Prompt
Answer
The code snippet imports the plotly.express library as px. The plotly.express library is a high-level API for creating interactive visualizations. It provides an easy way to create a variety of charts and plots.
The code then defines a sample dataset called 'data', which contains three columns: 'id', 'parent', and 'value'. Each row represents a node in a hierarchical structure.
Next, the code uses the px.sunburst() function from the plotly.express library to create a sunburst chart. A sunburst chart is a circular visual representation of a hierarchical structure, similar to a pie chart.
The px.sunburst() function takes the 'data' dataset as input, and specifies the 'names', 'parents', and 'values' columns from the dataset. The 'names' column contains the unique identifiers for each node, the 'parents' column specifies the parent node for each node, and the 'values' column represents the size or value associated with each node.
The code then uses the fig.update_layout() method to set the title of the chart to "Sunburst Chart". This method allows you to customize various aspects of the chart layout, such as the title, axis labels, and color scheme.
Finally, the code uses the fig.show() method to display the chart. This method shows the chart in the output console or in a separate window, depending on the programming environment or IDE being used.
In summary, this code snippet imports the plotly.express library, creates a sample dataset, generates a sunburst chart using the dataset, sets the chart title, and displays the chart.
Description
This project utilizes the plotly.express library, a high-level API for creating interactive visualizations, to create a sunburst chart. A sunburst chart is a circular visualization that represents a hierarchical structure, resembling a pie chart.
The code begins by importing the plotly.express library as "px". This library simplifies the process of creating a variety of charts and plots.
Next, a sample dataset called 'data' is defined. This dataset contains three columns: 'id', 'parent', and 'value', where each row represents a node in the hierarchical structure.
Using the px.sunburst() function, the code generates a sunburst chart. This function takes the 'data' dataset as input and specifies the relevant columns: 'names', which contains the unique identifiers for each node; 'parents', which indicates the parent node for each node; and 'values', representing the size or value associated with each node.
To customize the chart's layout, the code uses the fig.update_layout() method. This method allows adjustments to various aspects, such as setting the chart title to "Sunburst Chart".
Finally, the fig.show() method is used to display the chart, either in the output console or a separate window depending on the programming environment or IDE in use.
In summary, this code showcases how to create a sunburst chart with plotly.express, including importing the library, defining a dataset, generating the chart, and making customization to the chart's layout before displaying it.
More Code Explainers
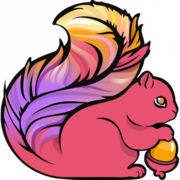
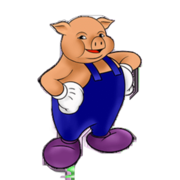
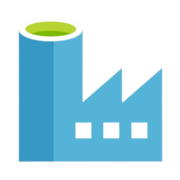
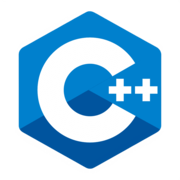
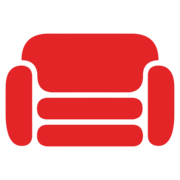
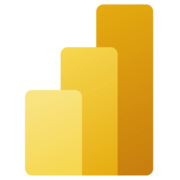
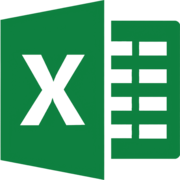
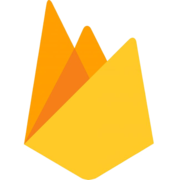
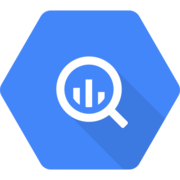
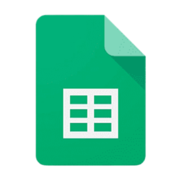
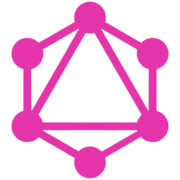
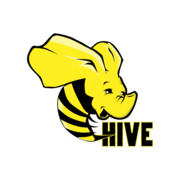
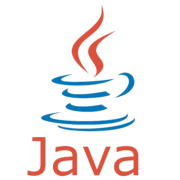
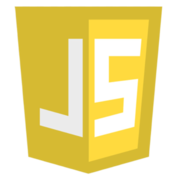
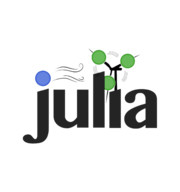
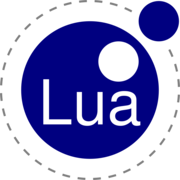
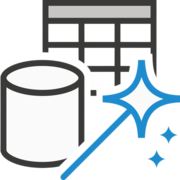
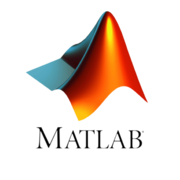
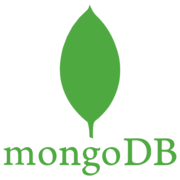
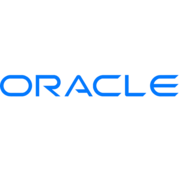
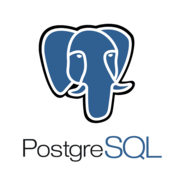
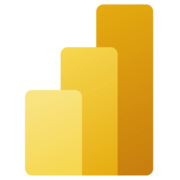
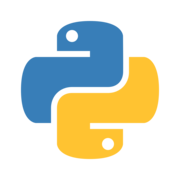
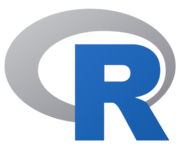
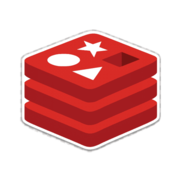
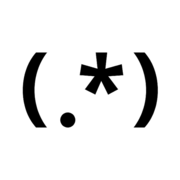
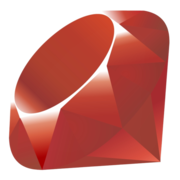
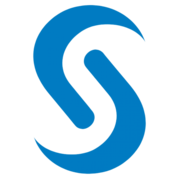
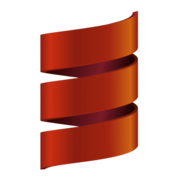
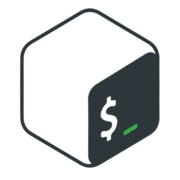
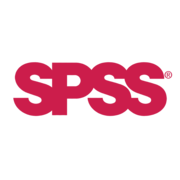
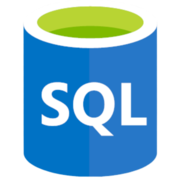
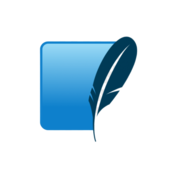
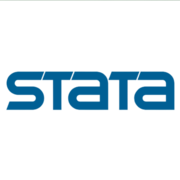
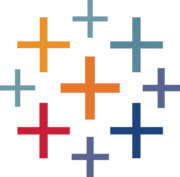
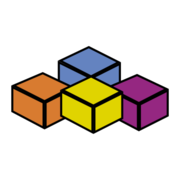