Prompt
Answer
Complexity Analysis of findMin
Function
Code Inspection
Below is the provided code snippet for a function called findMin
:
def findMin(nums: list[int]) -> int:
index = 0
minimum = nums[index]
if len(nums) > 1:
nums.pop(index)
minimums = []
for i in nums:
if minimum > i:
minimums.append(i)
if len(minimums) != 0:
return findMin(minimums)
return minimum
Time Complexity Analysis
Step-by-Step Breakdown
Initialization:
index = 0 minimum = nums[index]
This is O(1) since accessing a list element and assignment are constant-time operations.
Checking Length:
if len(nums) > 1:
Checking the length of the list is O(1).
Popping an Element:
nums.pop(index)
Removing the first element from the list using
pop(0)
is O(n) because all subsequent elements need to be shifted.Creating & Building
minimums
List:minimums = [] for i in nums: if minimum > i: minimums.append(i)
Initializing an empty list is O(1). The for loop iterates through the remaining
n-1
elements, making it an O(n) operation. Theappend
operation inside the loop is O(1), but considering it happens up ton-1
times, it remains O(n).Recursive Call:
if len(minimums) != 0: return findMin(minimums)
The length check is O(1), and the recursive call will process a list that is one element shorter.
Overall Time Complexity
Combining all these, each call results in an O(n) operation due to the pop(0)
and the for loop, and there are up to n
recursive calls in the worst case. This leads to the time complexity being O(n^2).
Summary:
- Best Case: O(n)
- Minimal recursion, all elements larger than the first one.
- Average Case: O(n^2)
- On average, a substantial fraction of the list is less than the initial minimum.
- Worst Case: O(n^2)
- When each element is examined in a descending sorted array.
Space Complexity Analysis
Step-by-Step Breakdown
Base Space Usage:
- Variables
index
,minimum
: O(1) - List
minimums
: O(n) in worst case, as it can store all elements except one initially.
- Variables
Recursive Stack:
- The recursion depth can go up to
n
in the worst case, resulting in O(n) additional space.
- The recursion depth can go up to
Overall Space Complexity
The primary contributors are the list minimums
and the recursive call stack.
- Space Complexity: O(n)
Summary:
- Overall Space Complexity: O(n)
Average-Case Scenario Illustration
Practical Input Scenario
Consider an unsorted array such as [3, 1, 4, 1, 5, 9, 2]
.
Expected Runtime or Memory Usage
- Average-Case Runtime: The function will experience an average of O(n^2) operations.
- Memory Usage: On average, the space requirement remains O(n) due to the dynamic size of the
minimums
list and recursion stack.
Comments on Non-Obvious Parts of Code
minimums.append(i)
Impact:- Critical as it determines new subarray for recursion, directly impacts overall complexity.
- Recursive Call
findMin(minimums)
:- Key factor in time complexity, due to potential repeated slicing and comparisons.
For further learning, consider exploring Big-O notation and algorithm analysis topics on the Enterprise DNA Platform.
Description
The document provides a thorough analysis of the time and space complexity of the findMin
function in Python, discussing the step-by-step breakdown, different scenarios, and key aspects impacting the algorithm's performance.
More AI Big-O Analyzers
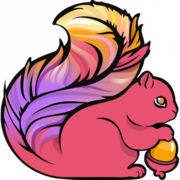
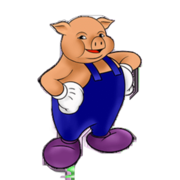
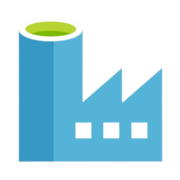
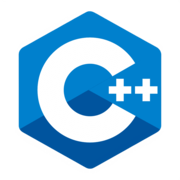
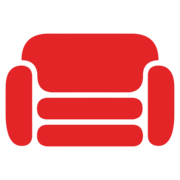
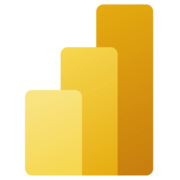
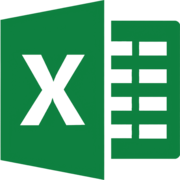
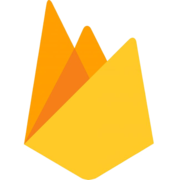
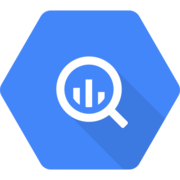
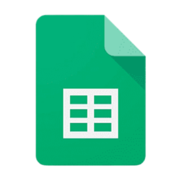
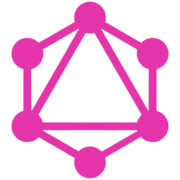
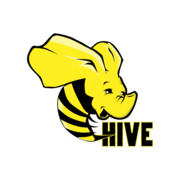
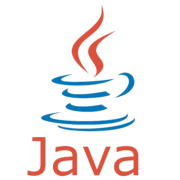
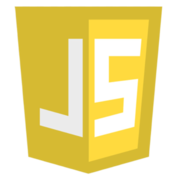
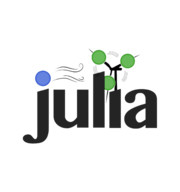
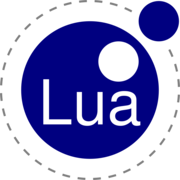
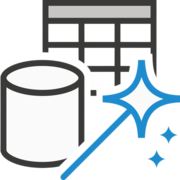
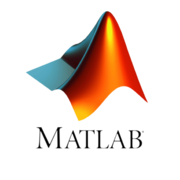
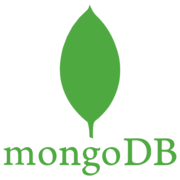
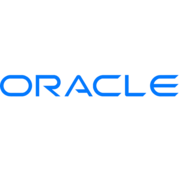
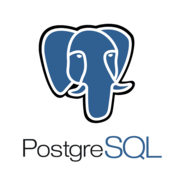
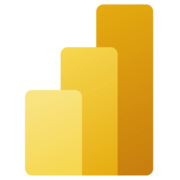
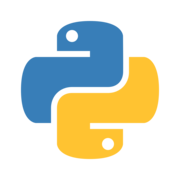
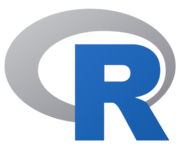
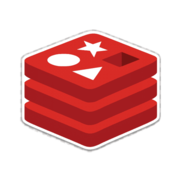
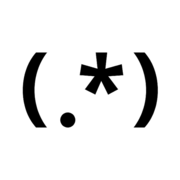
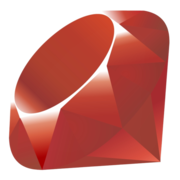
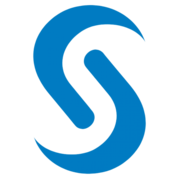
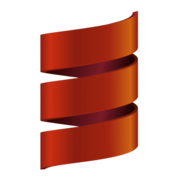
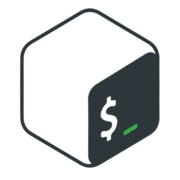
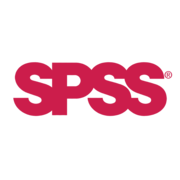
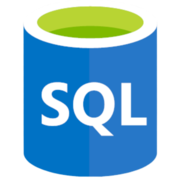
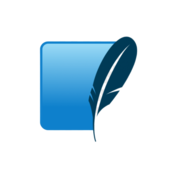
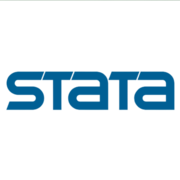
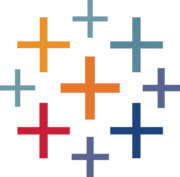
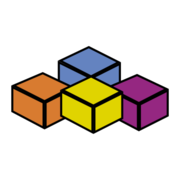