Prompt
Answer
Code Logic Visualization
Text Explanation Analysis
The given code is a simple C program that processes a string of numbers separated by spaces, converts them to floating-point values, computes their sum, and then calculates the average. The average is printed with three decimal precision.
Key Components
- Libraries:
stdio.h
,string.h
,stdlib.h
- Variables:
str
,ptr_start
,ptr_end
,sum
,amount
- Functions:
main
,scanf
,strchr
,atof
,printf
Main Logic Flow
- Initialize a character array
str
. - Scan input into
str
. - Define pointers to locate spaces and the end of
str
. - Iterate through the string to process each number.
- Convert each number from a substring to
double
and add tosum
. - Increment the count of numbers processed.
- Calculate the average by dividing the total sum by the count of numbers.
- Print the average to three decimal places.
Visual Representation
Flowchart
Below is a flowchart describing the logic and flow of the provided C code:
+--------------------------------+
| Start |
+--------------------------------+
|
v
+-----------------------------+
| Initialize `str` with 50 |
| characters set to 0 |
+-----------------------------+
|
v
+-----------------------------+
| Scan input into `str` |
+-----------------------------+
|
v
+-----------------------------+
| Find first space in `str` |
| using `strchr` (ptr_start) |
+-----------------------------+
|
v
+-----------------------------+
| Find end of `str` using |
| `strchr` (ptr_end) |
+-----------------------------+
|
v
+-----------------------------+
| Initialize `sum` to 0.0 |
| Initialize `amount` to 0 |
+-----------------------------+
|
v
+-----------------------------+
| For ptr_start <= ptr_end |
| - Convert to double |
| using `atof` |
| - Add value to `sum` |
| - Increment `amount` |
| - Move ptr_start by 3 |
+-----------------------------+
|
v
+-----------------------------+
| Calculate average: |
| sum = sum / amount |
+-----------------------------+
|
v
+-----------------------------+
| Print `sum` to 3 decimals |
+-----------------------------+
|
v
+-----------------------------+
| Return 0 (End) |
+-----------------------------+
Detailed Pseudocode
Here is the detailed pseudocode representation of the C code:
variables:
char str[50] = {0}
char *ptr_start
char *ptr_end
double sum = 0.0
int amount = 0
main:
// Read input into str
scanf("%s", &str)
// Find first space character
ptr_start = strchr(str, ' ')
// Find end of string
ptr_end = strchr(str, '\0')
// Loop through string and process each number
while ptr_start <= ptr_end:
// Convert current segment to float and add to sum
sum += atof(ptr_start)
// Increment count of numbers processed
amount += 1
// Move ptr_start 3 positions ahead
ptr_start += 3
// Calculate average
sum = sum / amount
// Print the result with 3 decimal places
printf("%.3f", sum)
// End of program
return 0
Conclusion
This document has transformed the provided C code into both a flowchart and detailed pseudocode. The goal is to present the logic and structure clearly, making it accessible to individuals with varied levels of technical expertise. The flowchart and pseudocode highlight the critical components and flow of the program, ensuring a comprehensive understanding of the code's operations.
Description
Detailed examination of a C program converting number strings to floats, summing, and finding average. Visual and pseudocode representations included for clear understanding.
More Logic Visualizers
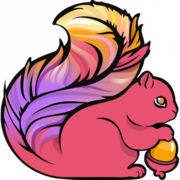
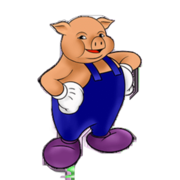
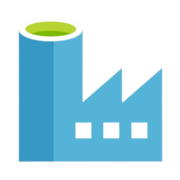
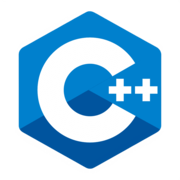
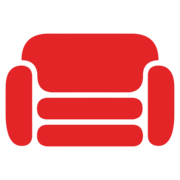
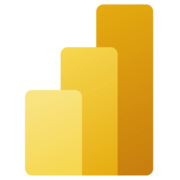
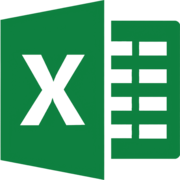
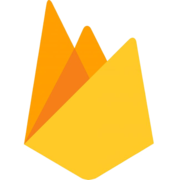
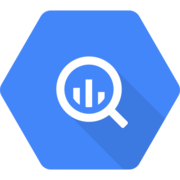
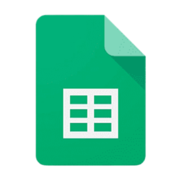
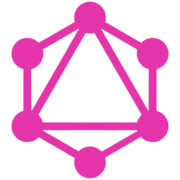
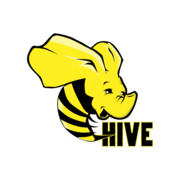
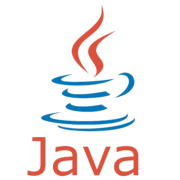
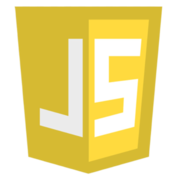
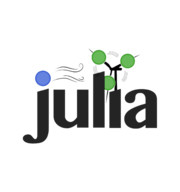
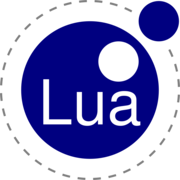
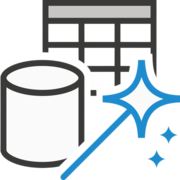
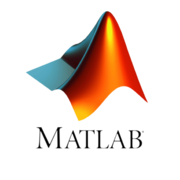
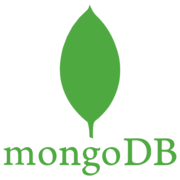
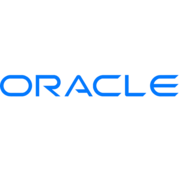
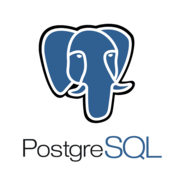
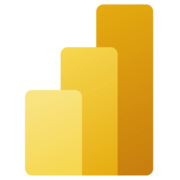
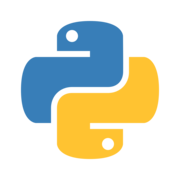
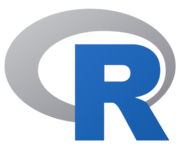
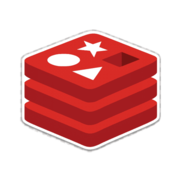
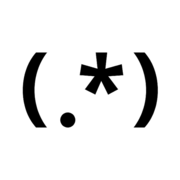
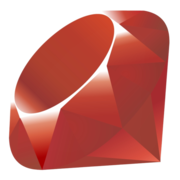
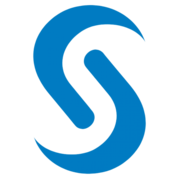
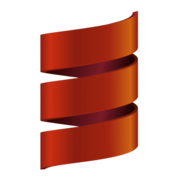
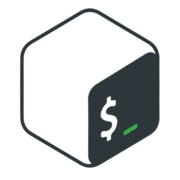
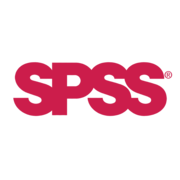
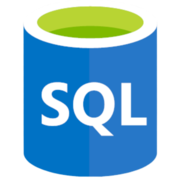
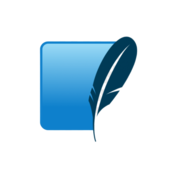
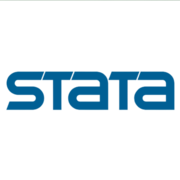
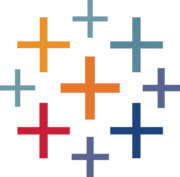
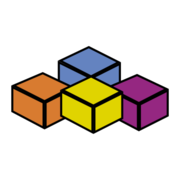