This Query related with thread "Mastering XGBoost: A Comprehensive Exploration"
Prompt
Answer
Text Explanation to Visual Representation
Objective
The task involves implementing and visualizing the logic of an XGBoost regression model for predicting house prices using the Boston housing dataset.
Key Components
- Import Libraries: Import essential Python libraries for XGBoost, data loading, splitting, and evaluation.
- Load Dataset: Load the Boston housing dataset.
- Split Data: Split the dataset into training and testing sets.
- Initialize Model: Set up the XGBoost regressor with specified parameters.
- Train Model: Train the model on the training data.
- Make Predictions: Predict values using the test data.
- Evaluate Model: Evaluate the predictions using Root Mean Squared Error (RMSE).
Visual Representation
The following flowchart provides a clear visual depiction of the outlined steps, illustrating the flow of logic and structure of the code.
Flowchart
flowchart TD
A[Import Libraries] --> B[Load Dataset]
B --> C[Split Data into Train/Test]
C --> D[Initialize XGBoost Model]
D --> E[Train the Model]
E --> F[Make Predictions]
F --> G[Evaluate Model with RMSE]
G --> H[Print RMSE]
Explanatory Comments for Flowchart Nodes
Import Libraries: Import libraries for data handling and model implementation.
xgboost
for the XGBoost model.sklearn.datasets
for loading the dataset.sklearn.model_selection
for splitting the dataset.sklearn.metrics
for model evaluation.
Load Dataset: Use the
load_boston()
function fromsklearn.datasets
to load the Boston housing dataset.Split Data: Utilize
train_test_split
fromsklearn.model_selection
to divide the dataset into training and testing sets (80% train, 20% test).Initialize XGBoost Model: Initialize an XGBoost regressor (
xgb.XGBRegressor
) with several parameters:objective='reg:squarederror'
: Specifies the learning task and corresponding objective.colsample_bytree=0.3
: Fraction of features to be used.learning_rate=0.1
: Step size shrinkage.max_depth=5
: Maximum depth of a tree.alpha=10
: L1 regularization term on weights.n_estimators=10
: Number of trees in the model.
Train the Model: Fit the model on the training data using
xg_reg.fit(X_train, y_train)
.Make Predictions: Use the trained model to make predictions on the test data with
xg_reg.predict(X_test)
.Evaluate Model: Use
mean_squared_error
withsquared=False
to compute the RMSE of the predictions compared to the actual test labels.Print RMSE: Output the computed RMSE to assess model performance.
Code Implementation (Python)
# Import necessary libraries
import xgboost as xgb
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# Load dataset
data = load_boston()
X, y = data.data, data.target
# Split data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize XGBoost model
xg_reg = xgb.XGBRegressor(objective ='reg:squarederror', colsample_bytree = 0.3, learning_rate = 0.1,
max_depth = 5, alpha = 10, n_estimators = 10)
# Train the model
xg_reg.fit(X_train, y_train)
# Make predictions
preds = xg_reg.predict(X_test)
# Evaluate the model
rmse = mean_squared_error(y_test, preds, squared=False)
print(f"RMSE: {rmse}")
Summary
This flowchart and detailed explanation provide a succinct yet comprehensive guide to understanding the logic and structure of implementing an XGBoost regressor for predicting house prices using the Boston housing dataset. For further learning, consider leveraging educational resources available on the Enterprise DNA Platform.
Description
This guide details the implementation of an XGBoost regression model to predict Boston housing prices, including data preparation, model training, and evaluation through RMSE, complemented with a flowchart for visual clarity.
More Logic Visualizers
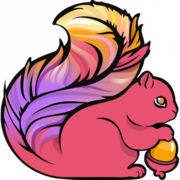
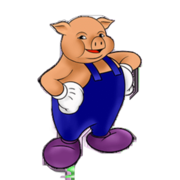
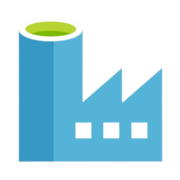
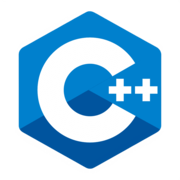
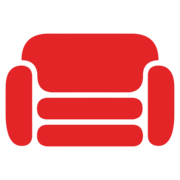
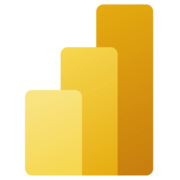
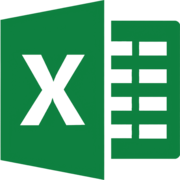
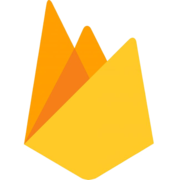
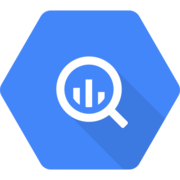
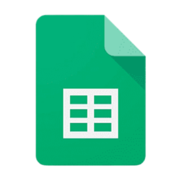
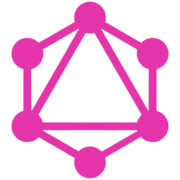
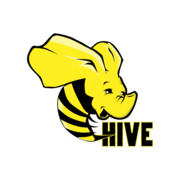
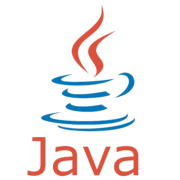
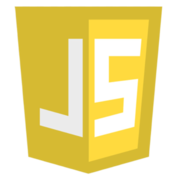
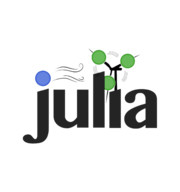
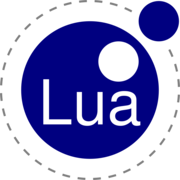
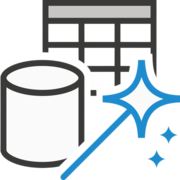
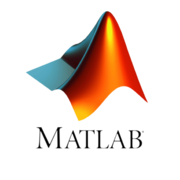
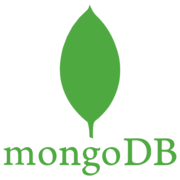
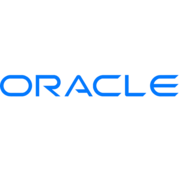
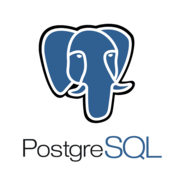
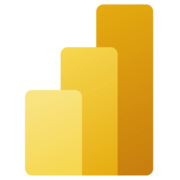
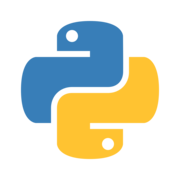
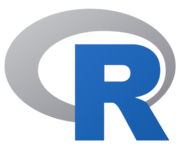
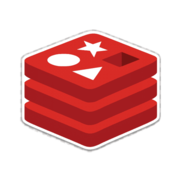
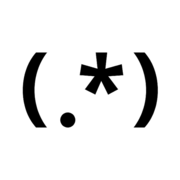
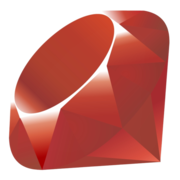
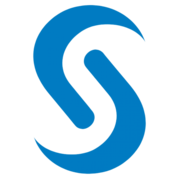
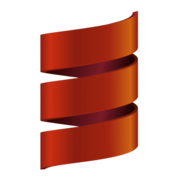
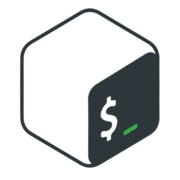
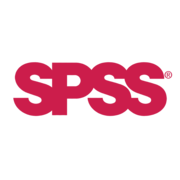
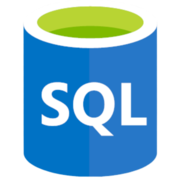
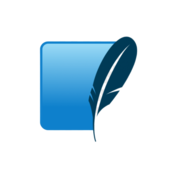
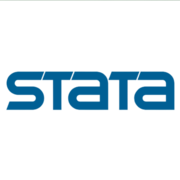
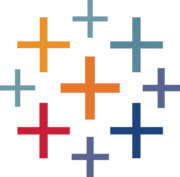
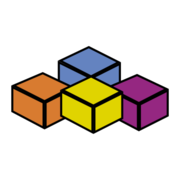