Prompt
Answer
digraph G {
nodesep=1.0;
node[shape=rectangle, fontname="Arial"];
main[label="int main()"];
declare_vars[label="int arr[10], n, i, j, temp"];
print_ask_size[label="printf(\"enter array size\")"];
scan_size[label="scanf(\"%d\", &n)"];
print_ask_elements[label="printf(\"enter the array elements\")"];
scan_elements[label="for(i=1;i<=n;i++)\n{\nscanf(\"%d\", &arr[i]);\n}" shape=box];
print_elements[label="for(i=1;i<=n;i++)\n{\nprintf(\"%d\n\", arr[i]);\n}" shape=box];
bubble_sort[label="for(i=1;i<=n;i++)\n{\nfor(j=1;j<=n-i;j++)\n{\nif(arr[j]>arr[j+1])\n{\ntemp=arr[j];\narr[j]=arr[j+1];\narr[j+1]=temp;\n}\n}\n}" shape=box];
print_sorted[label="for(i=1;i<=n;i++)\n{\nprintf(\"%d\", arr[i]);\n}" shape=box];
return_stmt[label="return 0"];
main -> declare_vars;
main -> print_ask_size;
main -> scan_size;
main -> print_ask_elements;
main -> scan_elements;
main -> print_elements;
main -> bubble_sort;
main -> print_sorted;
main -> return_stmt;
}
Code Structure Overview
Main Function:
int main()
- Entry point of the program.
Variable Declarations:
int arr[10], n, i, j, temp
- Declares an array of integers and several other integer variables used throughout the code.
User Input and Output:
printf("enter array size")
- Prompts user to enter the size of the array.
scanf("%d", &n)
- Reads the input size from the user.
printf("enter the array elements")
- Prompts user to enter the elements of the array.
for(i=1;i<=n;i++)\n{\nscanf("%d", &arr[i]);\n}
- Reads each element into the array.
for(i=1;i<=n;i++)\n{\nprintf(\"%d\n\", arr[i]);\n}
- Prints each element of the array.
Bubble Sort Algorithm:
for(i=1;i<=n;i++)\n{\nfor(j=1;j<=n-i;j++)\n{\nif(arr[j]>arr[j+1])\n{\ntemp=arr[j];\narr[j]=arr[j+1];\narr[j+1]=temp;\n}\n}\n}
- Performs the bubble sort to sort the array in ascending order.
Output Sorted Array:
for(i=1;i<=n;i++)\n{\nprintf(\"%d\", arr[i]);\n}
- Prints each sorted element of the array.
Return Statement:
return 0
- Signals successful termination of the program.
For more in-depth analysis and mentorship on code structure and optimization, consider exploring courses on the Enterprise DNA Platform.
Description
This code diagram illustrates a C program that implements a bubble sort algorithm. It covers array declaration, user input for size and elements, sorting the array, and printing both the original and sorted arrays.
More Code Visualizers
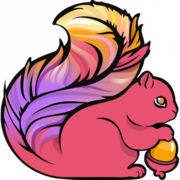
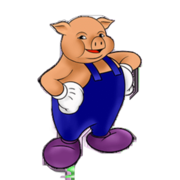
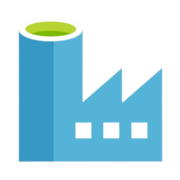
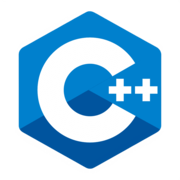
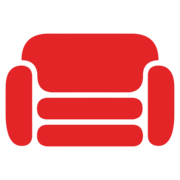
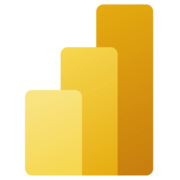
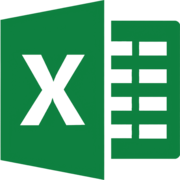
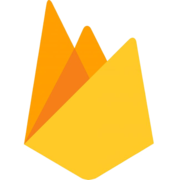
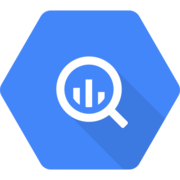
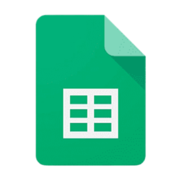
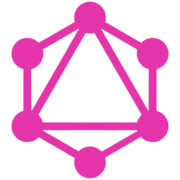
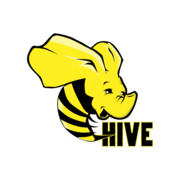
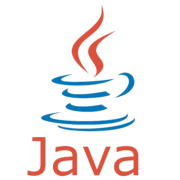
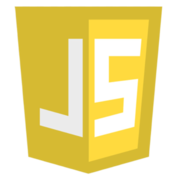
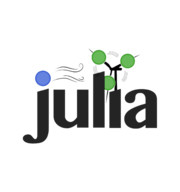
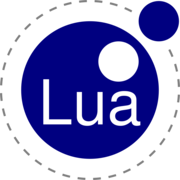
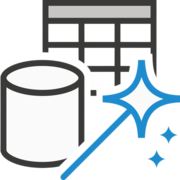
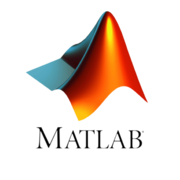
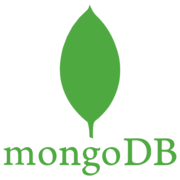
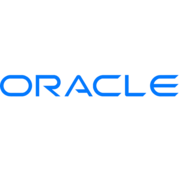
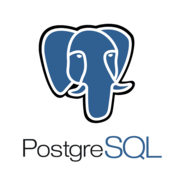
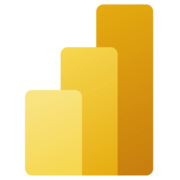
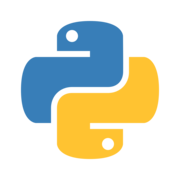
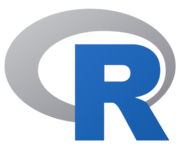
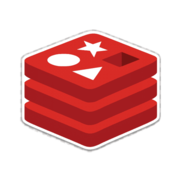
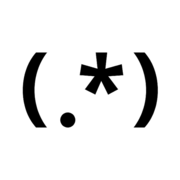
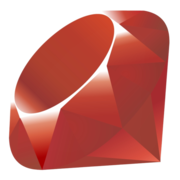
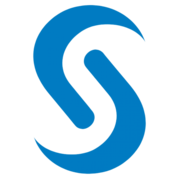
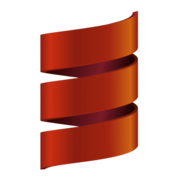
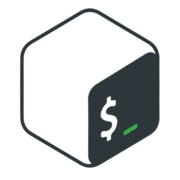
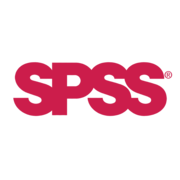
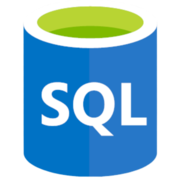
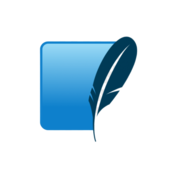
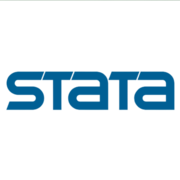
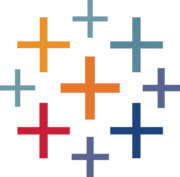
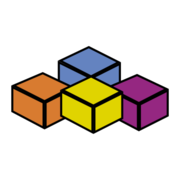