Prompt
Answer
Complexity Analysis of Given C Code
Code
#include
int main(void) {
int n;
scanf("%d", &n);
int arr[n][n];
for (int i = 0; i < n; i = i + 1){
int k = 0;
if (i % 2 == 0) k = 1;
if (i % 2 != 0) k = n;
for(int j = 0; j < n; j = j + 1){
arr[i][j] = k;
if (i % 2 == 0) k++;
else k--;
}
}
for (int i = 0; i < n; i = i + 1){
for(int j = 0; j < n; j = j + 1){
printf("%d ", arr[i][j]);
}
printf("\n");
}
return 0;
}
Time Complexity
Initialization of the Array (int arr[n][n]
)
- Operation: Allocation of an
n x n
2D array. - Complexity: This can be considered as O(1) in terms of operation, but typically ignored in complexity analysis since it doesn't involve loops.
First Nested Loop (Filling Array)
for (int i = 0; i < n; i = i + 1) {
int k = 0;
if (i % 2 == 0) k = 1;
if (i % 2 != 0) k = n;
for(int j = 0; j < n; j = j + 1) {
arr[i][j] = k;
if (i % 2 == 0) k++;
else k--;
}
}
- Here, we have a nested loop structure; the outer loop runs
n
times. - The inner loop also runs
n
times for each iteration of the outer loop. - Thus, the total number of iterations equals n * n, making this part of the code have a time complexity of O(n^2).
Second Nested Loop (Printing Array)
for (int i = 0; i < n; i = i + 1) {
for(int j = 0; j < n; j = j + 1) {
printf("%d ", arr[i][j]);
}
printf("\n");
}
- Similarly, the outer loop runs
n
times. - The inner loop also runs
n
times for each iteration of the outer loop. - Thus, the total number of iterations for this nested loop is also n * n, giving this part a time complexity of O(n^2).
Overall Time Complexity
- Combining both nested loops, the overall time complexity remains dominated by O(n^2) as they are sequential operations.
Space Complexity
Array Storage
- The primary storage requirement is for the
arr[n][n]
2D array. - This requires space proportional to n * n elements.
- Hence, the space complexity for the array storage is O(n^2).
Other Variables
- The other variables used (
n
,i
,j
,k
) are all of constant space. - Since the constant space is negligible, the additional space required does not affect the overall space complexity.
Overall Space Complexity
- The overall space complexity of the code is O(n^2) due to the 2D array storage.
Summary
- Time Complexity: O(n^2)
- Filling Array: O(n^2)
- Printing Array: O(n^2)
- Space Complexity: O(n^2)
- Array Storage: O(n^2)
Potential Bottlenecks and Optimizations
- The primary bottleneck is the nested loops manipulating and printing an n x n array.
- Optimization Insight: Given the problem's need to fill and print the entire array, reducing time complexity beyond O(n^2) for these specific operations is generally infeasible.
For further learning and deeper understanding of programming concepts, consider checking out additional courses on the Enterprise DNA platform.
Description
An in-depth analysis of a C program filling and printing a 2D array with time and space complexity evaluation.
More Complexity Estimators
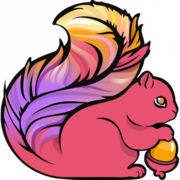
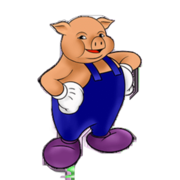
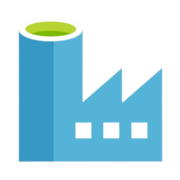
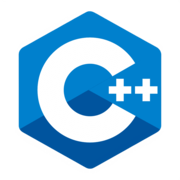
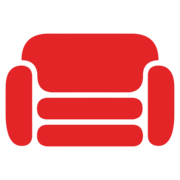
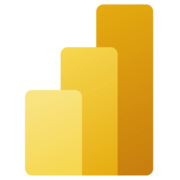
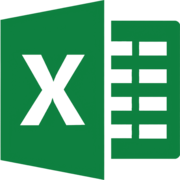
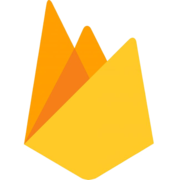
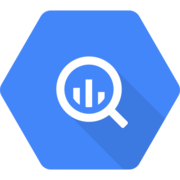
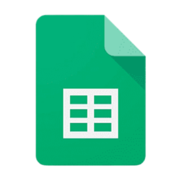
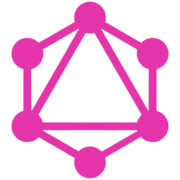
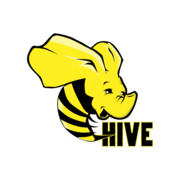
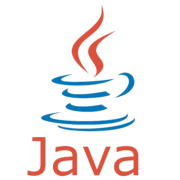
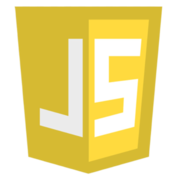
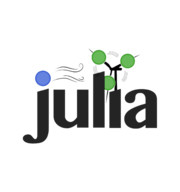
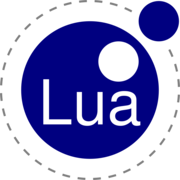
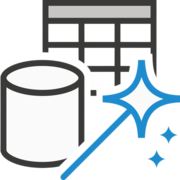
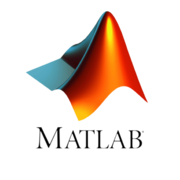
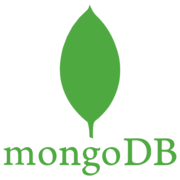
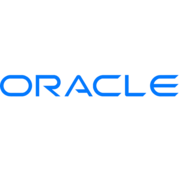
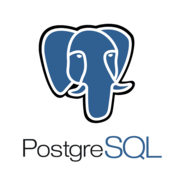
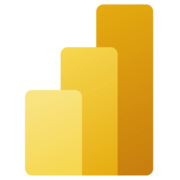
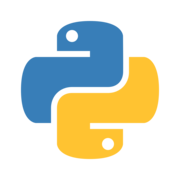
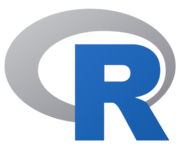
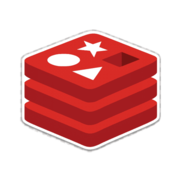
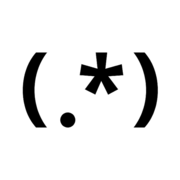
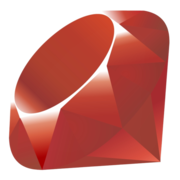
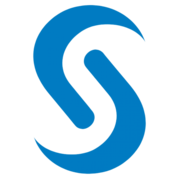
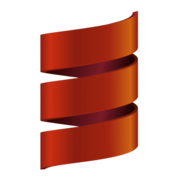
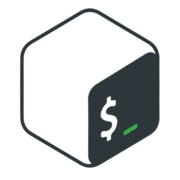
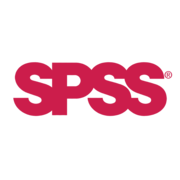
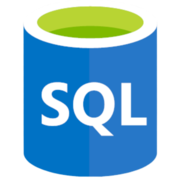
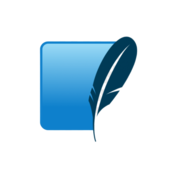
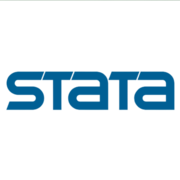
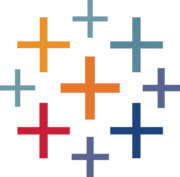
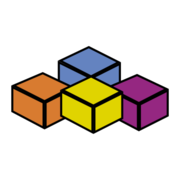