Code Generator
Optimization techniques for k-means clustering algorithm in R
This guide provides several techniques to optimize the performance of k-means clustering algorithm in R. It includes steps such as data normalization, dimension reduction, algorithm selection, parameter tuning, parallel computing, and memory usage op...

Prompt
Answer
To optimize a k-means clustering algorithm in R for better performance:
Normalize the dataset: Scaling the data can help improve the convergence rate of the algorithm.
Reduce dimensions: If the dataset has a high number of dimensions, consider using dimensionality reduction techniques like Principal Component Analysis (PCA) to reduce the number of features while preserving the most important information.
Use the Hartigan-Wong algorithm: Instead of the default "cluster" function, use the "flexclust" package with the Hartigan-Wong algorithm for faster convergence.
Set appropriate values for the "nstart" and "iter.max" parameters: Increasing the number of random starts ("nstart") and iterations ("iter.max") can help improve the algorithm's performance, but keep in mind that it will also increase the computation time.
Parallelize computations: Utilize parallel processing to distribute the workload across multiple cores or machines, and consider using the "foreach" package in combination with the "doParallel" or "doSNOW" backend.
Optimize memory usage: If memory is a constraint, consider using algorithms that can handle large datasets, such as mini-batch k-means or online k-means.
Example usage:
# Load required libraries
library(flexclust)
# Normalize the dataset
normalized_data <- scale(data)
# Use the Hartigan-Wong algorithm
kmeans_model <- kcca(normalized_data, k = 3, family = "kmeans")
# Increase the number of random starts and iterations
kmeans_model <- kcca(normalized_data, k = 3, family = "kmeans", nstart = 10, iter.max = 100)
# Parallelize computations
library(doParallel)
library(foreach)
# Set up parallel backend
cl <- makeCluster(4)
registerDoParallel(cl)
# Run k-means in parallel
kmeans_model <- foreach(i = 1:10, .combine = combine, .packages = "flexclust") %dopar% {
kcca(normalized_data, k = 3, family = "kmeans", nstart = 10, iter.max = 100)
}
# Stop parallel backend
stopCluster(cl)
Remember to measure the performance improvements and adjust the optimization techniques according to your specific requirements and constraints.
Description
This guide presents various strategies to enhance the performance of the k-means clustering algorithm in R. The techniques discussed include data normalization to improve convergence, dimensionality reduction using techniques like PCA, utilizing the Hartigan-Wong algorithm for faster convergence, tuning parameters such as 'nstart' and 'iter.max', parallelizing computations to distribute workload, and optimizing memory usage for large datasets. With these optimization techniques, the performance of the k-means clustering algorithm can be significantly improved in terms of speed and accuracy.
More Code Generators
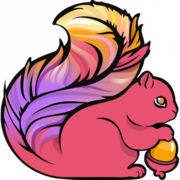
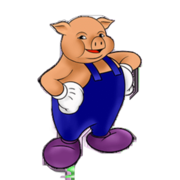
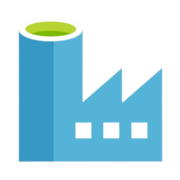
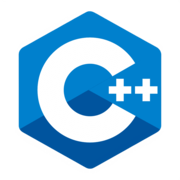
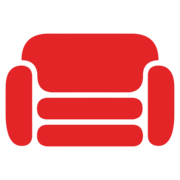
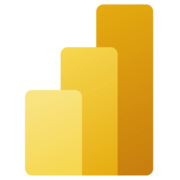
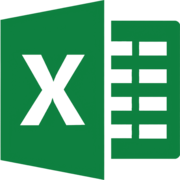
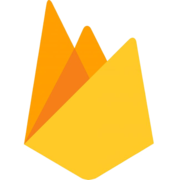
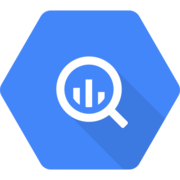
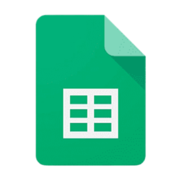
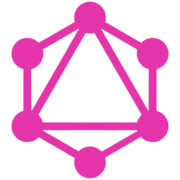
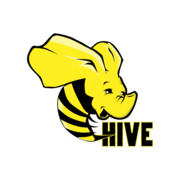
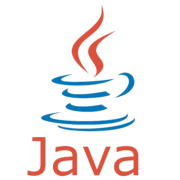
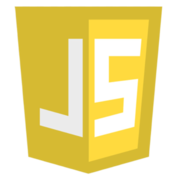
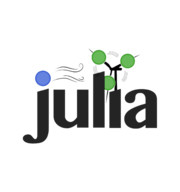
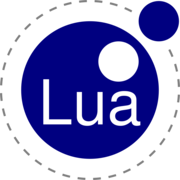
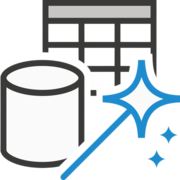
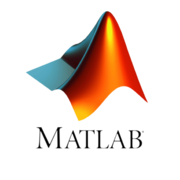
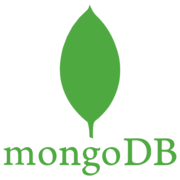
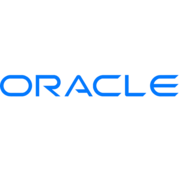
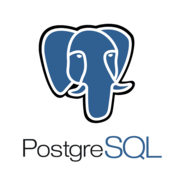
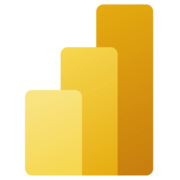
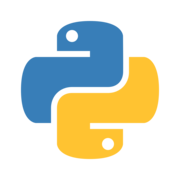
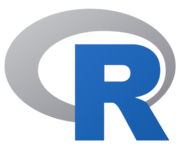
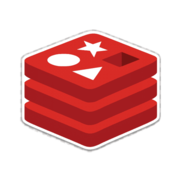
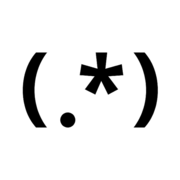
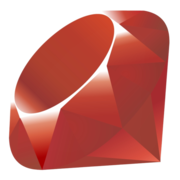
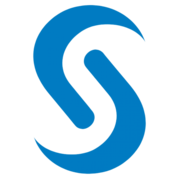
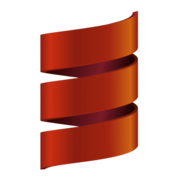
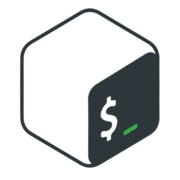
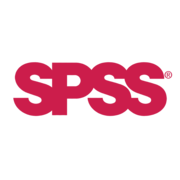
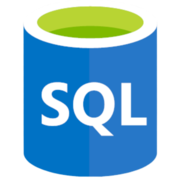
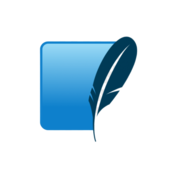
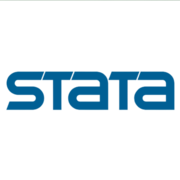
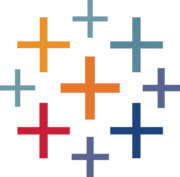
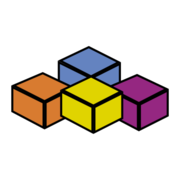