Prompt
Answer
To solve the given problem, we need to write a function in SQL that can handle the scenario where there is incorrect syntax near a certain keyword or code.
Here is the solution:
CREATE FUNCTION handle_syntax_error()
RETURNS VARCHAR(100)
AS
BEGIN
RETURN 'There is an incorrect syntax near the code.';
-- Or, you can raise an exception here if needed.
END;
Explanation:
- We create a function called handle_syntax_error using the
CREATE FUNCTION
statement. - The function has a return type of VARCHAR(100) which can be modified based on the specific requirements.
- Inside the function, we include the logic to handle the syntax error, in this case, returning a specific message.
- You can customize the message as per your requirements.
- If needed, you can raise an exception instead of returning a message by using the
THROW
statement.
Code Usage Example:
DECLARE @result VARCHAR(100);
BEGIN TRY
-- Your SQL code that may raise a syntax error
-- ...
-- ...
SET @result = 'Success';
END TRY
BEGIN CATCH
SET @result = dbo.handle_syntax_error();
END CATCH;
SELECT @result;
Explanation:
- We declare a variable @result of type VARCHAR(100).
- We wrap the code that may raise a syntax error inside a TRY block.
- If an error occurs, the control is passed to the CATCH block where we call the handle_syntax_error function to handle the error.
- If no error occurs, the code proceeds normally.
- We select the @result variable to see the output.
Note: This solution assumes you are using Microsoft SQL Server. The syntax may vary for different database systems.
Description
The SQL Syntax Error Handler solution showcases a function called handle_syntax_error
that can be used to handle syntax errors in SQL code. The function returns a message indicating that there is an incorrect syntax near a specific code. The solution also demonstrates how to use the function by wrapping the code that may raise a syntax error inside a TRY block and calling the function from the CATCH block to handle the error. By customizing the message and using this solution, developers can effectively handle syntax errors in their SQL code in a structured and controlled manner. Please note that the provided code example is specific to Microsoft SQL Server, and the syntax may vary for different database systems.
More Code Generators
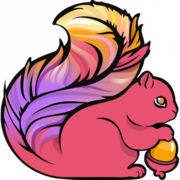
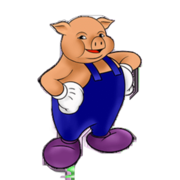
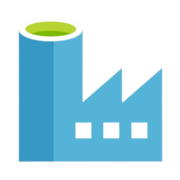
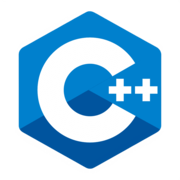
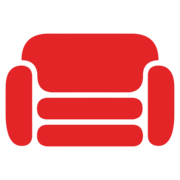
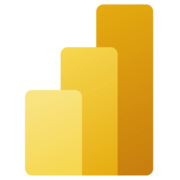
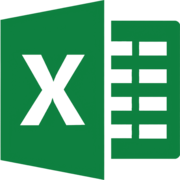
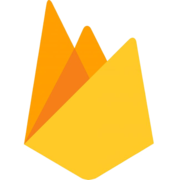
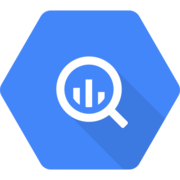
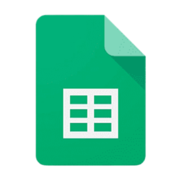
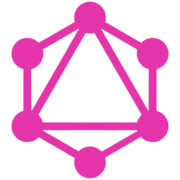
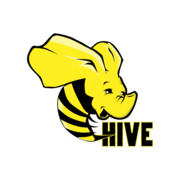
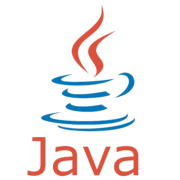
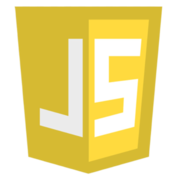
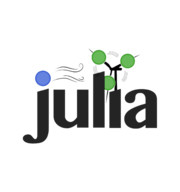
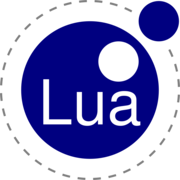
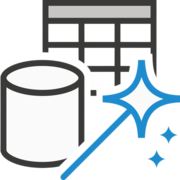
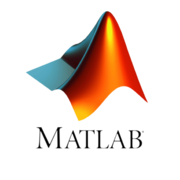
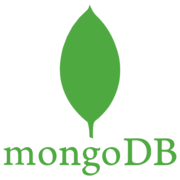
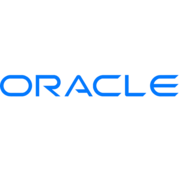
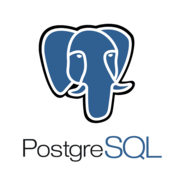
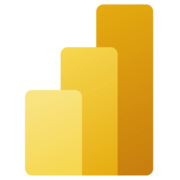
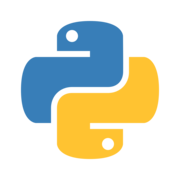
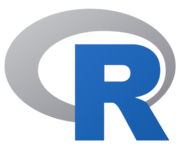
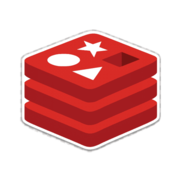
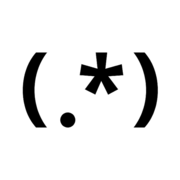
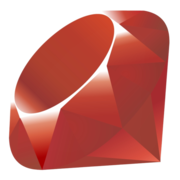
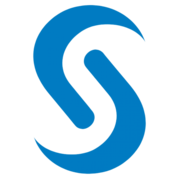
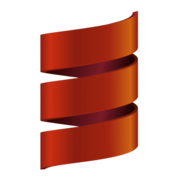
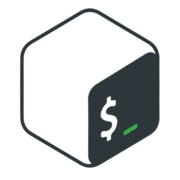
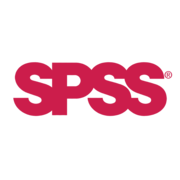
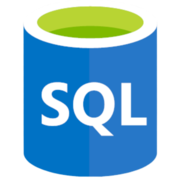
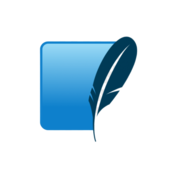
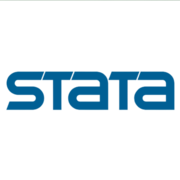
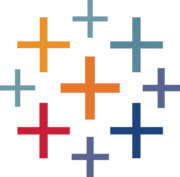
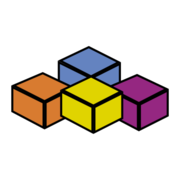