VBA Mastery Guide
Description
This thread provides a comprehensive exploration of essential VBA topics, including key syntax elements, common functions and custom functions in VBA. It also covers creating custom functions and demonstrates specific examples such as adding two numbers, generating random numbers, and retrieving the last non-empty cell in Excel using VBA.
Key VBA Syntax Elements
Tool: Languages Advisor
Created: 01/26/2024
Prompt
Main Syntax in VBA
When using VBA (Visual Basic for Applications), there are several key syntax elements you need to understand. These elements include variable declarations, loops, conditional statements, and subroutines/functions. Here's a summary of each element:
Variables and Data Types:
- Declare variables using the
Dim
statement, followed by the variable name and optional data type. - Common data types include
Integer
,Long
,Double
,String
,Date
, andBoolean
. - Example:
Dim myVariable As String
- Declare variables using the
Loops:
- The
For...Next
loop is used to execute a block of code for a specified number of times. - The
Do...Loop
loop is used when you want to repeat a block of code until a certain condition is met. - Example:
For i = 1 To 10 ' Code to be executed Next i
- The
Conditional Statements:
- The
If...Then...Else
statement is used to execute different blocks of code based on a condition. - The
Select Case
statement is used for multiple branching conditions. - Example:
If condition Then ' Code to be executed Else ' Code to be executed End If
- The
Subroutines and Functions:
- Subroutines are used for grouping code that performs a specific task.
- Functions return a value and can be used in expressions.
- Example:
Sub MySub() ' Code to be executed End Sub Function MyFunction() As Integer ' Code to be executed End Function
Objects and Methods:
- VBA is often used to automate tasks in other applications, such as Excel or Access.
- Objects represent elements within these applications, and methods are actions that can be performed on objects.
- Example:
' Excel example Dim wb As Workbook Set wb = Workbooks.Open("C:\MyWorkbook.xlsx") wb.Save ' Access example Dim db As Database Set db = CurrentDb db.Execute "SELECT * FROM Employees"
By understanding and mastering these key syntax elements in VBA, you'll be able to write efficient and effective code to automate tasks and analyze data.
Common Functions in VBA Overview
Tool: Languages Advisor
Created: 01/26/2024
Prompt
There are several common functions in VBA that can be used across many code bases. Here are a few essential functions:
MsgBox
: Used to display messages to the user. It allows you to provide information, ask questions, or prompt for input.InputBox
: Similar toMsgBox
, but specifically used to prompt the user for input. It can be used to get values or text from the user.Range
: Used to refer to cells or ranges in Excel. It is commonly used for reading or writing data to cells.Cells
: Similar toRange
, but it allows you to refer to cells using row and column numbers directly.IF
statement: Used for conditional branching. It allows you to execute different blocks of code based on a condition.For
loop: Used to iterate over a specified number of times or loop through items in a collection.Do
loop: Used for repetitive tasks that may have an unknown number of iterations. It can be terminated based on a condition.Sub
andFunction
: Used to define reusable code blocks.Sub
is used for procedures that don't return a value, whileFunction
is used for procedures that return a value.Workbook
andWorksheet
objects: Used to interact with workbooks and worksheets in Excel. They provide properties and methods to manipulate data and perform various tasks.Error handling
: Used to handle runtime errors gracefully. It includesOn Error Resume Next
,On Error GoTo
, andErr
object.
These are just a few essential functions, but there are many more available in VBA. It's important to familiarize yourself with the VBA documentation and explore additional functions based on your specific needs.
Common Custom Functions in VBA
Tool: Languages Advisor
Created: 01/26/2024
Prompt
There are a variety of common custom functions you can create and reuse across multiple VBA projects. Here are a few examples:
Calculate the Age:
- This function calculates the age based on a given birthdate and current date.
- Language: VBA
- Code snippet:
Function CalculateAge(ByVal birthdate As Date) As Integer CalculateAge = DateDiff("yyyy", birthdate, Date) End Function
Concatenate Range Values:
- This function combines the values of a range into a single string.
- Language: VBA
- Code snippet:
Function ConcatenateRange(ByVal rng As Range) As String Dim cell As Range For Each cell In rng ConcatenateRange = ConcatenateRange & cell.Value & " " Next cell End Function
Generate Random Number:
- This function generates a random number within a specified range.
- Language: VBA
- Code snippet:
Function GenerateRandomNumber(ByVal min As Double, ByVal max As Double) As Double Randomize GenerateRandomNumber = WorksheetFunction.RandBetween(min, max) End Function
Get Last Non-Empty Cell:
- This function retrieves the value of the last non-empty cell in a column.
- Language: VBA
- Code snippet:
Function GetLastNonEmptyCell(ByVal columnRange As Range) As Range Dim lastCell As Range On Error Resume Next Set lastCell = columnRange.SpecialCells(xlCellTypeConstants).Cells(columnRange.Rows.Count) On Error GoTo 0 Set GetLastNonEmptyCell = lastCell End Function
These are just a few examples, and the functions you can create are limitless. Consider your specific needs and create custom functions that can be reused across multiple projects to save time and improve efficiency.
Creating Custom Functions in VBA
Tool: Languages Advisor
Created: 01/26/2024
Prompt
To create a custom function in VBA, you can follow these steps:
- Open the VBA Editor by pressing
Alt + F11
. - In the VBA Editor, insert a new module by clicking
Insert
and selectingModule
. - In the module, define your custom function with the
Function
keyword, followed by the function name and any input parameters. - Write the code for your custom function. This can include any VBA code or built-in VBA functions.
- Assign the return value of the function using the function name followed by an equal sign and the desired value.
- Finally, save the VBA module and close the VBA Editor.
Here's an example that creates a custom function called AddTwoNumbers
that adds two input parameters and returns the result:
Function AddTwoNumbers(num1 As Integer, num2 As Integer) As Integer
AddTwoNumbers = num1 + num2
End Function
You can then call this custom function from an Excel cell like any other built-in function. For example, if you enter =AddTwoNumbers(5, 3)
in a cell, it will display 8
.
Remember to save your workbook as a macro-enabled file format, such as .xlsm, to preserve the VBA code.
VB Function for Adding Two Numbers
Tool: Code Explainer
Created: 01/26/2024
Prompt
Code Analysis: The provided code is written in Visual Basic (VB) and defines a function called "AddTwoNumbers" that takes two integer parameters, "num1" and "num2". The function returns an integer value, which is the sum of "num1" and "num2".
Simplified Explanation: The code defines a function that adds two numbers together and returns the result.
Elaborate on Key Concepts:
- Function: In VB, a function is a block of code that performs a specific task and returns a value. In this code, the function "AddTwoNumbers" takes two integer numbers as input and returns their sum.
- Parameters: Parameters are variables passed to a function that allow it to accept input values from the caller. In this code, "num1" and "num2" are the parameters of the function, representing the two numbers to be added.
- Return Value: The "AddTwoNumbers" function returns an integer value, which is the sum of "num1" and "num2". The "AddTwoNumbers = num1 + num2" line assigns the sum to the function name, which will be returned as the result.
Provide Additional Examples: Here is an example of how you can use the "AddTwoNumbers" function:
Dim result As Integer
result = AddTwoNumbers(5, 3)
In this example, the function is called with the numbers 5 and 3 as arguments. The returned value, which is the sum of 5 and 3 (i.e., 8), is assigned to the "result" variable.
VBA Code - GenerateRandomNumber
Tool: Code Explainer
Created: 01/26/2024
Prompt
Code Analysis:
The provided code is written in VBA (Visual Basic for Applications), which is a programming language used for automating tasks in Microsoft Office applications. It defines a function named "GenerateRandomNumber" that takes in two parameters: "min" and "max" of type Double. The purpose of this function is to generate a random number between the "min" and "max" values.
Simplified Explanation:
The code defines a function "GenerateRandomNumber" that generates a random number between the given minimum and maximum values.
Here's how the code works:
- The "Randomize" statement is used to initialize the random number generator.
- The "WorksheetFunction.RandBetween(min, max)" statement generates a random whole number between the "min" and "max" values.
- The generated random number is assigned to the function name "GenerateRandomNumber".
- Finally, the function returns the generated random number.
Elaborate on Key Concepts:
Randomize: The "Randomize" statement initializes the random number generator. It ensures that each time the function is called, a different sequence of random numbers is generated.
WorksheetFunction.RandBetween: This function is used to generate a random whole number between the specified minimum and maximum values. It is a built-in function provided by the WorksheetFunction class in VBA.
Provide Additional Examples:
Here's an example of how you can use the "GenerateRandomNumber" function:
Sub ExampleUsage()
Dim randomNumber As Double
' Generate a random number between 0 and 10
randomNumber = GenerateRandomNumber(0, 10)
' Display the generated random number
MsgBox "Random Number: " & randomNumber
End Sub
Conclusion:
The provided code demonstrates a simple function in VBA that generates a random number between the given minimum and maximum values. Understanding this code can be useful when you need to generate random numbers within a specific range for various purposes, such as simulations, games, or data analysis.
VBA Function - GetLastNonEmptyCell
Tool: Code Explainer
Created: 01/26/2024
Prompt
Code Explanation
The provided code is written in VBA (Visual Basic for Applications) language and defines a function called GetLastNonEmptyCell
. This function takes a columnRange
parameter of type Range
and returns the last non-empty cell in that range.
Here is a breakdown of the code:
Function GetLastNonEmptyCell(ByVal columnRange As Range) As Range
- This line declares a function named
GetLastNonEmptyCell
that takes a single parametercolumnRange
of typeRange
. - The function returns a value of type
Range
.
Dim lastCell As Range
- This line declares a variable
lastCell
of typeRange
.
On Error Resume Next
- This line enables error handling and instructs the code to continue execution even if an error occurs.
Set lastCell = columnRange.SpecialCells(xlCellTypeConstants).Cells(columnRange.Rows.Count)
- This line assigns the last non-empty cell in the
columnRange
to thelastCell
variable. - The
SpecialCells
method is used to filter for constant (non-formula) cells in thecolumnRange
. - The
Cells
property is used to get the cell with the highest row index (corresponding to the last non-empty cell in the range).
On Error GoTo 0
- This line disables error handling, so any subsequent errors will trigger an error message or break the code execution.
Set GetLastNonEmptyCell = lastCell
- This line assigns the value of
lastCell
to the function nameGetLastNonEmptyCell
, which is of typeRange
. - This effectively returns the last non-empty cell in the specified
columnRange
.
Key Concepts
- On Error Resume Next: This statement enables error handling and allows the code to continue execution even if an error occurs. It is useful in cases where errors are expected and can be handled gracefully.
- SpecialCells: This method is used to filter cells in a range based on specific criteria. In this code,
SpecialCells(xlCellTypeConstants)
filters for constant (non-formula) cells in thecolumnRange
. - Cells: This property is used to access a specific cell within a range. In this code,
Cells(columnRange.Rows.Count)
retrieves the cell with the highest row index in thecolumnRange
, which corresponds to the last non-empty cell in the range.
Example
Here's an example of how to use the GetLastNonEmptyCell
function:
Dim lastCell As Range
Set lastCell = GetLastNonEmptyCell(Range("A1:A10"))
If Not lastCell Is Nothing Then
MsgBox "Last non-empty cell: " & lastCell.Address
Else
MsgBox "No non-empty cells found."
End If
In this example, GetLastNonEmptyCell
is called with a range object Range("A1:A10")
, and the result is stored in lastCell
. It then checks if lastCell
is not empty and displays a message box with the address of the last non-empty cell, or a message indicating no non-empty cells were found.
More Code Generators
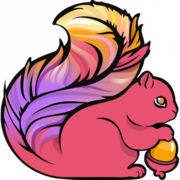
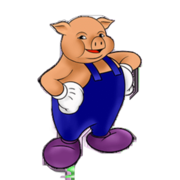
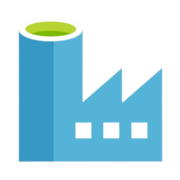
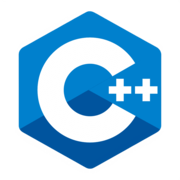
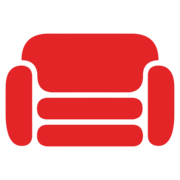
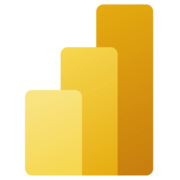
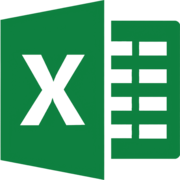
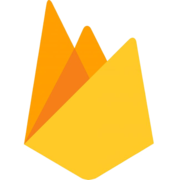
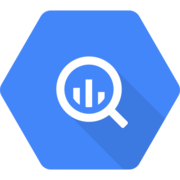
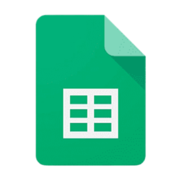
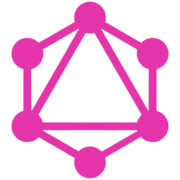
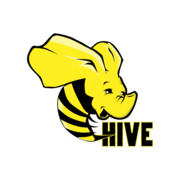
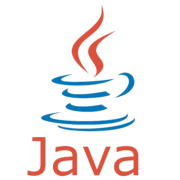
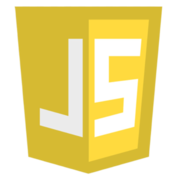
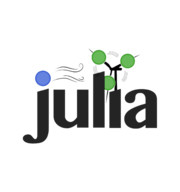
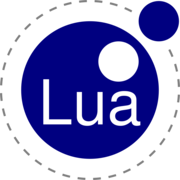
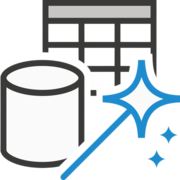
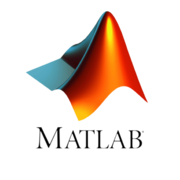
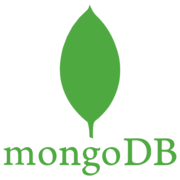
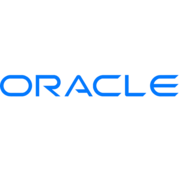
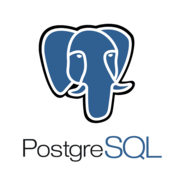
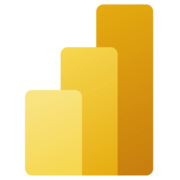
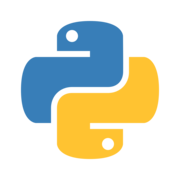
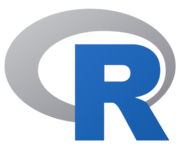
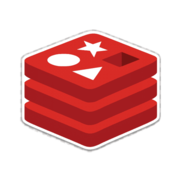
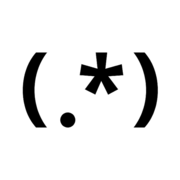
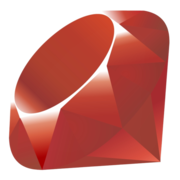
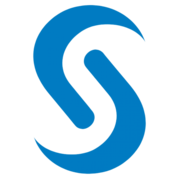
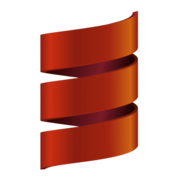
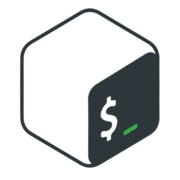
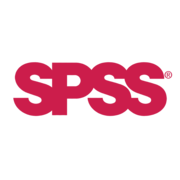
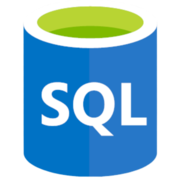
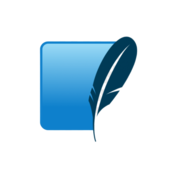
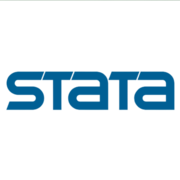
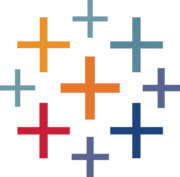
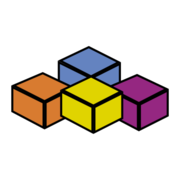