Bug Detector | Lua
Lua Code Inspection and Bug Fix for ClickDetector
This document reviews a Lua script for Roblox, identifying issues related to the ClickDetector setup and the retrieval of a tool from ServerStorage. It provides fixes for variable initialization, event connections, error handling, and

Prompt
Answer
Code Inspection and Bug Fix
Below is the provided code snippet for inspection and debugging:
local Model = script.Parent
local Button = Model.ClickDetector
local tool = game.ServerStorage["Scientist Keycard"]
--
Button:WaitForChild("ClickDetector").MouseClick:Connect(function(plr)
if not plr or not plr.Character or plr.Character.Parent == nil then return end
if not plr.Backpack:FindFirstChild(tool.Name) and not plr.Character:FindFirstChild(tool.Name) then
tool:Clone().Parent = plr.Backpack
end
end)
Identified Issues and Fixes
Incorrect Initialization of
Button
The
Button
variable is incorrectly assigned directly as theClickDetector
.ClickDetector
is the component we want to interact with, and it should be referenced correctly in theMouseClick
event.Fix
Reference the
ClickDetector
directly usingWaitForChild
to ensure it is available:local Model = script.Parent local Button = Model:WaitForChild("ClickDetector")
Unnecessary
WaitForChild
in ButtonThe line
Button:WaitForChild("ClickDetector").MouseClick:Connect
is redundant. SinceButton
is already referring to theClickDetector
, onlyMouseClick
event needs to be connected.Fix
Connect directly to the
MouseClick
event fromButton
:Button.MouseClick:Connect(function(plr)
Tool Retrieval from
ServerStorage
The retrieval of the
Scientist Keycard
fromServerStorage
might fail if the tool doesn't exist. Adding error handling can ensure the script doesn't encounter runtime failures.Fix
Ensure error handling is in place:
local tool = game.ServerStorage:FindFirstChild("Scientist Keycard") if not tool then warn("Scientist Keycard tool is not found in ServerStorage") return end
Nil Check for
plr.Character.Parent
The check
plr.Character.Parent == nil
is redundant ifplr.Character
is already validated. Ifplr.Character
is not nil, itsParent
will inherently be valid.Fix
Remove the redundant nil check:
if not plr or not plr.Character then return end
Complete Fixed Code
Below is the modified code with the fixes applied:
local Model = script.Parent
local Button = Model:WaitForChild("ClickDetector")
local tool = game.ServerStorage:FindFirstChild("Scientist Keycard")
if not tool then
warn("Scientist Keycard tool is not found in ServerStorage")
return
end
Button.MouseClick:Connect(function(plr)
if not plr or not plr.Character then return end
if not plr.Backpack:FindFirstChild(tool.Name) and not plr.Character:FindFirstChild(tool.Name) then
tool:Clone().Parent = plr.Backpack
end
end)
Additional Recommendations
To improve your coding skills further, you can consider taking programming courses on the Enterprise DNA Platform, which offers a range of resources to deepen your understanding of coding best practices and debugging techniques.
Description
This document reviews a Lua script for Roblox, identifying issues related to the ClickDetector setup and the retrieval of a tool from ServerStorage. It provides fixes for variable initialization, event connections, error handling, and redundant checks, enhancing overall code reliability and functionality.
More Bug Detectors
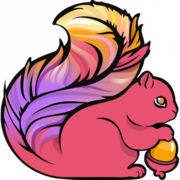
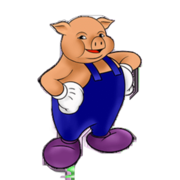
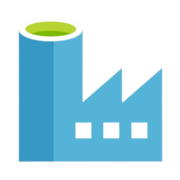
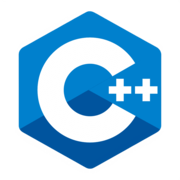
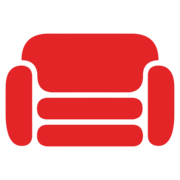
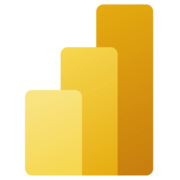
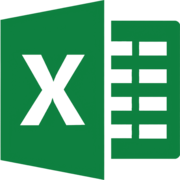
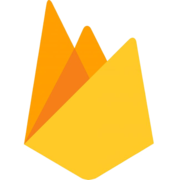
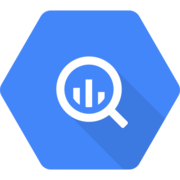
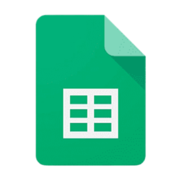
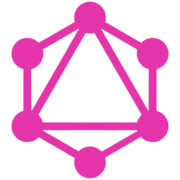
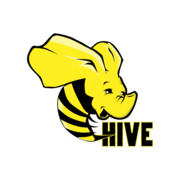
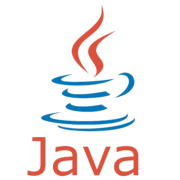
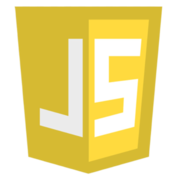
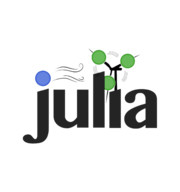
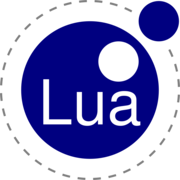
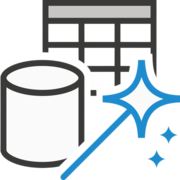
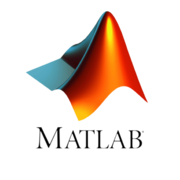
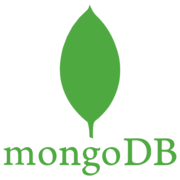
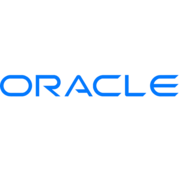
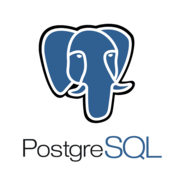
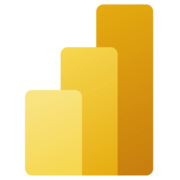
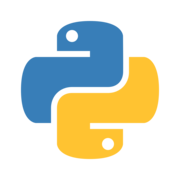
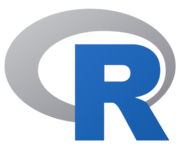
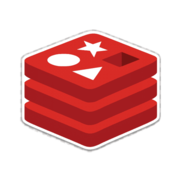
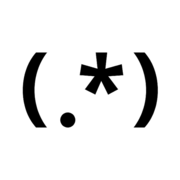
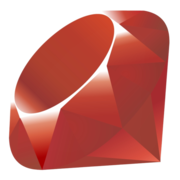
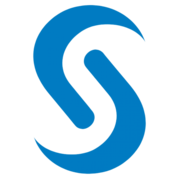
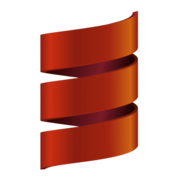
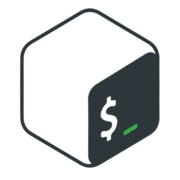
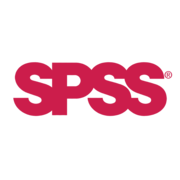
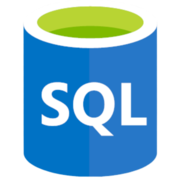
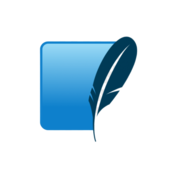
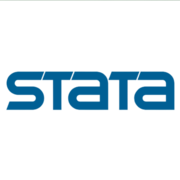
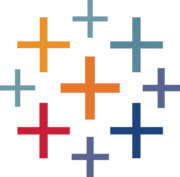
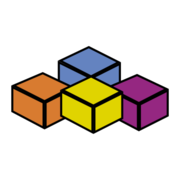