Prompt
Answer
Code Inspection and Bug Identification
1. Import Statements
- No issues detected with the import statements, except that some are unused (
sys
,datetime
,parser
, andpytz
).
2. Class Definition and Initialization
LOFDetector
class and its initializer (__init__
) method seem correctly defined and registered.
3. get_vector_df
Method
- No issues detected.
4. preprocess_idia_logs
Method
Bug Detected:
- Using
view(np.int64)
can cause unexpected results in newer pandas versions. It's better to use.astype
for safer operation.
Fix:
- Replace
log_features["eventTime"].view(np.int64) // 10**6
withlog_features["eventTime"].astype(np.int64) // 10**6
.
log_features["eventTime"] = log_features["eventTime"].astype(np.int64) // 10**6
5. detect_anomalies
Method
Bug Detected:
- Flattening the array might cause incorrect input for the
TfidfVectorizer
which expects iterable of text-like records rather than a single long string.
Fix:
- Ensure the input format for
fit_transform
is as expected.
log_vectors = self.get_vector_df(preprocessed_data.astype(str))
6. lime_explain_instance
Method
Bug Detected:
- The function
reshape
is incorrectly referenced. reshape
should be used after converting the DataFrame to a numpy array.
Fix:
- Convert
instance_vector
to a numpy array before reshaping.
instance_vector_2d = instance_vector.values.reshape(1, -1)
7. visualize_anomalies
Method
Bug Detected:
- String formatting for title has a syntax error (
\
).
Fix:
- Correct string formatting.
plt.title(f'Comparison of Anomaly Scores with {feature}')
8. human_readable_insights
Method
Bug Detected:
- Syntax error in the string formatting method.
Fix:
- Correct string formatting within the list comprehension.
insights = ["Feature: {}, Contribution: {}".format(feature, contribution) for feature, contribution in explanation]
9. shap_explain
Function
Bug Detected:
- Using
KernelExplainer
might produce incorrect results ifpredict_func
is not returning the expected format.
Fix:
- Ensure
predict_func
returns the correct format for SHAP.
def predict_func(X):
return model.model.decision_function(X)
10. plot_shap_values
Function
Bug Detected:
- Not directly referencing the
feature_names
of DataFrame columns might cause inconsistency.
Fix:
- Explicitly get feature names if needed.
shap.summary_plot(shap_values, log_vectors, feature_names=log_vectors.columns)
Final Code with Fixes
import json
import numpy as np
import pandas as pd
from sklearn.neighbors import LocalOutlierFactor
from sklearn.feature_extraction.text import TfidfVectorizer
import matplotlib.pyplot as plt
import seaborn as sns
import lime.lime_tabular
import shap
from shap import KernelExplainer
from logai.algorithms.algo_interfaces import AnomalyDetectionAlgo
from logai.config_interfaces import Config
from logai.algorithms.factory import factory
from idia_logai.LOFParams import LOFParams
@factory.register("detection", "lof", LOFParams)
class LOFDetector(AnomalyDetectionAlgo):
def __init__(self, params: LOFParams):
self.model = LocalOutlierFactor(
n_neighbors=params.n_neighbors,
algorithm=params.algorithm,
leaf_size=params.leaf_size,
metric=params.metric,
p=params.p,
metric_params=params.metric_params,
contamination=params.contamination,
novelty=params.novelty,
n_jobs=params.n_jobs,
)
self.vectorizer = TfidfVectorizer(max_features=100)
def get_vector_df(self, log_features):
tfidf_matrix = self.vectorizer.fit_transform(log_features).toarray()
tfidf_df = pd.DataFrame(tfidf_matrix, columns=self.vectorizer.get_feature_names_out())
return tfidf_df
def preprocess_idia_logs(self, log_data: pd.DataFrame) -> pd.DataFrame:
log_features = log_data[["eventTime", "severity"]].copy()
log_features["eventTime"] = pd.to_datetime(log_features["eventTime"], errors='coerce')
log_features = log_features.dropna(subset=["eventTime"])
log_features["eventTime"] = log_features["eventTime"].astype(np.int64) // 10**6
return log_features
def detect_anomalies(self, log_data: pd.DataFrame) -> pd.DataFrame:
preprocessed_data = self.preprocess_idia_logs(log_data)
log_vectors = self.get_vector_df(preprocessed_data.astype(str))
log_data["anom_score"] = self.model.fit_predict(log_vectors)
return log_data
def lime_explain_instance(self, instance: pd.DataFrame):
preprocessed_instance = self.preprocess_idia_logs(instance)
instance_vector = self.get_vector_df(preprocessed_instance.astype(str))
instance_vector_2d = instance_vector.values.reshape(1, -1)
training_data = self.vectorizer.transform(preprocessed_instance.astype(str)).toarray()
def predict_func(X):
return self.model.decision_function(X)
explainer = lime.lime_tabular.LimeTabularExplainer(
training_data=training_data,
feature_names=self.vectorizer.get_feature_names_out(),
verbose=True,
mode="regression"
)
explanation = explainer.explain_instance(
data_row=instance_vector_2d[0],
predict_fn=predict_func
)
return explanation
def visualize_anomalies(self, log_data: pd.DataFrame, feature: str):
plt.figure(figsize=(10, 6))
sns.boxplot(x=log_data[feature], y=log_data['anom_score'])
plt.title(f'Comparison of Anomaly Scores with {feature}')
return plt.gcf()
def human_readable_insights(self, explanation: list):
insights = ["Feature: {}, Contribution: {}".format(feature, contribution) for feature, contribution in explanation]
return insights
def shap_explain(model, log_data: pd.DataFrame):
sample_data = log_data.sample(n=100)
log_features = model.preprocess_idia_logs(sample_data)
log_vectors = model.get_vector_df(log_features.astype(str))
def predict_func(X):
return model.model.decision_function(X)
explainer = KernelExplainer(predict_func, log_vectors)
shap_values = explainer.shap_values(log_vectors)
return shap_values, log_vectors
def plot_shap_values(shap_values, log_vectors):
shap.summary_plot(shap_values, log_vectors, feature_names=log_vectors.columns)
return plt.gcf()
Recommendation
For further learning and improving your skills, consider courses offered on the Enterprise DNA Platform.
Description
This analysis provides a detailed inspection of the LOFDetector class code, identifying bugs and suggesting fixes for methods including preprocessing, anomaly detection, LIME, and SHAP explainability, ensuring robust performance in anomaly detection tasks.
More Bug Detectors
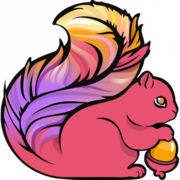
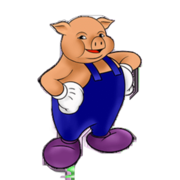
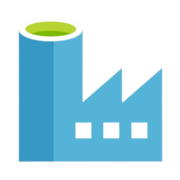
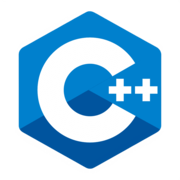
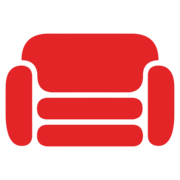
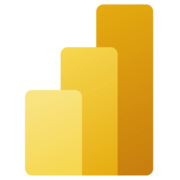
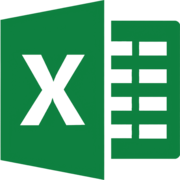
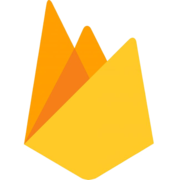
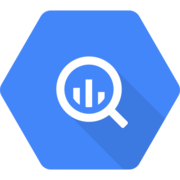
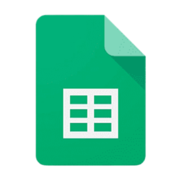
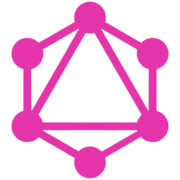
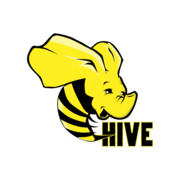
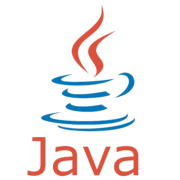
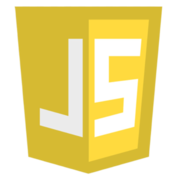
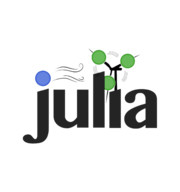
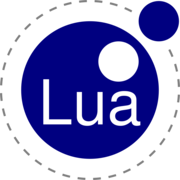
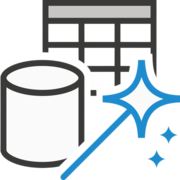
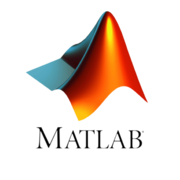
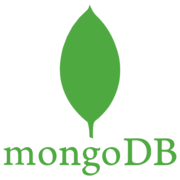
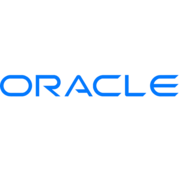
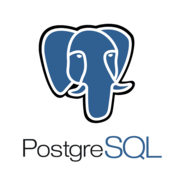
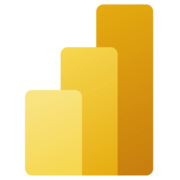
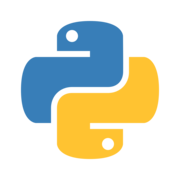
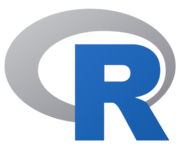
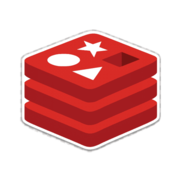
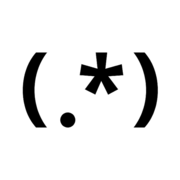
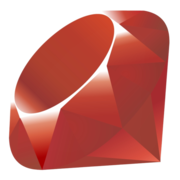
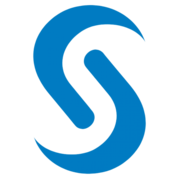
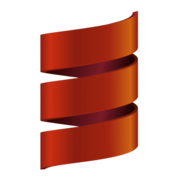
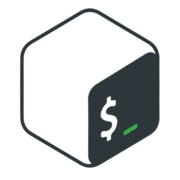
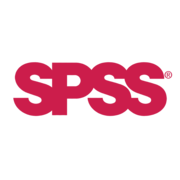
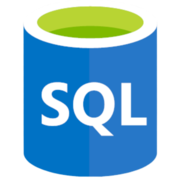
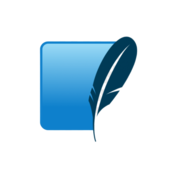
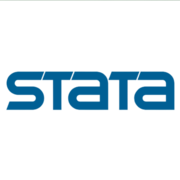
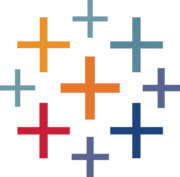
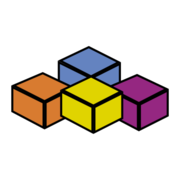