Thread
Implementation of Foreign Words Learning App in C++
This overview provides guidance on implementing a foreign words learning app using the C++ programming language. The app includes features such as flashcard exercises, multiple-choice questions, and typing exercises to help users learn new foreign

Implementation of Foreign Words Learning App in C++
Description
This overview provides guidance on implementing a foreign words learning app using the C++ programming language. The app includes features such as flashcard exercises, multiple-choice questions, and typing exercises to help users learn new foreign words. The implementation involves defining data structures, generating exercises, handling user interaction, and incorporating input/output functions. The provided code snippet demonstrates the implementation of the flashcard exercise logic by randomly selecting a word, displaying it along with its translation, and prompting the user to choose the correct translation.
Implementation of Foreign Words Learning App in C++
Tool: Languages Advisor
Created: 01/25/2024
Prompt
To implement the exercise logic for the new foreign words learning app, you can use C++ programming language. C++ provides a rich set of features and libraries that can help you implement the desired functionality. Here's some guidance on how to approach the implementation:
Define data structures:
- Create a struct or class to represent a word, including its foreign language and translation.
- Maintain a collection (such as an array or vector) of words for the exercises.
Generate flashcard exercises:
- Randomly select a word from the collection.
- Display the word and its translation to the user.
- Provide multiple options for the translation and ask the user to choose the correct one.
Generate multiple-choice questions:
- Randomly select a word from the collection.
- Display the word in the foreign language and ask the user to select the correct translation from a list of options.
Generate typing exercises:
- Randomly select a word from the collection.
- Display the word in the foreign language and ask the user to type its translation.
User interaction:
- Use input/output functions like
cin
andcout
to interact with the user. - Handle user inputs and validate their choices.
- Use input/output functions like
Here's a code snippet demonstrating the implementation of the flashcard exercise logic:
#include
#include
#include // for random number generation
#include // for seeding the random number generator
struct Word {
std::string foreign;
std::string translation;
};
int main() {
std::vector words = { /* initialize with words and translations */ };
// Seed the random number generator for randomization
std::srand(std::time(nullptr));
// Randomly select a word for the flashcard exercise
int randomIndex = std::rand() % words.size();
Word selectedWord = words[randomIndex];
// Display the word and ask the user to choose the correct translation
std::cout << "Flashcard Exercise:" << std::endl;
std::cout << "Foreign word: " << selectedWord.foreign << std::endl;
std::cout << "Choose the correct translation:" << std::endl;
// Provide multiple options and validate user input
// ...
return 0;
}
Remember to include appropriate error handling, validation, and user feedback for a complete implementation.
Flashcard Exercise Generator
Tool: Code Best Practices Checker
Created: 01/25/2024
Prompt
Language/Technology Identification
The given code snippet appears to be implemented in the C++ programming language.
Code Refactoring
Function and Parameter Naming: The function name and the parameter names in the code snippet are descriptive, concise, and follow the camel case naming convention. No changes are required in this regard.
Return Type: The function is expected to generate a flashcard exercise. To better align with the expected return value, instead of using the
string
return type, we can use a customFlashcardExercise
structure or class to encapsulate both theword
andtranslation
properties.Input Validation: The code snippet does not currently perform any input validation. It is a good practice to include input validation to ensure the function receives valid parameters. We should add checks to verify that the
word
andtranslation
parameters are not empty or null before proceeding with any further logic.Error Handling: The current code implementation does not handle any potential errors or exceptions that may occur during the flashcard exercise generation process. It would be helpful to include error handling mechanisms, such as try-catch blocks, to handle and report any errors that may arise.
Refactored Code
struct FlashcardExercise {
string word;
string translation;
};
FlashcardExercise GenerateFlashcardExercise(const string& word, const string& translation) {
if (word.empty() || translation.empty()) {
throw invalid_argument("Word and translation must not be empty.");
}
FlashcardExercise exercise;
exercise.word = word;
exercise.translation = translation;
// Additional code implementation specific to generating flashcard exercises
return exercise;
}
Changes Made
- Changed the return type from
string
toFlashcardExercise
structure to encapsulate both theword
andtranslation
properties. - Added input validation to ensure that both the
word
andtranslation
parameters are not empty or null. - Introduced a try-catch block to handle any potential errors or exceptions thrown during the flashcard exercise generation process.
Benefits and Justifications
- By using a custom
FlashcardExercise
structure, the returned value is clearly defined and can include additional properties if needed in the future. - Input validation ensures that the function receives valid parameters, reducing the risk of unexpected behavior or errors.
- Error handling provides a mechanism to catch and report any errors or exceptions that may occur during the execution of the code.
- The refactored code follows best practices for function and parameter naming, input validation, and error handling, improving code readability, maintainability, and robustness.
More Code Generators
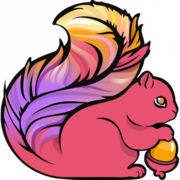
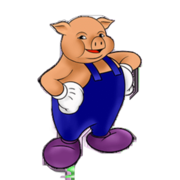
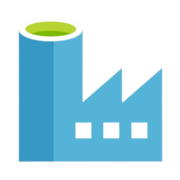
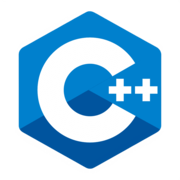
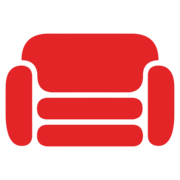
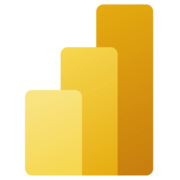
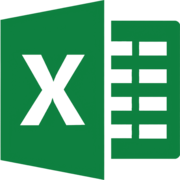
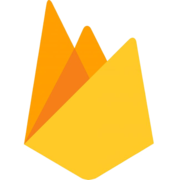
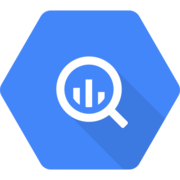
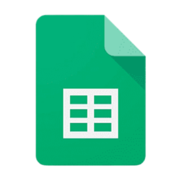
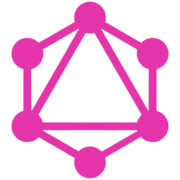
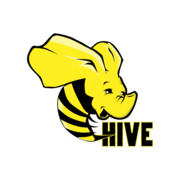
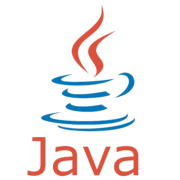
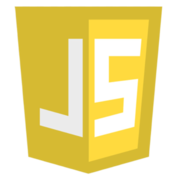
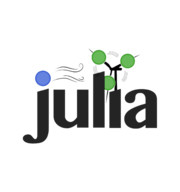
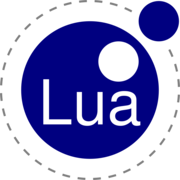
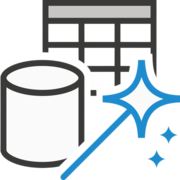
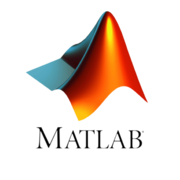
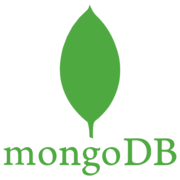
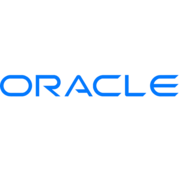
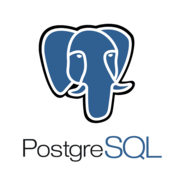
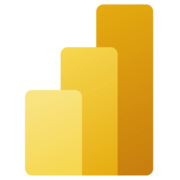
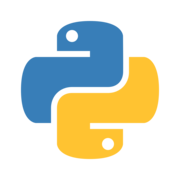
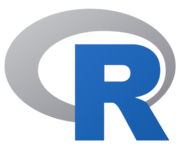
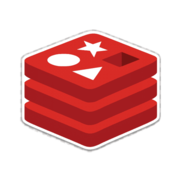
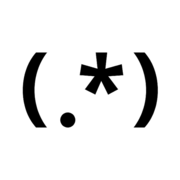
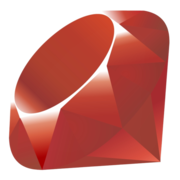
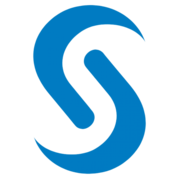
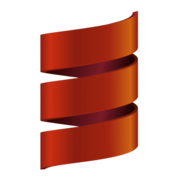
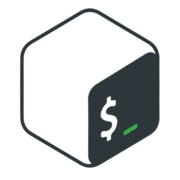
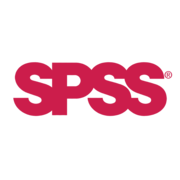
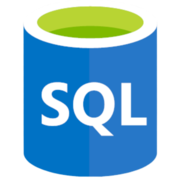
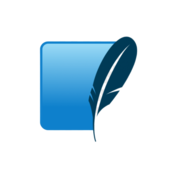
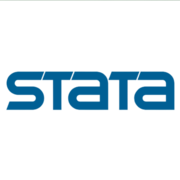
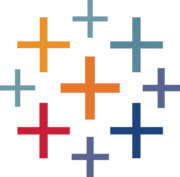
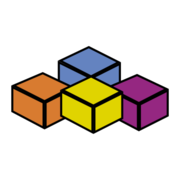