Language Translator | Python
Python Recursive Factorial Calculation
This code calculates the factorial of a number using a recursive function in Python. It checks if the input is 0 and returns 1, otherwise it multiplies the number with the factorial of (number - 1) through recursive calls. The translated code

Prompt
Answer
Translated Python code:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
number = 5
result = factorial(number)
print(f"The factorial of {number} is {result}")
The provided code snippet is a recursive function that calculates the factorial of a number. It first checks if the input n
is equal to 0. If it is, the function returns 1, as the factorial of 0 is defined as 1. Otherwise, it calculates the factorial by multiplying n
with the factorial of n - 1
, obtained through a recursive call to the same function. The translated code preserves this logic and behavior in Python. The factorial function and the calculation are implemented in the same way as the original code, and the result is printed using an f-string.
Description
This code calculates the factorial of a number using a recursive function in Python. It checks if the input is 0 and returns 1, otherwise it multiplies the number with the factorial of (number - 1) through recursive calls. The translated code preserves the logic and behavior of the original code.
More Language Translators
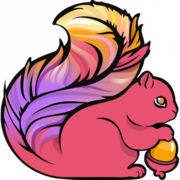
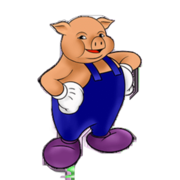
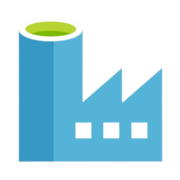
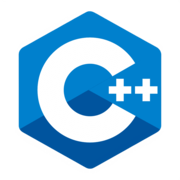
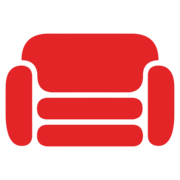
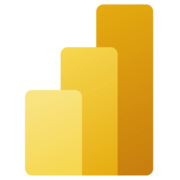
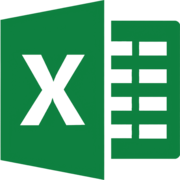
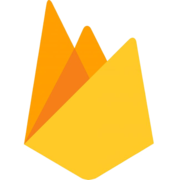
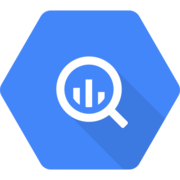
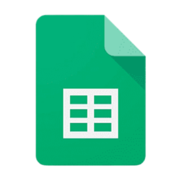
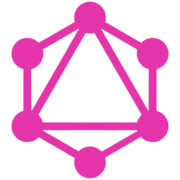
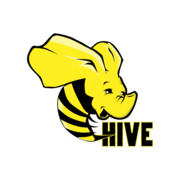
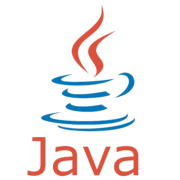
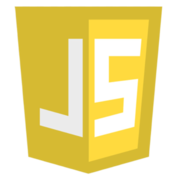
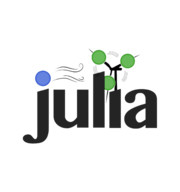
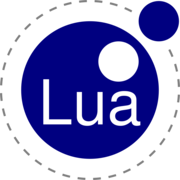
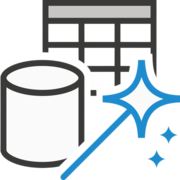
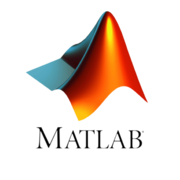
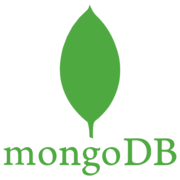
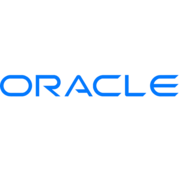
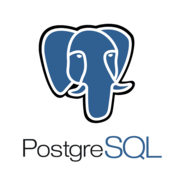
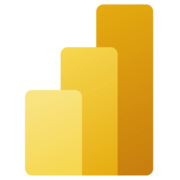
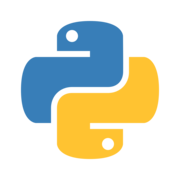
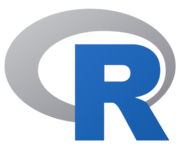
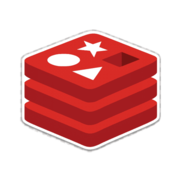
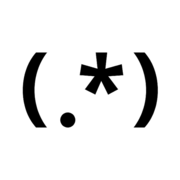
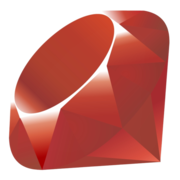
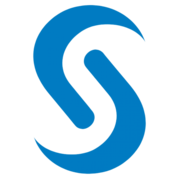
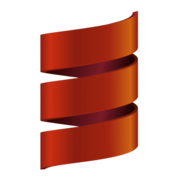
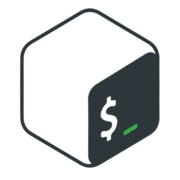
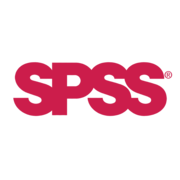
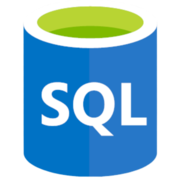
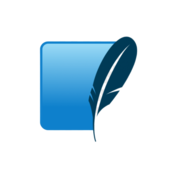
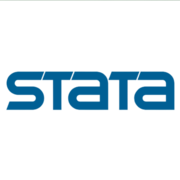
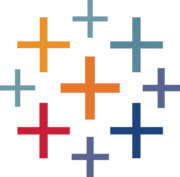
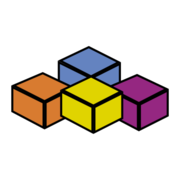