Code Generator | VBA
Error Handling and Exception Reporting in VBA
Managing error handling and exception reporting is crucial in VBA macros to ensure smooth code execution and easy debugging. By implementing error handling techniques, developers can anticipate and handle unexpected errors gracefully, improving the r...

Prompt
Answer
Error Handling and Exception Reporting in VBA
Managing error handling and exception reporting is crucial in VBA macros to ensure smooth code execution and easy debugging. By implementing error handling techniques, developers can anticipate and handle unexpected errors gracefully, improving the reliability and robustness of their code. Here are some best practices to efficiently manage error handling and exception reporting in VBA:
Enable Error Handling: Enable error handling in VBA by using the
On Error
statement. This allows the code to handle runtime errors and prevents the application from crashing.Use Specific Error Handlers: Use specific error handlers to handle different types of errors. You can define error handlers using the
On Error GoTo
statement followed by a label.Handle Critical Errors: Separate critical errors from non-critical errors. For critical errors, provide appropriate error messages to the user and exit the code gracefully.
Log Errors: Implement error logging to record error details such as error number, error description, source of the error, and timestamp. This helps in debugging the code and identifying recurring errors.
Avoid Silent Failures: Avoid silent failures where the error is ignored and not reported to the user. Always handle errors and display meaningful error messages to the user.
Clean Up Resources: Use the
On Error GoTo 0
statement to turn off error handling and release any resources (e.g. objects, file handles) that were used in the error handling code.Test Error Handling Code: Test the error handling code by intentionally causing errors. This ensures that the error handling code is working as expected and provides a robust solution.
Handle Unexpected Errors: Handle unexpected errors by using a generic error handler at the top level of the code. This captures any unhandled errors and logs them for further investigation.
By following these best practices, developers can effectively manage error handling and exception reporting in VBA macros, resulting in more reliable, maintainable, and easily debuggable code.
Example usage: Consider a VBA macro that reads data from an external file. By implementing error handling and exception reporting, the macro can gracefully handle errors such as file not found, invalid file format, or read/write errors. Instead of crashing or displaying cryptic error messages, the macro can provide meaningful error messages to the user and log the error details for further investigation.
Description
This project focuses on best practices for error handling and exception reporting in VBA macros. It covers enabling error handling, using specific error handlers, handling critical errors, logging errors, avoiding silent failures, cleaning up resources, testing error handling code, and handling unexpected errors. By following these best practices, developers can effectively manage error handling and exception reporting in VBA macros, resulting in more reliable, maintainable, and easily debuggable code. An example usage is provided where a VBA macro reads data from an external file and handles errors such as file not found, invalid file format, or read/write errors gracefully, providing meaningful error messages to the user and logging error details for further investigation.
More Code Generators
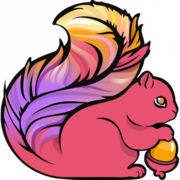
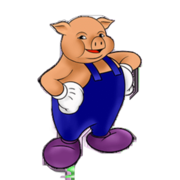
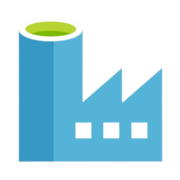
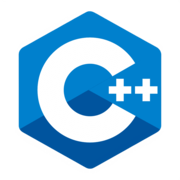
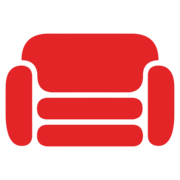
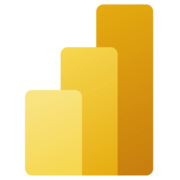
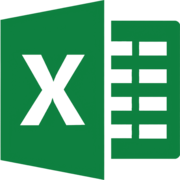
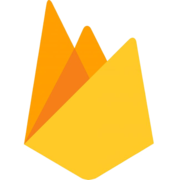
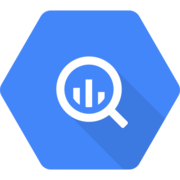
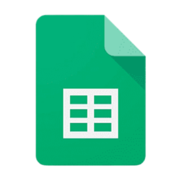
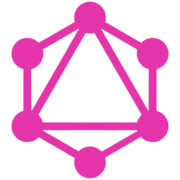
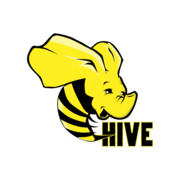
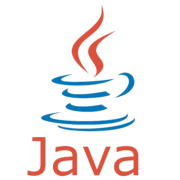
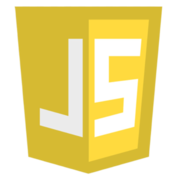
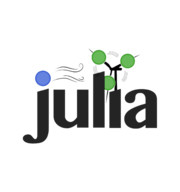
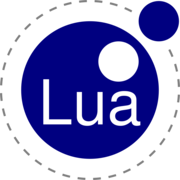
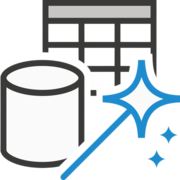
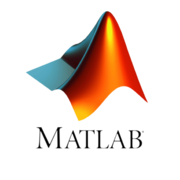
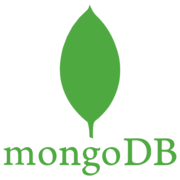
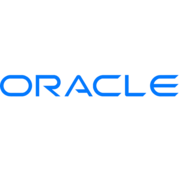
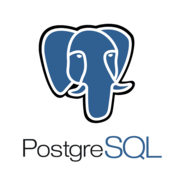
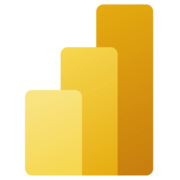
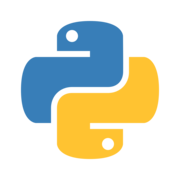
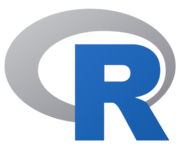
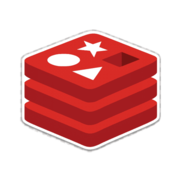
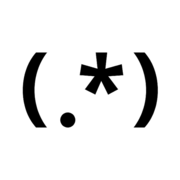
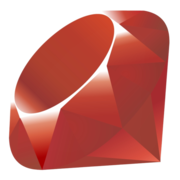
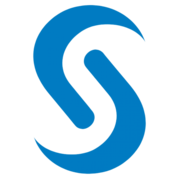
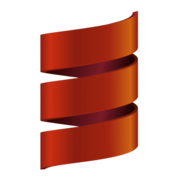
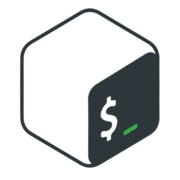
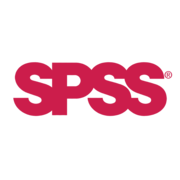
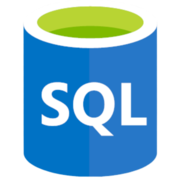
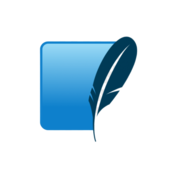
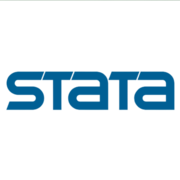
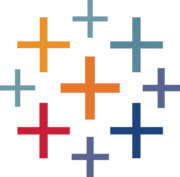
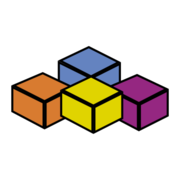