Prompt
Answer
Code Explanation
import math
float1 = 123.356
float2 = -2434.545
print(math.trunc(float1))
print(math.trunc(float2))
Overview
The provided code snippet is written in Python and performs the following steps:
- Importing the Math Module: It imports the
math
module, which includes various mathematical functions. - Variable Assignment: It assigns two floating-point numbers (
float1
andfloat2
) to variables. - Truncating Floats: It uses the
math.trunc()
function to truncate (i.e., remove the decimal part) these floating-point numbers and prints the results.
Detailed Breakdown
1. Importing the Math Module
import math
- Purpose: Makes the mathematical functions defined in the
math
module available for use in this script. Specifically, the script utilizes themath.trunc()
function.
2. Variable Assignment
float1 = 123.356
float2 = -2434.545
- Purpose: Defines two floating-point variables.
float1
has a value of123.356
.float2
has a value of-2434.545
.
3. Truncating Floats
print(math.trunc(float1))
print(math.trunc(float2))
- Purpose: Uses the
math.trunc()
function to truncate the floating-point numbers and print the results.math.trunc(float1)
: This function call removes the decimal part offloat1
(which is123.356
), resulting in123
.math.trunc(float2)
: Similarly, this function call removes the decimal part offloat2
(which is-2434.545
), resulting in-2434
.
Summary of math.trunc()
- Function:
math.trunc(x)
- Purpose: Truncates the decimal part of
x
and returns the integer part only. - Behavior:
- Positive floats are truncated down towards zero. Example:
math.trunc(123.356)
results in123
. - Negative floats also truncate towards zero. Example:
math.trunc(-2434.545)
results in-2434
.
- Positive floats are truncated down towards zero. Example:
Complete Code with Output
Below is the complete code along with the expected output:
import math
float1 = 123.356
float2 = -2434.545
print(math.trunc(float1)) # Output: 123
print(math.trunc(float2)) # Output: -2434
Additional Examples
To further illustrate the concept, consider the following variations:
import math
float3 = 56.789
float4 = -98.765
print(math.trunc(float3)) # Output: 56
print(math.trunc(float4)) # Output: -98
Key Concepts
- Truncation: The process of discarding the fractional part of a number without rounding. The
math.trunc()
function performs this operation. - Math Module: A standard Python library used for mathematical operations.
Learning Path
For a deeper understanding, consider exploring the following topics on the Enterprise DNA Platform:
- Introduction to Python Programming
- Working with the Math Module in Python
- Understanding Floating-Point Arithmetic in Python
Description
This Python code snippet demonstrates how to truncate the decimal part of floating-point numbers using the math.trunc()
function from the math module, enhancing understanding of number manipulation in programming.
More Code Explainers
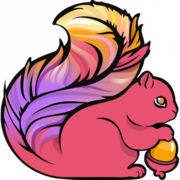
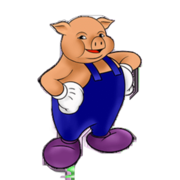
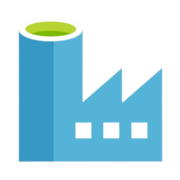
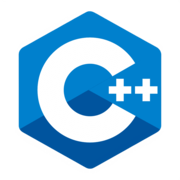
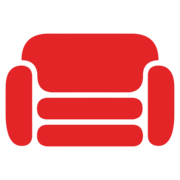
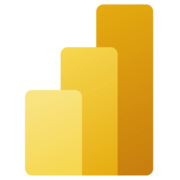
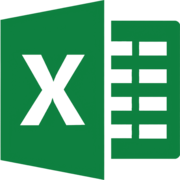
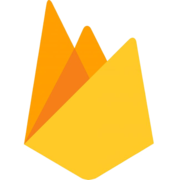
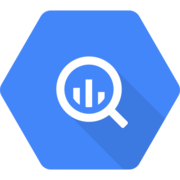
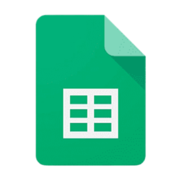
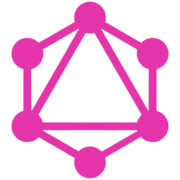
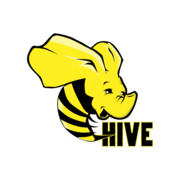
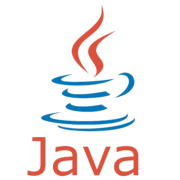
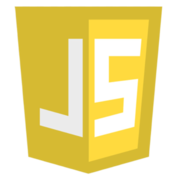
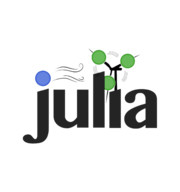
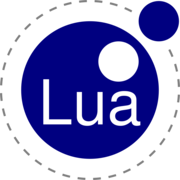
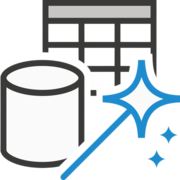
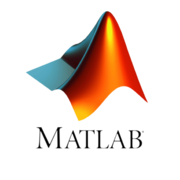
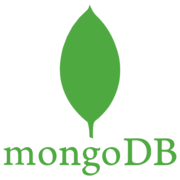
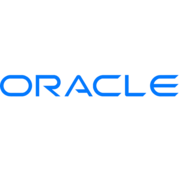
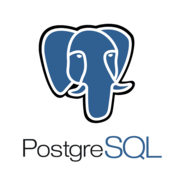
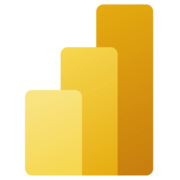
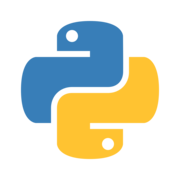
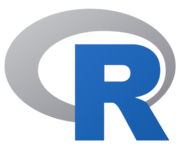
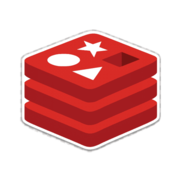
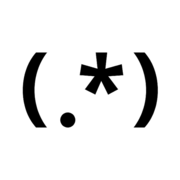
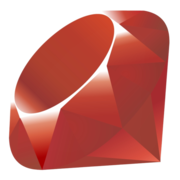
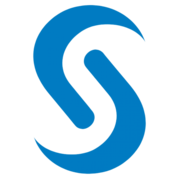
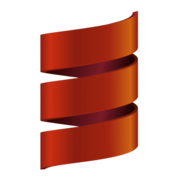
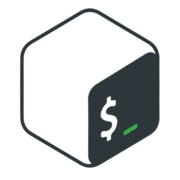
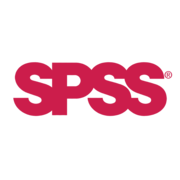
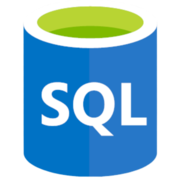
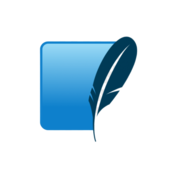
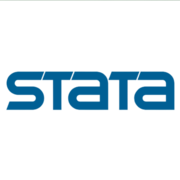
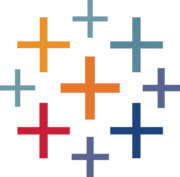
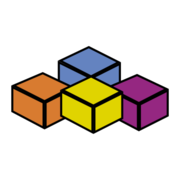