Code Generator | Python
generate_text_with_huggingface
This function employs Hugging Face models for generative AI projects, using a specified model to generate text based on a prompt. It includes parameters for model name and prompt, with error handling for invalid inputs. An example usage is provided

Prompt
Answer
Function to Use Hugging Face Models for Generative AI Projects
Purpose: This function is designed to utilize Hugging Face models for generative AI projects. It will take a prompt as input and generate text based on the prompt using the selected Hugging Face model.
Parameters:
- model_name: The name of the Hugging Face model to be used for text generation
- prompt: The prompt based on which the text will be generated
Return Type: String - Generated text based on the provided prompt
Exceptions: This function will raise an exception if the model_name is invalid or if there are any issues with the text generation process.
All Necessary Imports: The function will require the following imports:
from transformers import pipeline
Code:
def generate_text_with_huggingface(model_name, prompt):
try:
# Load the specified Hugging Face model for text generation
text_generator = pipeline('text-generation', model=model_name)
# Generate text based on the prompt using the loaded model
generated_text = text_generator(prompt, max_length=50, num_return_sequences=1, do_sample=True)[0]['generated_text']
return generated_text
except Exception as e:
raise Exception("An error occurred during text generation: " + str(e))
Code Usage Example:
generated_text = generate_text_with_huggingface('distilgpt2', 'Once upon a time')
print(generated_text)
This code will use the 'distilgpt2' model to generate text based on the prompt "Once upon a time" and then print the generated text.
Description
This function employs Hugging Face models for generative AI projects, using a specified model to generate text based on a prompt. It includes parameters for model name and prompt, with error handling for invalid inputs. An example usage is provided for clarity.
More Code Generators
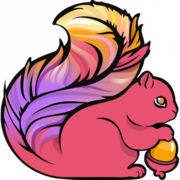
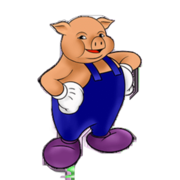
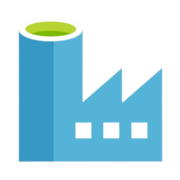
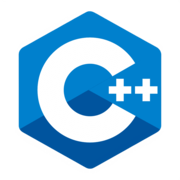
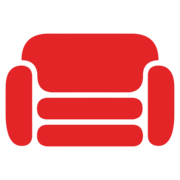
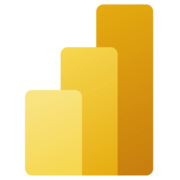
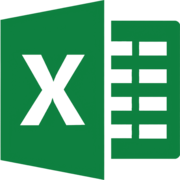
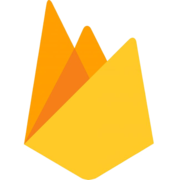
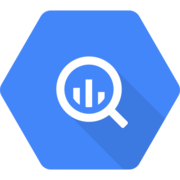
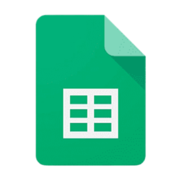
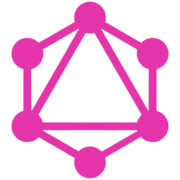
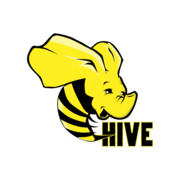
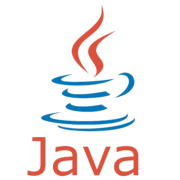
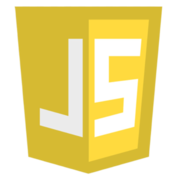
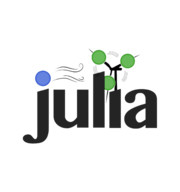
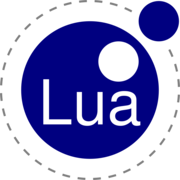
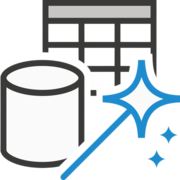
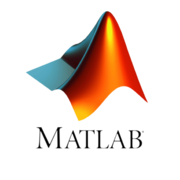
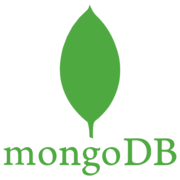
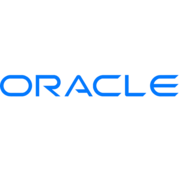
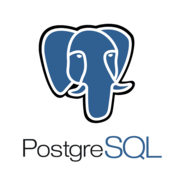
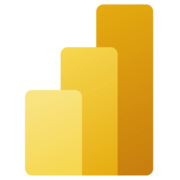
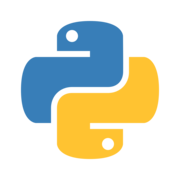
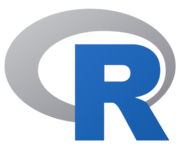
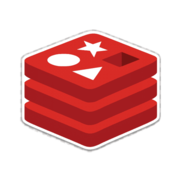
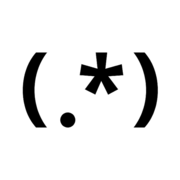
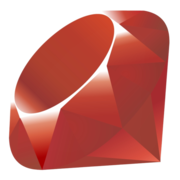
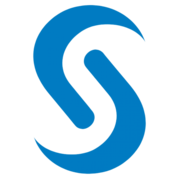
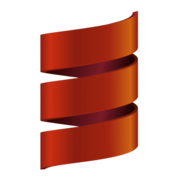
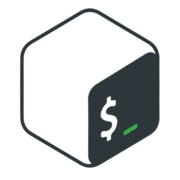
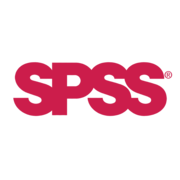
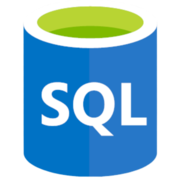
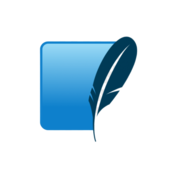
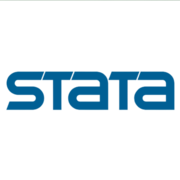
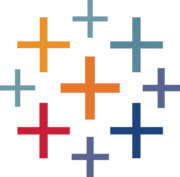
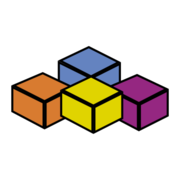