Prompt
Answer
API Endpoint Structure
Overview
Based on the provided code snippet, a single GET endpoint will be created to retrieve data from https://api.example.com/data
.
Endpoint Details
- Method: GET
- Endpoint:
https://api.example.com/data
- Headers:
Accept: application/json
Authorization: Bearer YOUR_ACCESS_TOKEN
API Endpoint Code
Below is a sample Python Flask code snippet to create the API endpoint:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/data', methods=['GET'])
def get_data():
# Simulate data retrieval logic
sample_data = {'example_key': 'example_value'}
return jsonify(sample_data)
if __name__ == '__main__':
app.run()
Testing the Endpoint
You can test the created endpoint using tools like Postman or by running the Python script and sending a GET request to http://127.0.0.1:5000/data
(assuming the script is running locally).
Authorization
For implementation of authorization with Bearer token, you can validate the token before processing the request in the get_data
function.
Improvements
- Implement actual data retrieval logic based on the provided API URL.
- Add error handling for cases where data retrieval fails.
- Enhance security measures like rate-limiting, input validation, etc.
By following this structure, you can integrate the API endpoint into your web application to fetch and display data securely.
Description
Learn how to create a single GET API endpoint in Python Flask to retrieve data from a specific URL and implement authorization with Bearer token. Test the endpoint and consider improvements for better functionality.
More API Endpoint Generators
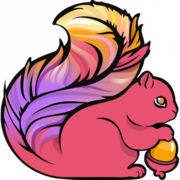
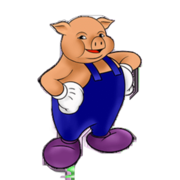
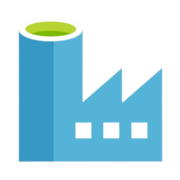
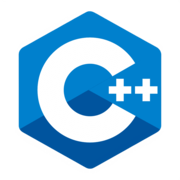
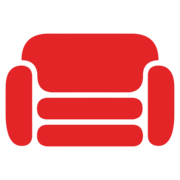
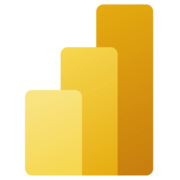
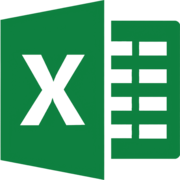
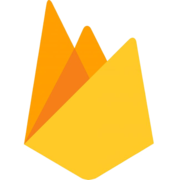
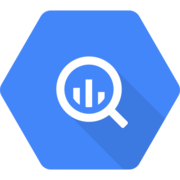
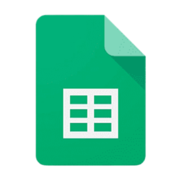
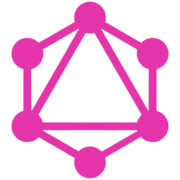
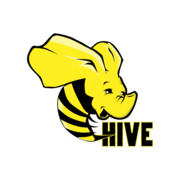
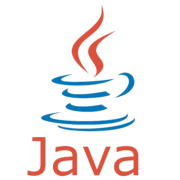
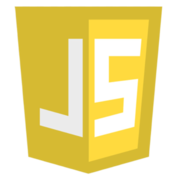
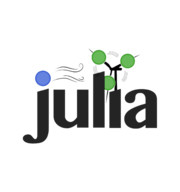
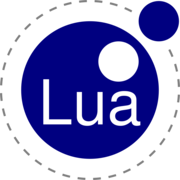
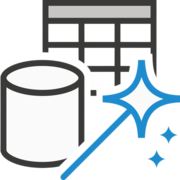
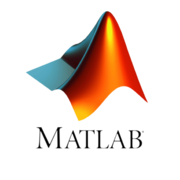
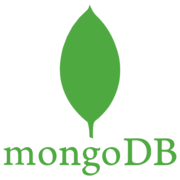
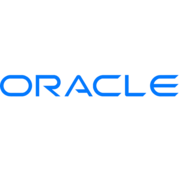
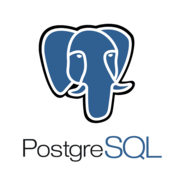
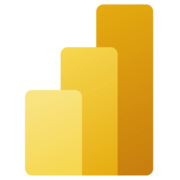
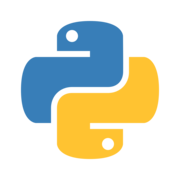
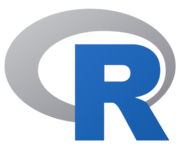
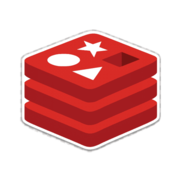
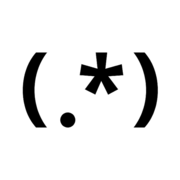
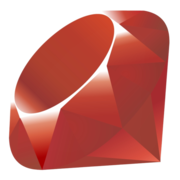
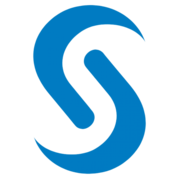
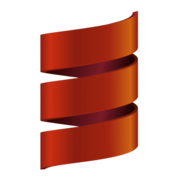
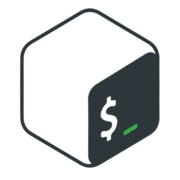
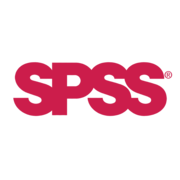
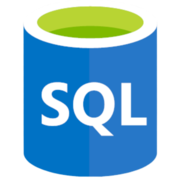
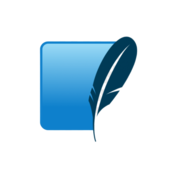
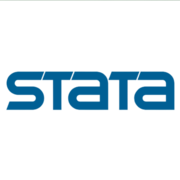
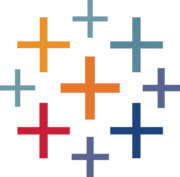
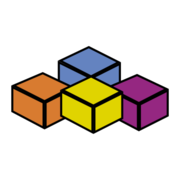