Prompt
Answer
Pseudocode: Year-over-Year (YoY) and Month-over-Month (MoM) Membership Enrollments
Objective
Calculate Year-over-Year (YoY) and Month-over-Month (MoM) growth rates for membership enrollments.
Key Definitions
- YoY Growth Rate: Percentage change of enrollments compared to the same month in the previous year.
- MoM Growth Rate: Percentage change of enrollments compared to the previous month.
Inputs
enrollment_data
: List of dictionaries containing monthly enrollment counts.- Each dictionary has keys:
year
,month
,enrollments
.
- Each dictionary has keys:
Outputs
calculated_data
: List of dictionaries containing the year, month, enrollments, YoY growth rate, and MoM growth rate.
Process
- Initialize an empty list for
calculated_data
. - Iterate through sorted
enrollment_data
. - For each record, calculate YoY and MoM growth rates, if applicable.
- Append results to
calculated_data
.
Pseudocode
FUNCTION calculate_growth_rates(enrollment_data):
# Sort enrollment_data list by year, then by month
sorted_data = SORT(enrollment_data BY year, month)
# Initialize an empty list for the results
calculated_data = []
# Loop through sorted enrollment_data
FOR i FROM 0 TO LENGTH(sorted_data) - 1:
current_record = sorted_data[i]
current_year = current_record["year"]
current_month = current_record["month"]
current_enrollments = current_record["enrollments"]
# Initialize YoY and MoM growth rates
YoY_growth_rate = NULL
MoM_growth_rate = NULL
# Calculate YoY growth rate if possible
previous_year_record = FIND_RECORD(sorted_data, current_year - 1, current_month)
IF previous_year_record EXISTS:
previous_year_enrollments = previous_year_record["enrollments"]
YoY_growth_rate = (current_enrollments - previous_year_enrollments) / previous_year_enrollments * 100
# Calculate MoM growth rate if possible
previous_month_record = FIND_RECORD(sorted_data, current_year, current_month - 1)
IF NOT previous_month_record EXISTS AND current_month == 1:
previous_month_record = FIND_RECORD(sorted_data, current_year - 1, 12)
IF previous_month_record EXISTS:
previous_month_enrollments = previous_month_record["enrollments"]
MoM_growth_rate = (current_enrollments - previous_month_enrollments) / previous_month_enrollments * 100
# Append calculated data to the results list
calculated_record = {
"year": current_year,
"month": current_month,
"enrollments": current_enrollments,
"YoY_growth_rate": YoY_growth_rate,
"MoM_growth_rate": MoM_growth_rate
}
APPEND(calculated_data, calculated_record)
RETURN calculated_data
# Helper Function to find a record by year and month
FUNCTION FIND_RECORD(data, year, month):
FOR record IN data:
IF record["year"] == year AND record["month"] == month:
RETURN record
RETURN NULL
Notes
- This pseudocode assumes that
enrollment_data
is a list of dictionaries and uses helper functions likeSORT
andFIND_RECORD
. - For leap years and other calendar considerations, adjustments may be necessary.
- To learn more about advanced analytics and pseudocode implementation, consider courses on the Enterprise DNA platform.
Description
This pseudocode outlines a method to calculate Year-over-Year (YoY) and Month-over-Month (MoM) growth rates for membership enrollments based on provided monthly enrollment data. It includes data sorting, calculations, and result compilation.
More Pseudo Code Generators
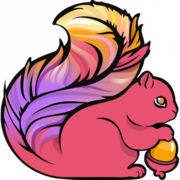
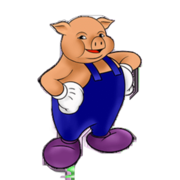
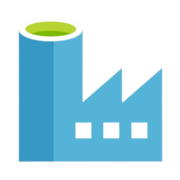
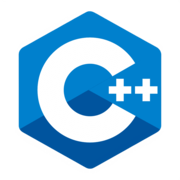
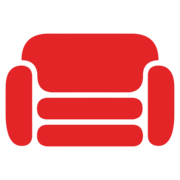
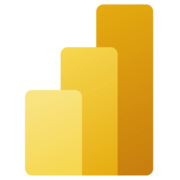
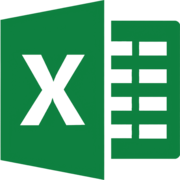
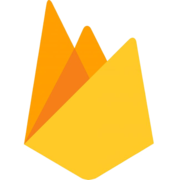
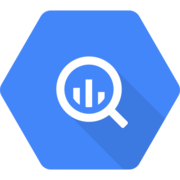
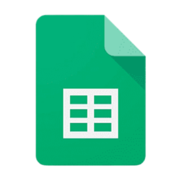
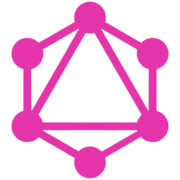
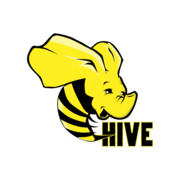
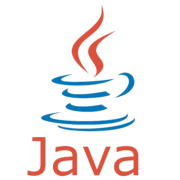
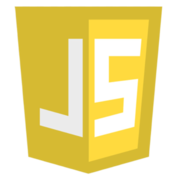
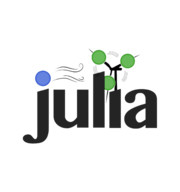
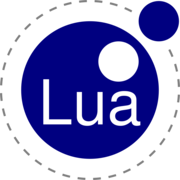
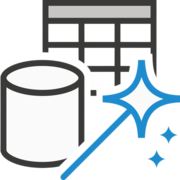
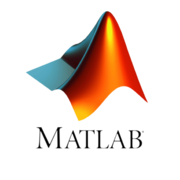
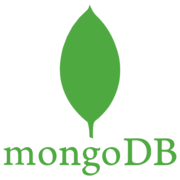
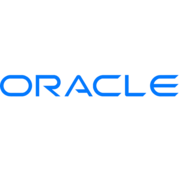
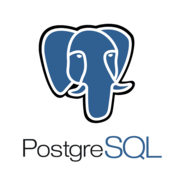
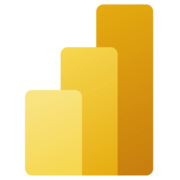
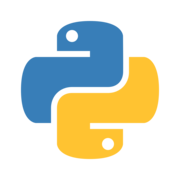
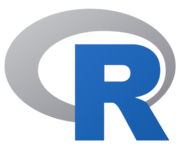
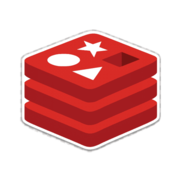
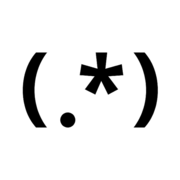
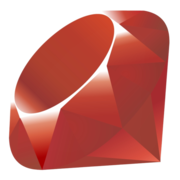
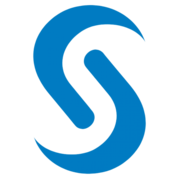
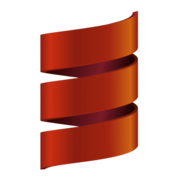
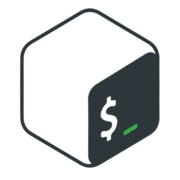
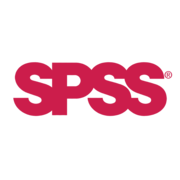
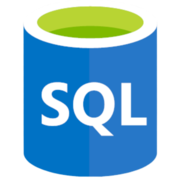
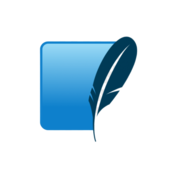
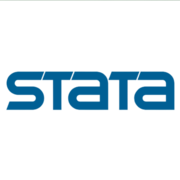
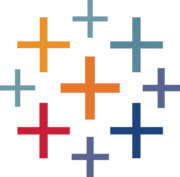
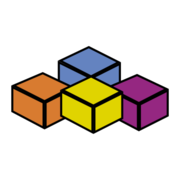