This Query related with thread "Comprehensive R Programming Resource"
Prompt
Answer
Uploading Data into R Environment
Overview
Uploading data into the R environment is a fundamental step in data analysis. Various data formats can be imported into R, such as CSV, Excel, and databases. This guide outlines the main methods for loading data.
Common Methods
1. Loading CSV Files
CSV is among the most common file formats.
Using readr
Package
# R
# Install and load the readr package
install.packages("readr")
library(readr)
# Use read_csv function to read a CSV file
data_frame <- read_csv("path/to/your/file.csv")
Using Base R
# R
# Use read.csv function in base R
data_frame <- read.csv("path/to/your/file.csv", header = TRUE, sep = ",")
2. Loading Excel Files
To read Excel files, the readxl
package is very effective.
Using readxl
Package
# R
# Install and load the readxl package
install.packages("readxl")
library(readxl)
# Use read_excel function to read an Excel file
data_frame <- read_excel("path/to/your/file.xlsx", sheet = 1)
3. Loading Data from Databases
For database interaction, the DBI
package in combination with a specific database driver is commonly used.
Using DBI
Package
# R
# Install and load the DBI and RSQLite packages
install.packages(c("DBI", "RSQLite"))
library(DBI)
library(RSQLite)
# Establish a connection to the SQLite database
con <- dbConnect(RSQLite::SQLite(), "path/to/your/database.sqlite")
# Query data from a table
data_frame <- dbGetQuery(con, "SELECT * FROM tablename")
# Disconnect from the database
dbDisconnect(con)
4. Loading Text Files
Text files can also be loaded in a similar manner to CSV files by specifying delimiters.
Using readr
Package
# R
# Use read_delim function in the readr package
data_frame <- read_delim("path/to/your/file.txt", delim = "\t")
5. Loading Web Data
Data from the web can be fetched using the httr
and rvest
packages.
Using httr
and rvest
Packages
# R
# Install and load the httr and rvest packages
install.packages(c("httr", "rvest"))
library(httr)
library(rvest)
# Fetch HTML content from a webpage
webpage <- read_html("http://example.com")
# Extract desired data using appropriate rvest functions
data_frame <- webpage %>%
html_nodes("css_selector") %>%
html_text()
Conclusion
These methods cover the most common ways to upload data into the R environment. Each method has its advantages, and the choice depends on the source and format of your data. For more advanced techniques, consider exploring further courses and resources available on the Enterprise DNA platform.
Description
This guide covers various methods for importing data into the R environment, including loading CSV, Excel files, databases, text files, and web data. Each method is illustrated with sample code for practical implementation.
More Languages Advisors
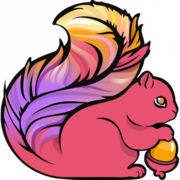
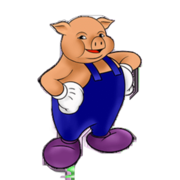
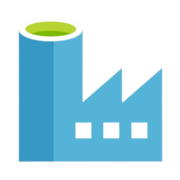
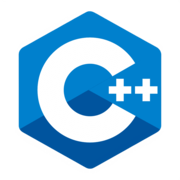
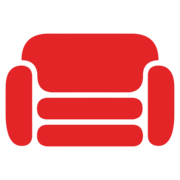
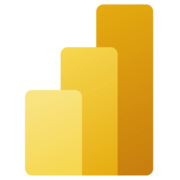
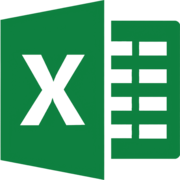
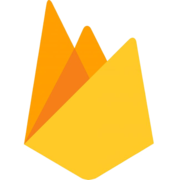
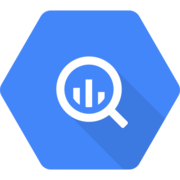
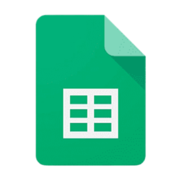
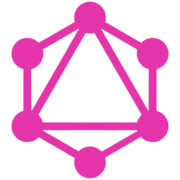
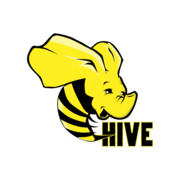
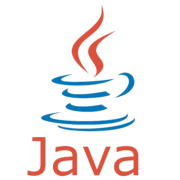
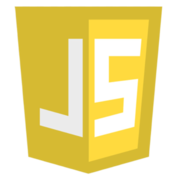
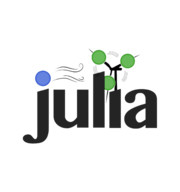
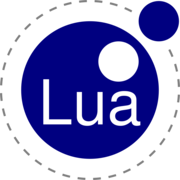
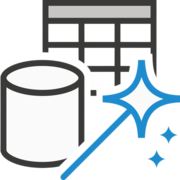
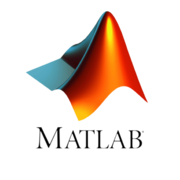
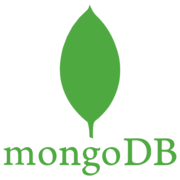
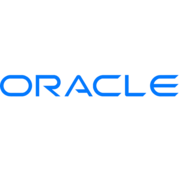
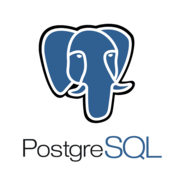
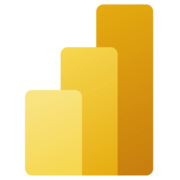
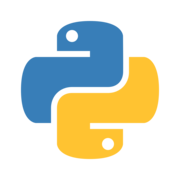
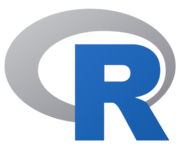
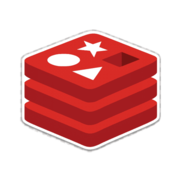
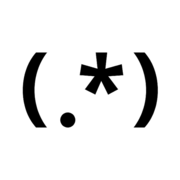
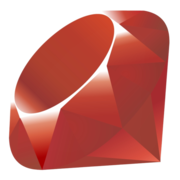
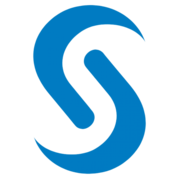
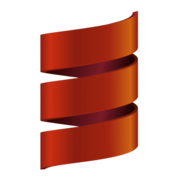
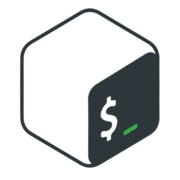
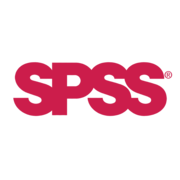
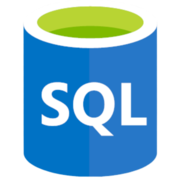
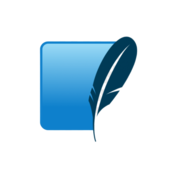
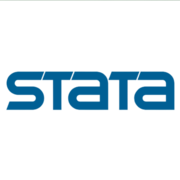
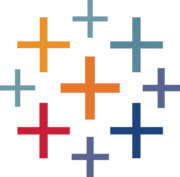
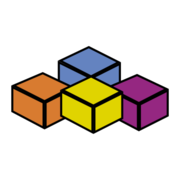