Prompt
Answer
Code Snippet Analysis
The provided code snippet consists of a simple Java class named HelloWorld
with a main method that prints out "Hello, World!" to the console.
Test Case Development
Test Case 1: TestPrintHelloWorld
- Description: Verify that the program prints "Hello, World!" to the console.
- Test Steps:
- Redirect standard output to a ByteArrayOutputStream.
- Execute the main method of HelloWorld.
- Capture the output from ByteArrayOutputStream.
- Assertion:
- Assert that the output matches "Hello, World!".
Test Case 2: TestArgsInput
- Description: Test the behavior of the program with input arguments.
- Test Steps:
- Pass input arguments to the main method.
- Redirect standard output to a ByteArrayOutputStream.
- Execute the main method of HelloWorld with input arguments.
- Capture the output from ByteArrayOutputStream.
- Assertion:
- Assert that the output matches "Hello, World!".
Test Case 3: TestEmptyArgsInput
- Description: Test the program behavior with empty input arguments.
- Test Steps:
- Pass empty arguments to the main method.
- Redirect standard output to a ByteArrayOutputStream.
- Execute the main method of HelloWorld with empty input arguments.
- Capture the output from ByteArrayOutputStream.
- Assertion:
- Assert that the output matches "Hello, World!".
Assertion Implementation
Java Unit Tests
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
import java.io.ByteArrayOutputStream;
import java.io.PrintStream;
public class HelloWorldTest {
@Test
public void testPrintHelloWorld() {
// Redirect standard output to a ByteArrayOutputStream
ByteArrayOutputStream outContent = new ByteArrayOutputStream();
System.setOut(new PrintStream(outContent));
// Execute the main method of HelloWorld
HelloWorld.main(new String[0]);
// Capture the output from ByteArrayOutputStream
String expectedOutput = "Hello, World!\n";
assertEquals(expectedOutput, outContent.toString());
}
@Test
public void testArgsInput() {
// Redirect standard output to a ByteArrayOutputStream
ByteArrayOutputStream outContent = new ByteArrayOutputStream();
System.setOut(new PrintStream(outContent));
// Execute the main method of HelloWorld with input arguments
HelloWorld.main(new String[]{"arg1", "arg2"});
// Capture the output from ByteArrayOutputStream
String expectedOutput = "Hello, World!\n";
assertEquals(expectedOutput, outContent.toString());
}
@Test
public void testEmptyArgsInput() {
// Redirect standard output to a ByteArrayOutputStream
ByteArrayOutputStream outContent = new ByteArrayOutputStream();
System.setOut(new PrintStream(outContent));
// Execute the main method of HelloWorld with empty input arguments
HelloWorld.main(new String[0]);
// Capture the output from ByteArrayOutputStream
String expectedOutput = "Hello, World!\n";
assertEquals(expectedOutput, outContent.toString());
}
}
Summary
The unit tests provided cover essential functionality by ensuring the program prints "Hello, World!" correctly. The tests also consider scenarios with input arguments and empty arguments to validate the program's behavior in different situations. By implementing these tests and assertions, developers can confidently verify the correctness and reliability of the HelloWorld
class in Java.
Description
Develops test cases to validate the HelloWorld Java class, including printing "Hello, World!" and handling input arguments, ensuring program functionality. Provides Java unit tests for verification.
More Unit Test Writers
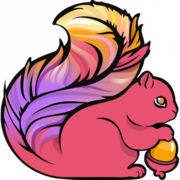
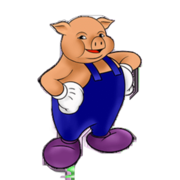
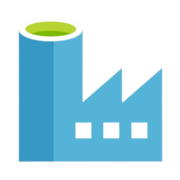
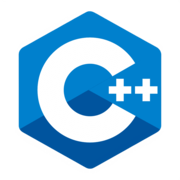
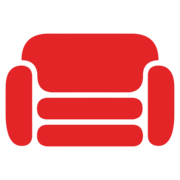
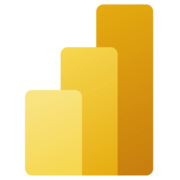
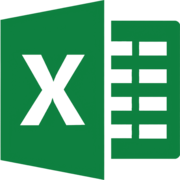
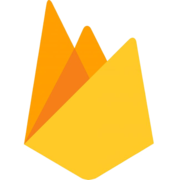
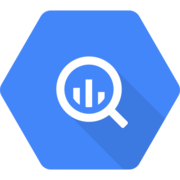
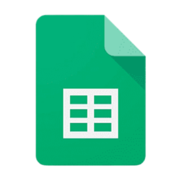
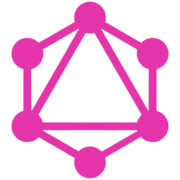
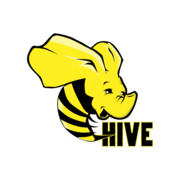
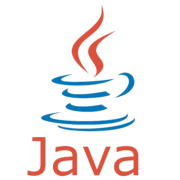
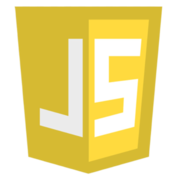
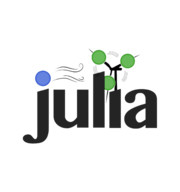
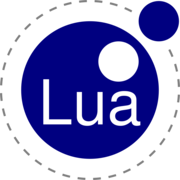
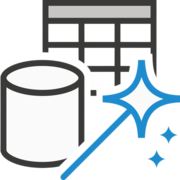
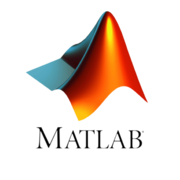
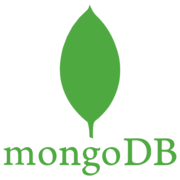
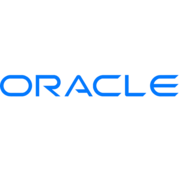
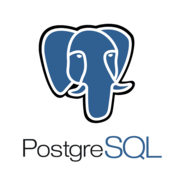
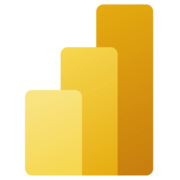
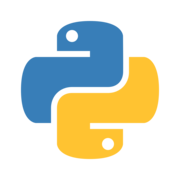
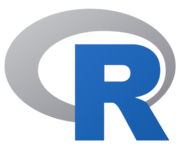
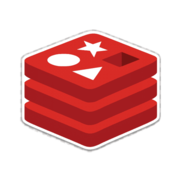
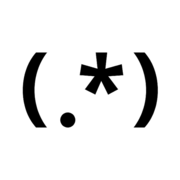
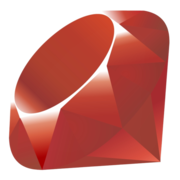
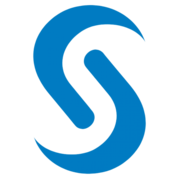
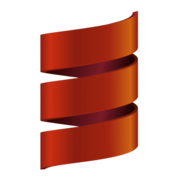
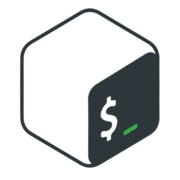
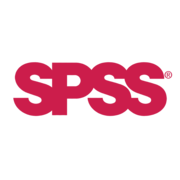
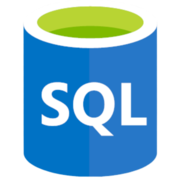
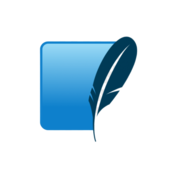
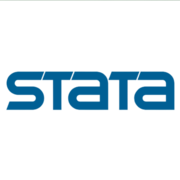
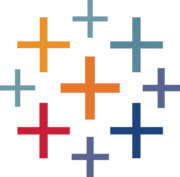
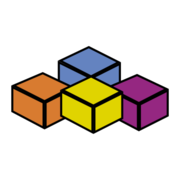