Prompt
Answer
Visual Representation of the Code using DOT Notation
Overview
The given Java method Solution
generates all permutations of a string str
by using a recursive approach. Below is the DOT notation to showcase the relationships between various calls in this method.
DOT Notation
digraph G {
node [shape=box];
main_function [label="Solution(str, ans)"];
if_condition [label="if(str.length() == 0)"];
print_statement [label='System.out.print(ans + " ")'];
for_loop [label="for(int i = 0; i < str.length(); i++)"];
recursive_call [label="Solution(newstr, ans + curr)"];
char_extraction [label="char curr = str.charAt(i)"];
substring_creation [label="String newstr = str.substring(0, i) + str.substring(i + 1, str.length())"];
main_function -> if_condition;
if_condition -> print_statement [label="True"];
if_condition -> for_loop [label="False"];
for_loop -> char_extraction;
char_extraction -> substring_creation;
substring_creation -> recursive_call;
recursive_call -> main_function [label="Recursive Call"];
}
Explanation
- main_function: Represents the entry point of the method, where
Solution
is invoked. - if_condition: Checks if the length of the string
str
is zero.- If true, it executes the
print_statement
to print theans
. - If false, it proceeds to the
for_loop
.
- If true, it executes the
- for_loop: Iterates over each character of the string
str
.- Extracts the current character (
char_extraction
) fromstr
. - Creates a new substring
newstr
excluding the current character (substring_creation
). - Recursively calls the
Solution
method with the new substring and the updated answerans + curr
(recursive_call
).
- Extracts the current character (
Further Learning
For more detailed learning on recursion and algorithmic complexity, consider exploring resources and courses available on the Enterprise DNA Platform.
Description
This document details a Java method that generates all permutations of a string through recursion, illustrated with DOT notation to visualize the method's structure and flow, enhancing understanding of recursion and algorithmic processes.
More Code Visualizers
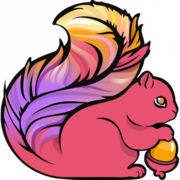
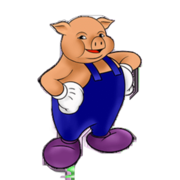
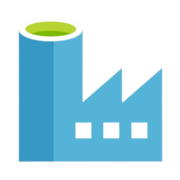
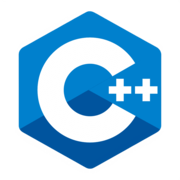
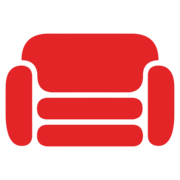
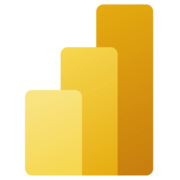
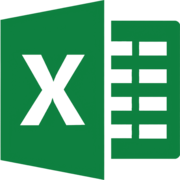
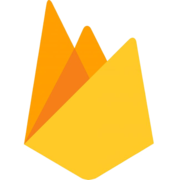
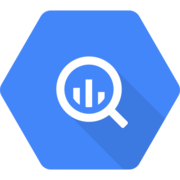
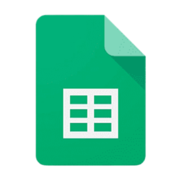
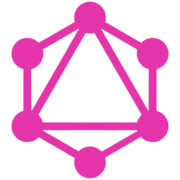
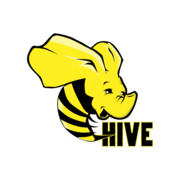
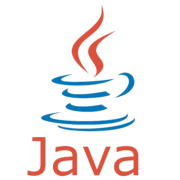
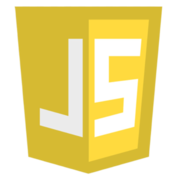
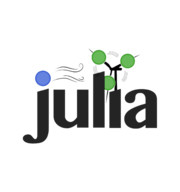
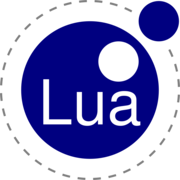
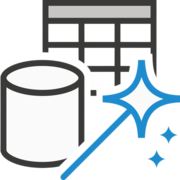
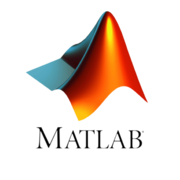
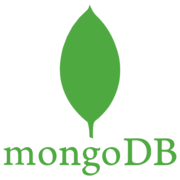
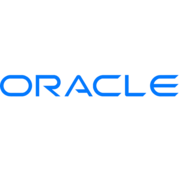
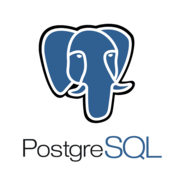
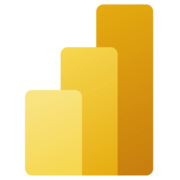
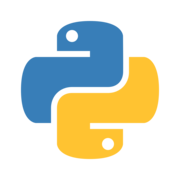
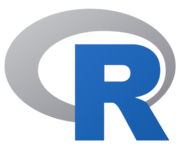
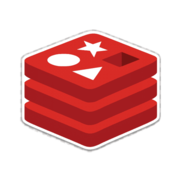
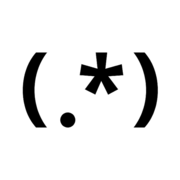
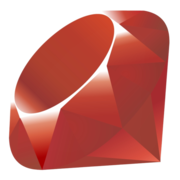
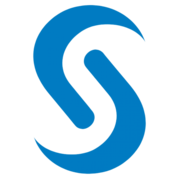
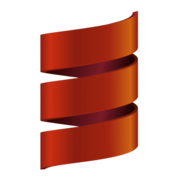
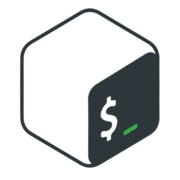
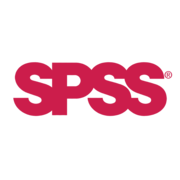
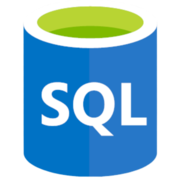
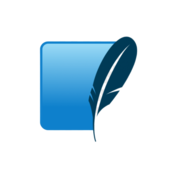
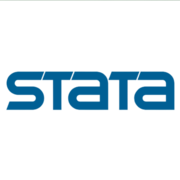
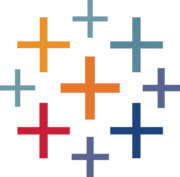
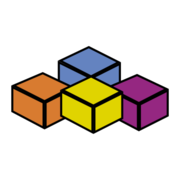