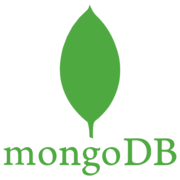
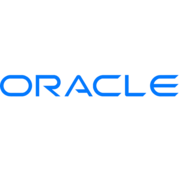
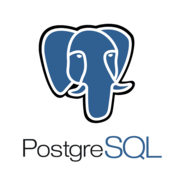
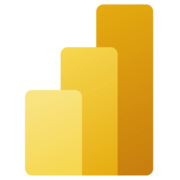
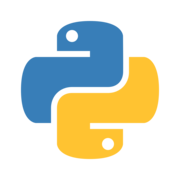
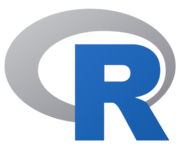
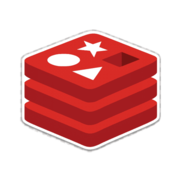
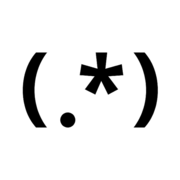
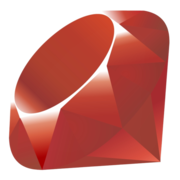
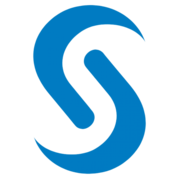
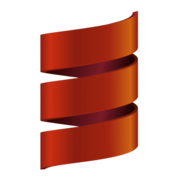
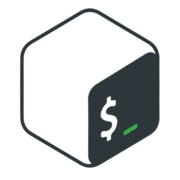
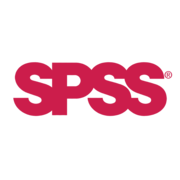
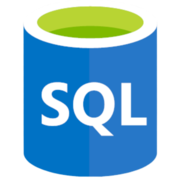
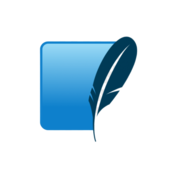
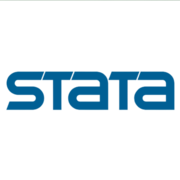
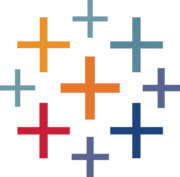
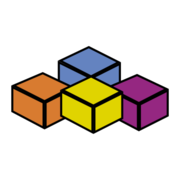