Prompt
Answer
digraph PuppeteerScript {
// Define nodes
Browser [label="Browser" shape=box];
Page1 [label="Page 1\nhttps://whatthesigma.com/" shape=box];
Page2 [label="Page 2\nhttp://giga.nigga.com" shape=box];
Page3 [label="Page 3\nLink from Page 1" shape=box];
AuthenticatePage1 [label="Authenticate\nlog1:pass1" shape=ellipse];
AuthenticatePage2 [label="Authenticate\nlog2:pass2" shape=ellipse];
WaitForSelector1 [label="Wait for Selector\n#content table tbody [...]" shape=ellipse];
ExtractText1 [label="Extract Text\n#content table tbody [...]" shape=ellipse];
ErrorHandling [label="Error Handling" shape=ellipse];
// Define relationships
Browser -> Page1 [label="newPage()"];
Browser -> Page2 [label="newPage()"];
Browser -> Page3 [label="newPage()"];
Page1 -> AuthenticatePage1 [label="authenticate()"];
Page1 -> "Wait for Selector" [label="waitForSelector()"];
Page1 -> "Extract Text" [label="evaluate()"];
AuthenticatePage1 -> "Wait for Selector";
"Wait for Selector" -> "Extract Text";
"Extract Text" -> Page3 [label="goto() with extracted link"];
Page2 -> AuthenticatePage2 [label="authenticate()"];
AuthenticatePage2 -> Page2 [label="goto()"];
// Error handling flow
subgraph cluster_Error {
label = "Error Handling";
ErrorHandling;
Page1 -> ErrorHandling [label="catch(error)"];
Page2 -> ErrorHandling [label="catch(error)"];
Page3 -> ErrorHandling [label="catch(error)"];
}
// Browser closing
subgraph cluster_Finally {
label = "Finally";
Browser -> ErrorHandling [label="close()"];
}
}
Explanation
- Nodes: Each major component and actions, such as
Browser
,Page 1
,Page 2
, authentication steps, etc., are represented as nodes. - Edges: The relationships between nodes are represented as directed edges, showcasing the flow of the program.
- Error Handling: A subgraph
cluster_Error
is created to visualize error handling in the script. - Browser Closing: If the browser is intended to close at the end, it is depicted under the
Finally
cluster.
This DOT notation graph provides a visual representation of the Puppeteer script, showing the flow and relationships of various components and actions in the code.
For more advanced data manipulation and visualization techniques, consider exploring courses on the Enterprise DNA Platform.
Description
This DOT notation diagram illustrates the components and interactions of a Puppeteer script, detailing browser actions, page navigation, authentication steps, error handling, and final browser closure, providing a clear visual representation of the script's flow.
More Code Visualizers
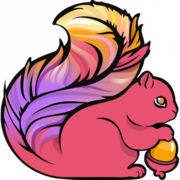
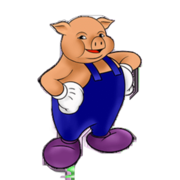
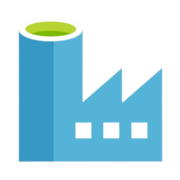
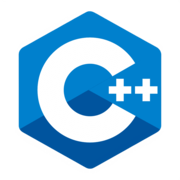
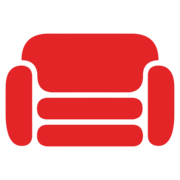
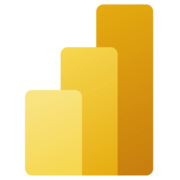
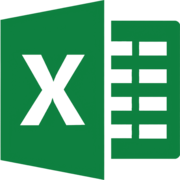
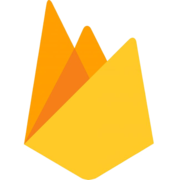
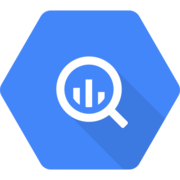
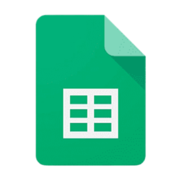
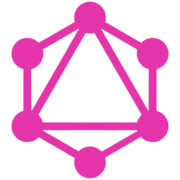
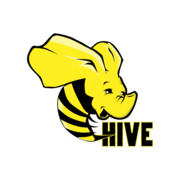
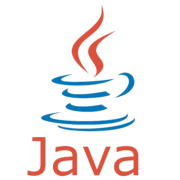
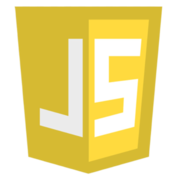
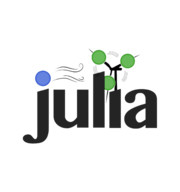
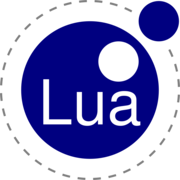
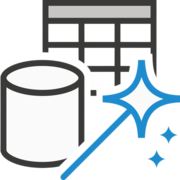
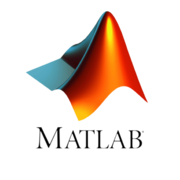
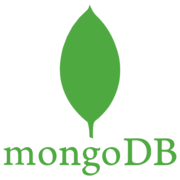
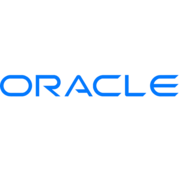
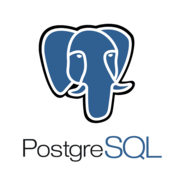
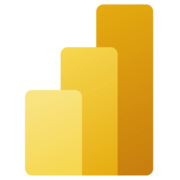
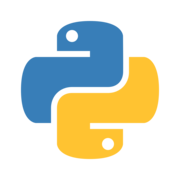
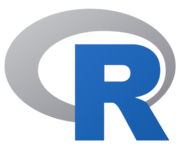
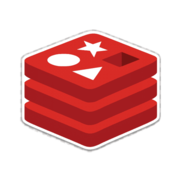
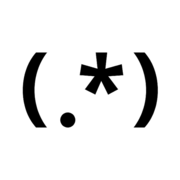
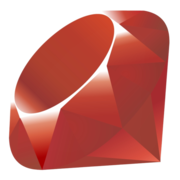
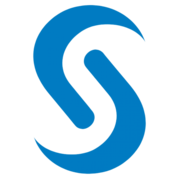
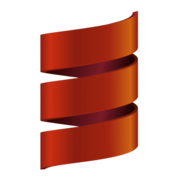
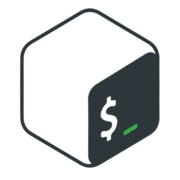
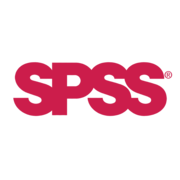
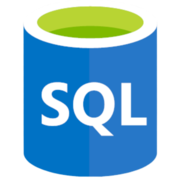
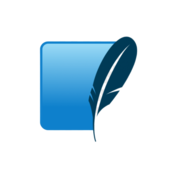
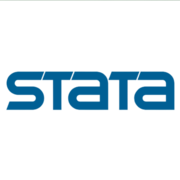
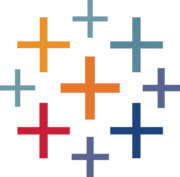
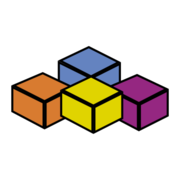