Complexity Estimator | Python
Efficient Duplicate Detection Algorithm
Analyzing a code snippet with O(n) time complexity for finding duplicates in a list using a dictionary. The space complexity is also O(n), offering optimal performance. Insights and potential optimizations are provided for enhancing code
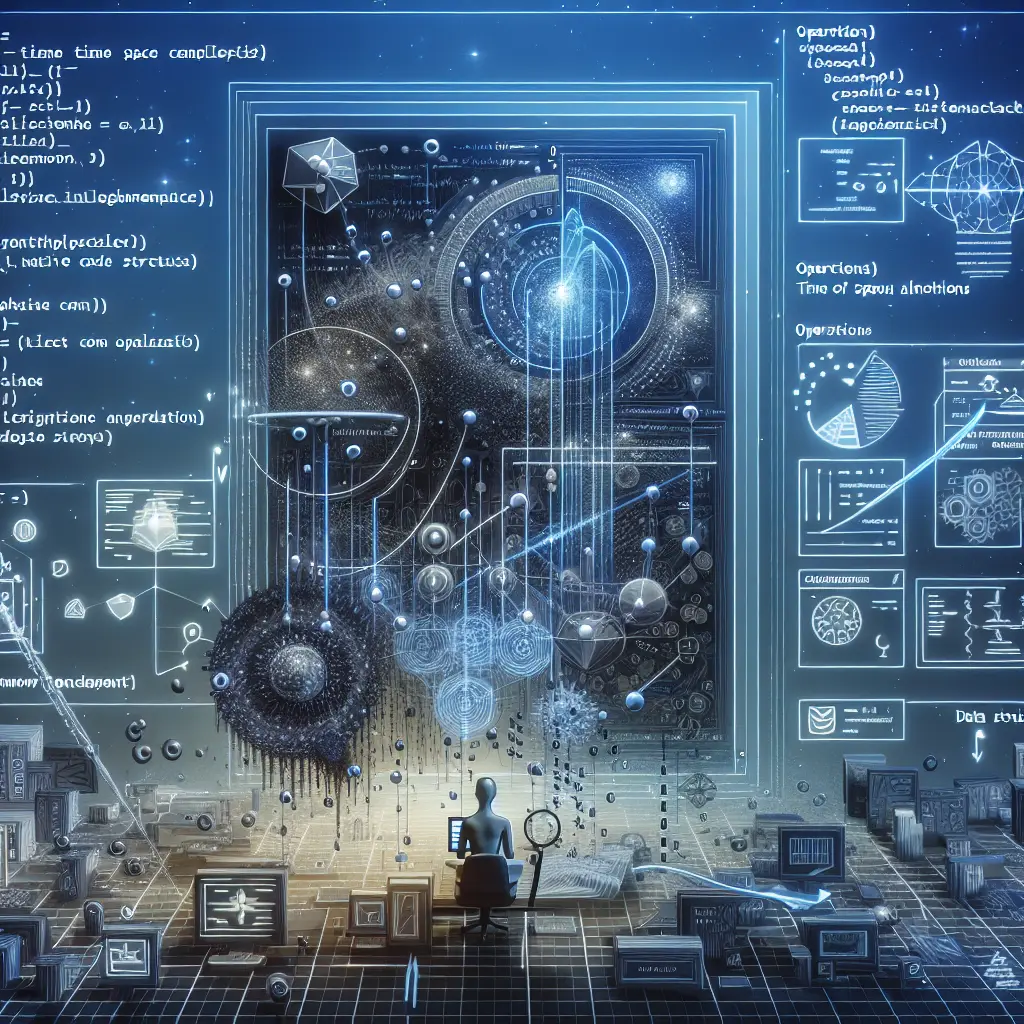
Prompt
def find_duplicates(lst):
d = {}
for i in lst:
if i in d:
d[i] += 1
else:
d[i] = 1
return [k for k, v in d.items() if v > 1]
Answer
Time Complexity:
- Best case: O(n)
- Average case: O(n)
- Worst case: O(n)
Explanation:
- The for loop iterates through each element in the input list once, resulting in a linear time complexity of O(n).
- The dictionary lookup for each element has an average time complexity of O(1) due to the use of hashing.
- Therefore, the overall time complexity of the function is O(n) in all cases.
Space Complexity:
- Space complexity: O(n)
Explanation:
- The space complexity is O(n) because the function uses a dictionary to store the count of each element in the list.
- In the worst case scenario where all elements are unique, the dictionary will store each element, resulting in O(n) space complexity.
Insights:
- The code has efficient time complexity of O(n) due to linear traversal of the input list.
- The dictionary lookup for element counts is done in constant time on average, aiding in overall performance.
- The space complexity is optimal at O(n) due to storing unique elements in the dictionary.
- There are no significant bottlenecks in the code, and it is already optimized for finding duplicates efficiently.
Optimization:
- The code is already well-optimized given the problem requirements.
- Further optimization may involve reducing memory usage by processing elements one at a time instead of storing them in a dictionary.
- Enterprise DNA's platform offers courses on algorithm optimization techniques that can help enhance coding skills further.
Description
Analyzing a code snippet with O(n) time complexity for finding duplicates in a list using a dictionary. The space complexity is also O(n), offering optimal performance. Insights and potential optimizations are provided for enhancing code efficiency. Enterprise DNA's platform provides algorithm optimization courses for skill development.
More Complexity Estimators
Apache Flink Complexity EstimatorApache Pig Complexity EstimatorAzure Data Factory Complexity EstimatorC/C++ Complexity EstimatorCouchDB Complexity EstimatorDAX Complexity EstimatorExcel Complexity EstimatorFirebase Complexity EstimatorGoogle BigQuery Complexity EstimatorGoogle Sheets Complexity EstimatorGraphQL Complexity EstimatorHive Complexity EstimatorJava Complexity EstimatorJavaScript Complexity EstimatorJulia Complexity EstimatorLua Complexity EstimatorM (Power Query) Complexity EstimatorMATLAB Complexity EstimatorMongoDB Complexity EstimatorOracle Complexity EstimatorPostgreSQL Complexity EstimatorPower BI Complexity EstimatorPython Complexity EstimatorR Complexity EstimatorRedis Complexity EstimatorRegex Complexity EstimatorRuby Complexity EstimatorSAS Complexity EstimatorScala Complexity EstimatorShell Complexity EstimatorSPSS Complexity EstimatorSQL Complexity EstimatorSQLite Complexity EstimatorStata Complexity EstimatorTableau Complexity EstimatorVBA Complexity Estimator