Prompt
Answer
Pseudo Code
Problem Statement
Given three integers a
, b
, and c
, execute the following operations:
- Set initial values:
a = 8
,b = 3
,c = 10
. - Update
c
using the formula:c = (b^b) + c
. - Further update
c
using the formula:c = (c + c) + c
. - Print the sum of
a
,b
, andc
.
Pseudo Code
Declare integer a, b, c
Set a = 8
Set b = 3
Set c = 10
# Calculate b^b and update c
c = (b ^ b) + c # In many languages, ^ denotes exponentiation
# Update c with the new value
c = (c + c) + c # This is equivalent to c = 3 * c
# Calculate and print the sum of a, b, and c
Print a + b + c
Explanation of Pseudo Code
Initialization of Variables:
- Set
a
to 8. - Set
b
to 3. - Set
c
to 10.
- Set
First Update to
c
:- Calculate
b
raised to the power ofb
(which is3^3 = 27
). - Add this result to
c
. Now,c = 27 + 10
.
- Calculate
Second Update to
c
:- For the value of
c
obtained after the first update (which is 37), perform the operation(c + c) + c
. - This results in
c = (37 + 37) + 37 = 111
.
- For the value of
Output:
- Calculate the sum of
a
,b
, andc
(i.e.,8 + 3 + 111
). - Print the result.
- Calculate the sum of
Conclusion
The pseudo code clearly outlines the steps and updates performed on the variables. This serves as a basic roadmap for implementing the solution in any programming language. For further learning and deeper understanding of pseudo code and algorithm development, consider exploring resources available on the Enterprise DNA Platform.
Description
This pseudo code demonstrates a series of operations on three integers, a
, b
, and c
. It initializes the values, updates c
with calculations based on b
, and finally prints the total sum of the three integers.
More Pseudo Code Generators
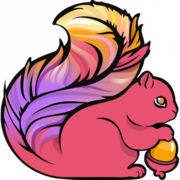
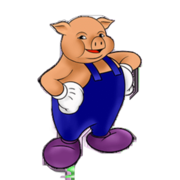
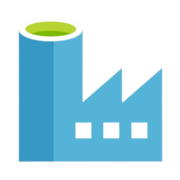
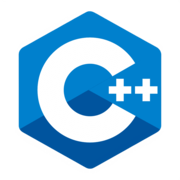
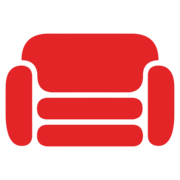
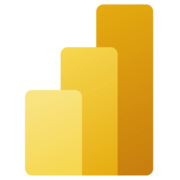
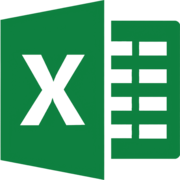
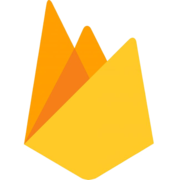
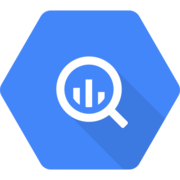
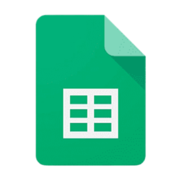
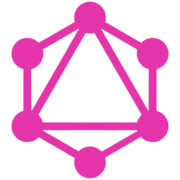
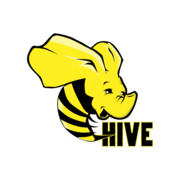
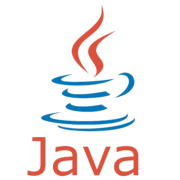
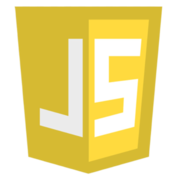
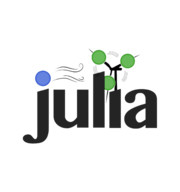
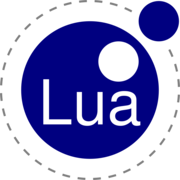
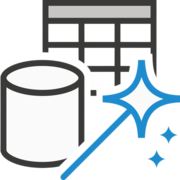
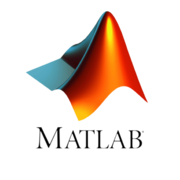
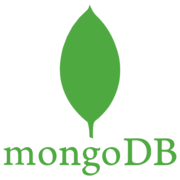
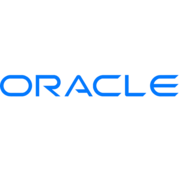
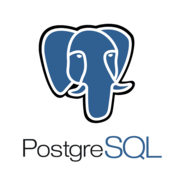
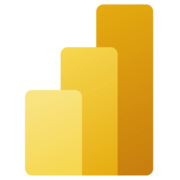
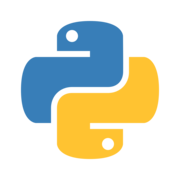
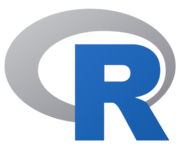
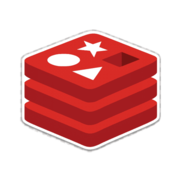
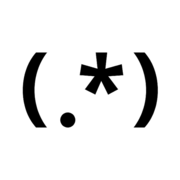
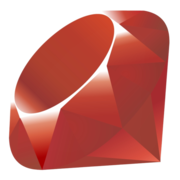
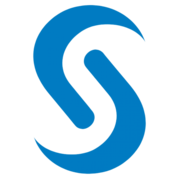
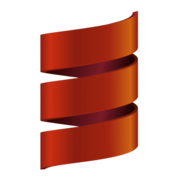
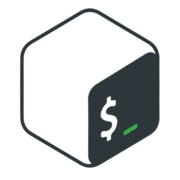
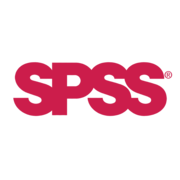
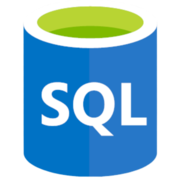
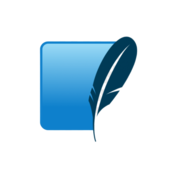
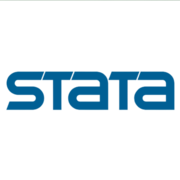
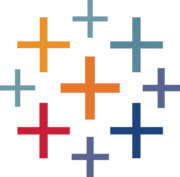
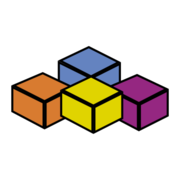