Prompt
Answer
local result = ''
if model['PressureRange'] and not ScriptHelpers:IsNullOrEmpty(model['PressureRange']) then
result = model['PressureRange']['Name']
end
if model['TemperatureRange'] and not ScriptHelpers:IsNullOrEmpty(model['TemperatureRange']) then
result = model['TemperatureRange']['Name']
end
if result == '' then
if model['Smax'] ~= model['Smin'] and (model['Smax'] ~= 0 and model['Smin'] ~= 0) then
result = model['Smin'] .. '...' .. model['Smax']
end
end
if result ~= '' then
if not ScriptHelpers:IsNullOrEmpty(model['VUnits']) and model['VUnits']['Name'] ~= '-' then
result = result .. ' ' .. model['VUnits']['Name']
end
end
if ScriptHelpers:IsNullOrEmpty(model['VUnits']) and not ScriptHelpers:IsNullOrEmpty(model['SUnits']) and model['SUnits']['Name'] ~= '-' then
result = result .. ' ' .. model['SUnits']['Name']
end
return result
Explanation:
Initialization:
local result = ''
Pressure Range Check:
- Checks if
model['PressureRange']
is not null/empty and assignsresult
.
- Checks if
Temperature Range Check:
- Checks if
model['TemperatureRange']
is not null/empty and assignsresult
.
- Checks if
Range Condition:
- Checks if
result
is still empty, and ifmodel['Smax']
andmodel['Smin']
are not equal and both non-zero, assigns a range toresult
.
- Checks if
Result Suffix for VUnits:
- Appends
VUnits
name toresult
if not null/empty and not'-'
.
- Appends
Result Suffix for SUnits:
- Appends
SUnits
name toresult
ifVUnits
is null/empty andSUnits
is not null/empty and not'-'
.
- Appends
Note:
- Lua uses the
~=
operator for inequality, unlike!=
in some other languages. - Lua uses the
:
syntax for calling methods of objects (e.g.,ScriptHelpers:IsNullOrEmpty
). - The code is written in a compact and readable format consistent with Lua best practices.
Description
This Lua script dynamically generates a result string based on pressure, temperature, and size ranges from a given model, appending appropriate units if provided. It ensures meaningful output by checking for null values and formatting conditions.
More Language Translators
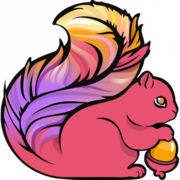
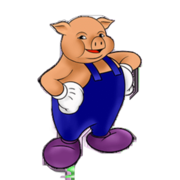
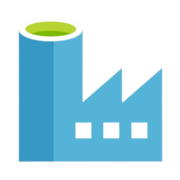
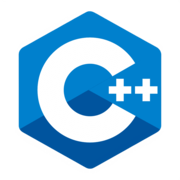
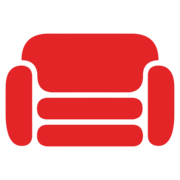
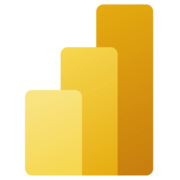
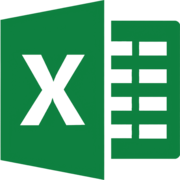
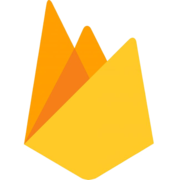
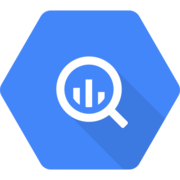
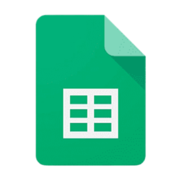
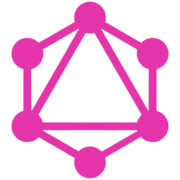
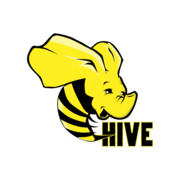
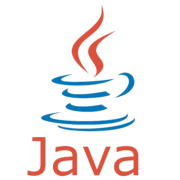
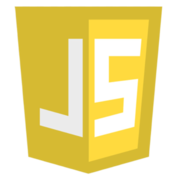
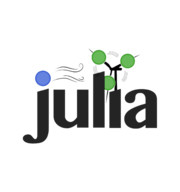
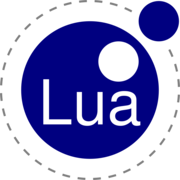
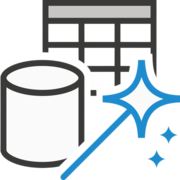
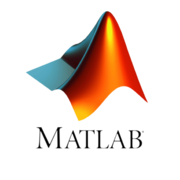
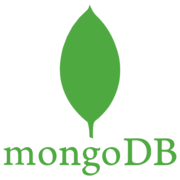
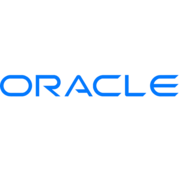
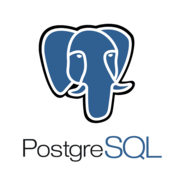
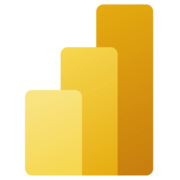
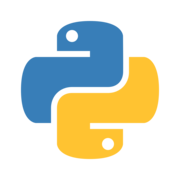
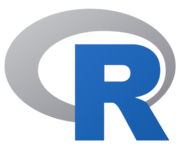
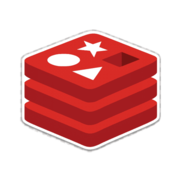
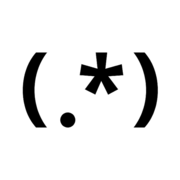
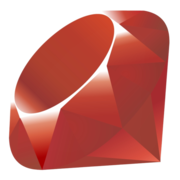
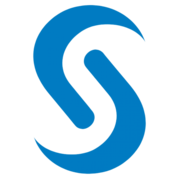
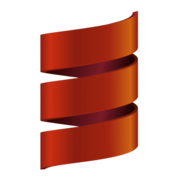
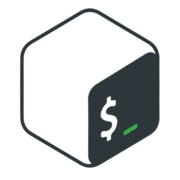
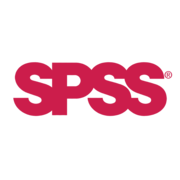
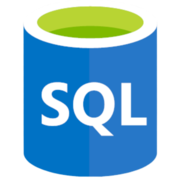
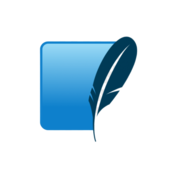
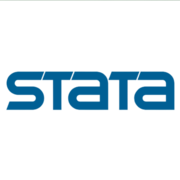
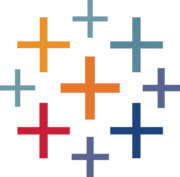
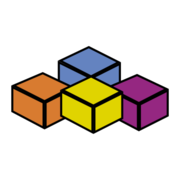