Code Issues Solver
Overcoming XGBoost Challenges for Regression on Large Datasets
This guide details key challenges faced while implementing XGBoost for regression on large datasets, including memory management, overfitting, and feature engineering. Solutions and Python code snippets are provided for effective model

This Query related with thread "Mastering XGBoost: A Comprehensive Exploration"
Prompt
Answer
Common Challenges When Implementing XGBoost for Regression on Large Datasets
1. Memory Management
- Issue: Large datasets require substantial memory, leading to potential memory overflow.
- Solution: Use the
DMatrix
functionality in XGBoost which optimizes memory usage. Additionally, employ batch processing to handle datasets incrementally.
2. Overfitting
- Issue: XGBoost, like other powerful models, can overfit on large datasets.
- Solution: Use regularization parameters such as
lambda
(L2 regularization) andalpha
(L1 regularization). Also, make use of cross-validation for hyperparameter tuning.
3. Feature Engineering
- Issue: Irrelevant or redundant features can impact model performance.
- Solution: Perform thorough feature selection and engineering. Utilize techniques like mutual information gain and correlation analysis to filter features.
4. Parameter Tuning
- Issue: XGBoost has numerous hyperparameters, which can be overwhelming to tune manually.
- Solution: Utilize automated hyperparameter optimization tools like Grid Search, Random Search, or Bayesian Optimization (
optuna
package).
5. Model Interpretability
- Issue: XGBoost models can be complex and difficult to interpret.
- Solution: Use SHAP (SHapley Additive exPlanations) values to explain model predictions and gain insights.
6. Imbalanced Data
- Issue: Imbalanced datasets can bias the model towards majority values.
- Solution: Apply techniques such as SMOTE (Synthetic Minority Over-sampling Technique) or use specialized parameters in XGBoost like
scale_pos_weight
.
Solution Implementation in Python
1. Data Import and Preprocessing
import xgboost as xgb
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
from sklearn.model_selection import GridSearchCV
import pandas as pd
import numpy as np
# Load dataset
boston = load_boston()
X = pd.DataFrame(boston.data, columns=boston.feature_names)
y = pd.Series(boston.target)
# Train-Test Split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
2. Memory Optimization Using DMatrix
# Create DMatrix for efficient memory usage
dtrain = xgb.DMatrix(X_train, label=y_train)
dtest = xgb.DMatrix(X_test, label=y_test)
3. Parameter Tuning via Grid Search
# Parameter grid
param_grid = {
'max_depth': [3, 4, 5],
'learning_rate': [0.01, 0.1, 0.2],
'n_estimators': [100, 200, 300],
'reg_lambda': [1, 2, 3],
'reg_alpha': [0.1, 0.2, 0.3]
}
# Grid Search for best parameters
xgb_reg = xgb.XGBRegressor()
grid_search = GridSearchCV(estimator=xgb_reg, param_grid=param_grid, scoring='neg_mean_squared_error', cv=3, verbose=1)
grid_search.fit(X_train, y_train)
# Best parameters
best_params = grid_search.best_params_
print(best_params)
4. Model Training and Evaluation
# Train model with best parameters
model = xgb.XGBRegressor(**best_params)
model.fit(X_train, y_train)
# Predictions and Evaluation
predictions = model.predict(X_test)
rmse = np.sqrt(mean_squared_error(y_test, predictions))
print(f"RMSE: {rmse}")
5. Model Interpretability
import shap
# Initialize and visualize SHAP
explainer = shap.Explainer(model)
shap_values = explainer(X_test)
# Plot summary of SHAP values
shap.summary_plot(shap_values, X_test)
Conclusion
Implementing XGBoost for regression on large datasets entails overcoming several challenges such as memory management, overfitting, feature engineering, parameter tuning, and model interpretability. Proper handling and optimization of these factors ensure effective model performance. Further knowledge can be gained through Enterprise DNA's advanced courses on machine learning and data science techniques.
Description
This guide details key challenges faced while implementing XGBoost for regression on large datasets, including memory management, overfitting, and feature engineering. Solutions and Python code snippets are provided for effective model optimization and interpretability.
More Code Issues Solvers
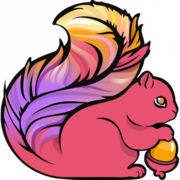
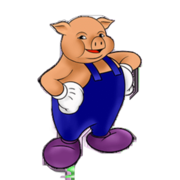
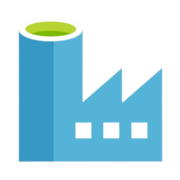
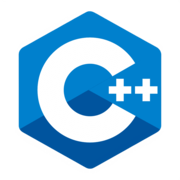
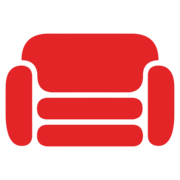
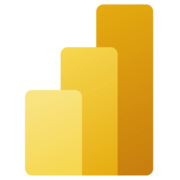
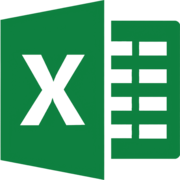
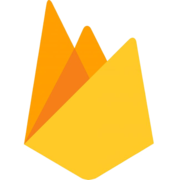
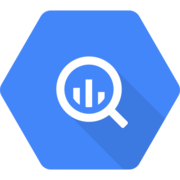
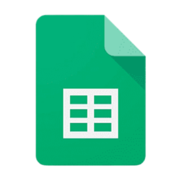
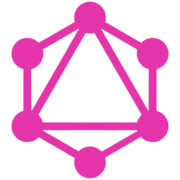
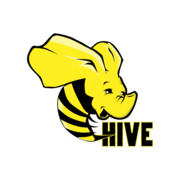
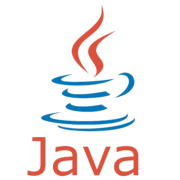
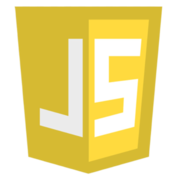
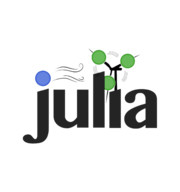
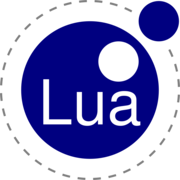
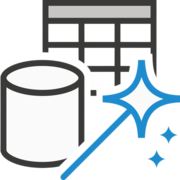
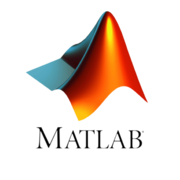
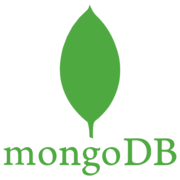
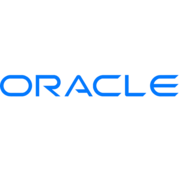
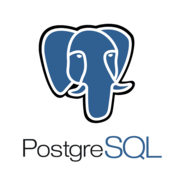
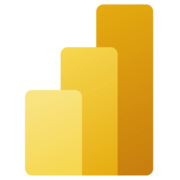
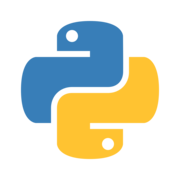
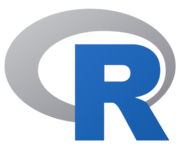
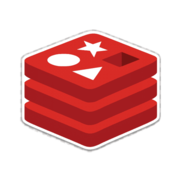
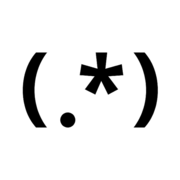
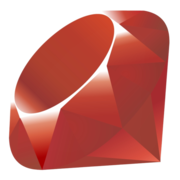
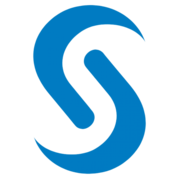
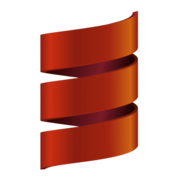
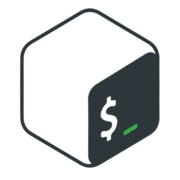
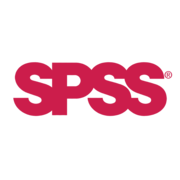
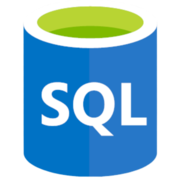
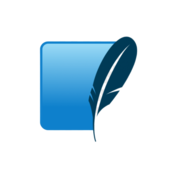
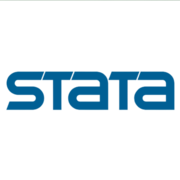
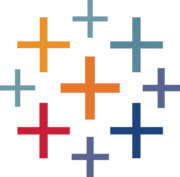
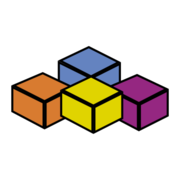