Techniques for User-Friendly Forms and
Description
This thread explores various techniques for creating user-friendly forms and interfaces, with a focus on topics such as creating user forms in Excel, event procedures for controls in VBA user forms, exploring VBA event procedures for Excel, VBA command button click event, VBA text box key press event, and best practices for designing user forms.
Techniques for Creating User-Friendly Forms and Interfaces using
Tool: Languages Advisor
Created: 01/10/2024
Prompt
When creating user forms and interfaces using VBA, consider the following techniques to make them user-friendly:
1. Consistent Layout and Design
Maintain a consistent layout and design throughout the form. Use align and distribute options to organize controls neatly. Ensure that labels, input fields, and buttons are aligned and evenly spaced.
2. Clear and Descriptive Labels
Use clear and descriptive labels for controls to guide users. Labels should be concise yet informative, helping users understand the purpose and expected input of each control.
3. Error Handling
Implement error handling to anticipate and handle user errors gracefully. Use VBA's error handling features, such as On Error Resume Next
and On Error GoTo
, to catch and handle errors appropriately. Provide informative error messages to guide users in correcting their input.
4. Input Validation
Validate user input to ensure data integrity. Use techniques like data validation rules and input masks to restrict input to specified formats or ranges. Verify user input against expected values and handle invalid input by displaying appropriate error messages.
5. Keyboard Shortcuts and Mnemonics
Assign keyboard shortcuts and mnemonics to controls to enhance usability. This allows users to quickly navigate and interact with the form using the keyboard, saving time and improving efficiency.
6. Default Focus
Set the default focus to the appropriate control when the form is opened. This ensures that users can start entering data immediately without having to manually select an input field.
7. Intuitive Navigation
Implement intuitive navigation within the form. Use tab order and shortcut keys to allow users to move between controls smoothly. Provide visually distinct visual cues, such as highlighting or changing the appearance of the active control, to indicate the current focus.
8. Help and Tooltips
Include help text or tooltips to provide additional guidance and clarification for users. Help text can be displayed as a separate section within the form or as tooltips that appear when users hover over specific controls.
9. Context-Sensitive Assistance
Implement context-sensitive assistance to guide users based on the specific task or context. Display relevant instructions or tips dynamically based on the user's current action or input.
10. Testing and Feedback
Thoroughly test the form to ensure smooth functionality and usability. Gather feedback from users to identify areas for improvement and make necessary adjustments to enhance the user experience.
By applying these techniques, you can create user-friendly forms and interfaces that enhance usability and efficiency, leading to a positive user experience.
How to Create User Forms in Excel with
Tool: Tools Advisor
Created: 01/10/2024
Prompt
Sure! To create user forms in Excel, you can use the VBA (Visual Basic for Applications) programming language. Here's a step-by-step guide:
Step 1: Enable the Developer tab
- Go to the "File" tab -> "Options" -> "Customize Ribbon".
- Check the box for "Developer" in the right column, then click "OK".
Step 2: Open the Visual Basic Editor
- Click on the "Developer" tab in the Excel ribbon.
- Click on the "Visual Basic" button to open the Visual Basic Editor.
Step 3: Insert a User Form
- In the Visual Basic Editor, navigate to the "Insert" menu and click on "UserForm".
- A new user form will appear in the editor window.
Step 4: Design the User Form
- In the UserForm design mode, you can add controls (like labels, text boxes, buttons, etc.) from the toolbox to the form.
- Customize the appearance and layout of the user form by resizing and positioning the controls.
- Set properties for each control, such as captions, names, and event handlers.
Step 5: Write Code for the User Form
- Double-click on any control to access its code window.
- Write VBA code within event procedures to define the actions to be performed when events occur (e.g., button clicks, data entry, etc.).
- You can use VBA code to interact with worksheets, manipulate data, perform calculations, etc.
Step 6: Test the User Form
- Exit the UserForm design mode by clicking on the "Exit Design Mode" button in the toolbar.
- Save your Excel workbook.
- Go back to the Excel workbook and click on the form control (e.g., button) you created.
- The user form will appear, and the code you wrote will be executed when events are triggered.
Note: To view and edit the code associated with the form, go back to the Visual Basic Editor and find the code module for the UserForm.
You can use VBA code to create user-friendly and interactive interfaces in Excel. There are additional features and techniques you can use to enhance the functionality of your user forms, such as data validation, error handling, and advanced controls.
Let me know if you need any further assistance!
Event Procedures for Controls in VBA User
Tool: Skills Advisor
Created: 01/10/2024
Prompt
In VBA, we can define actions for controls in user forms by using event procedures. These event procedures are triggered by various events that occur when interacting with the controls in the user form. Some common events and event procedures that can be used in VBA to define actions for controls in user forms include:
Click Event: This event occurs when a control is clicked. It can be used to define actions when a button or any other control is clicked.
Private Sub CommandButton_Click() ' Code to be executed when the button is clicked End Sub
Change Event: This event occurs when the value of a control changes. It can be used to define actions when the value of a textbox or any other control is changed.
Private Sub TextBox_Change() ' Code to be executed when the textbox value changes End Sub
Activate Event: This event occurs when a control or a user form becomes the active control or form. It can be used to define actions when a control or user form is activated.
Private Sub UserForm_Activate() ' Code to be executed when the user form is activated End Sub
Close Event: This event occurs when a user form is closed. It can be used to define actions before or after the user form is closed.
Private Sub UserForm_QueryClose(Cancel As Integer, CloseMode As Integer) ' Code to be executed before the user form is closed End Sub
KeyPress Event: This event occurs when a key is pressed while a control has the focus. It can be used to define actions when a specific key or combination of keys is pressed.
Private Sub TextBox_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger) ' Code to be executed when a key is pressed in the textbox End Sub
These are just a few examples of the events and event procedures that can be used in VBA to define actions for controls in user forms. By leveraging these event procedures, you can create interactive user interfaces and automate tasks in your Excel workbooks.
Exploring VBA Event Procedures for Excel
Tool: Project Advisor
Created: 01/10/2024
Prompt
Project Description: The project aims to explore the use of event procedures in VBA to create interactive user interfaces and automate tasks in Excel workbooks. The main objective is to improve efficiency and user experience by automating repetitive tasks and enhancing the functionality of Excel workbooks through the use of VBA event procedures.
Recommended Structure: To successfully implement this project, it is recommended to follow the following structure:
Project Setup:
- Set up a development environment with the necessary tools, such as Microsoft Excel and the Visual Basic Editor (VBE).
- Create a new Excel workbook or open an existing one to work with.
Identify User Requirements:
- Understand the specific tasks and processes that need to be automated and enhanced using VBA event procedures.
- Identify the specific events (such as button clicks, worksheet changes, or workbook opening) that should trigger the desired actions.
Design User Interface:
- Create an intuitive and user-friendly interface using Excel's built-in controls (such as buttons, checkboxes, and dropdown lists) or custom forms.
- Map out the user interface elements to the corresponding VBA event procedures that will handle the desired actions.
Implement VBA Event Procedures:
- Write VBA code to handle the specified events and automate the desired tasks.
- Use the relevant event procedures, such as Worksheet_SelectionChange, Workbook_Open, or UserForm_Click, to trigger the actions based on user interactions or workbook events.
Test and Debug:
- Thoroughly test the functionality of the implemented VBA event procedures.
- Use debugging techniques, such as breakpoints and watch windows, to identify and fix any issues or errors in the code.
Documentation and Maintenance:
- Document the implemented VBA event procedures and their functionality for future reference and maintenance purposes.
- Ensure that the code is well-documented and easy to understand for future enhancements or modifications.
Training and User Support:
- Provide training materials and support documentation to help users understand and utilize the implemented VBA event procedures.
- Offer ongoing user support to address any questions or issues that arise during the project implementation or subsequent usage.
Recommended Technologies and Techniques:
- Use Microsoft Excel and the Visual Basic Editor (VBE) for writing VBA code.
- Leverage Excel's built-in controls or create custom forms to design the user interface.
- Implement error handling techniques to handle potential runtime errors and provide informative error messages.
- Utilize debugging tools and techniques to identify and fix issues in the VBA code.
- Document the implemented VBA event procedures thoroughly for future reference and maintenance.
By following this structured approach, you can effectively use event procedures in VBA to create interactive user interfaces and automate tasks in Excel workbooks, leading to improved efficiency and enhanced functionality for end-users.
VBA TextBox KeyPress Event
Tool: Code Explainer
Created: 01/10/2024
Prompt
Code Analysis:
The provided code snippet is written in VBA (Visual Basic for Applications) and defines a Private Sub
procedure named TextBox_KeyPress
. This procedure is an event handler that is executed when a key is pressed on a text box control.
Simplified Explanation:
The Private Sub TextBox_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
code defines a procedure that handles the event when a key is pressed in a text box control. The code inside this procedure will be executed whenever a key is pressed in the text box.
Elaborate on Key Concepts:
Private Sub
: This keyword combination is used to define a procedure that is accessible only within its own module or class. It cannot be accessed or called from outside the module or class it is defined in.TextBox_KeyPress
: This is the name of the procedure. It is an event handler that is called when a key is pressed in a text box control.KeyAscii
: This parameter represents the key code of the pressed key. It is declared asMSForms.ReturnInteger
which means it is an integer value returned by the key press event.MSForms.ReturnInteger
: This is a data type that represents an integer value returned from a subroutine or function associated with a Microsoft Forms object.
Provide Additional Examples:
Here is an example that demonstrates the usage of the TextBox_KeyPress
event handler in VBA:
Private Sub TextBox1_KeyPress(ByVal KeyAscii As MSForms.ReturnInteger)
If KeyAscii = 13 Then
MsgBox "Enter key pressed!"
End If
End Sub
In this example, the code checks if the key code of the pressed key is equal to 13 (which represents the Enter key). If it is, a message box with the text "Enter key pressed!" is displayed.
Best Practices for Designing Userforms in
Tool: Languages Advisor
Created: 01/10/2024
Prompt
When designing a userform in VBA, there are several best practices you can follow to create a user-friendly and efficient interface. Here are some key points to consider:
Use a clear and consistent layout: Arrange the controls on the userform in a logical and organized manner. Use consistent spacing, alignment, and font styles to ensure a professional and polished appearance.
Keep it simple: Avoid cluttering the userform with too many controls or unnecessary information. Focus on the essential elements and prioritize usability and clarity.
Utilize labels and captions: Clearly label each control with descriptive text to provide context and instructions to the user. Captions should be concise but informative.
Enable tab navigation: Set the
TabKeyBehavior
property of controls toTrue
to allow users to navigate between controls using the tab key. This enhances usability and accessibility.Validate user input: Implement appropriate data validation checks to ensure that users enter valid data into the userform. Use event procedures such as
BeforeUpdate
orValidation
to validate user input.Provide feedback and error handling: Display informative messages or use colored labels to provide feedback to the user about the success or failure of their actions. Implement error handling routines to handle any unexpected errors and provide meaningful error messages.
Use appropriate control types: Select the most appropriate control types for the data entry or retrieval tasks. For example, use a text box for text input, a combo box for selecting from predefined options, and a list box for displaying a list of items.
Optimize performance: Minimize the load time of the userform by loading data and populating controls only when necessary. Avoid unnecessary calculations or complex operations in the userform's code.
Consistent naming convention: Use a consistent and meaningful naming convention for controls, variables, and procedures to improve code readability and maintainability. Consider using prefixes or suffixes to indicate the control type (e.g., txt for text box, cbo for combo box).
Test and debug: Thoroughly test the userform to ensure that it functions as expected in different scenarios. Use breakpoints and debug techniques to identify and fix any issues.
Remember, these best practices are meant to guide you, but you should also consider the specific requirements and preferences of your users when designing a userform.
More Code Generators
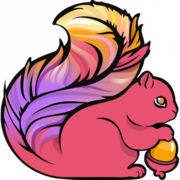
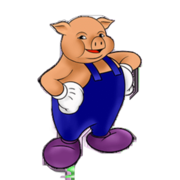
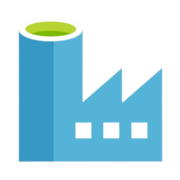
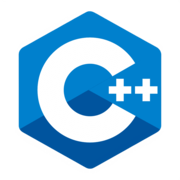
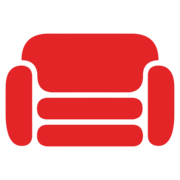
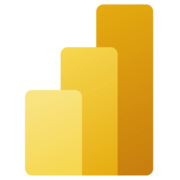
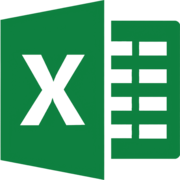
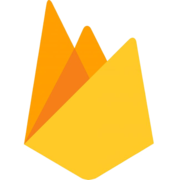
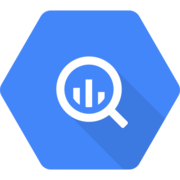
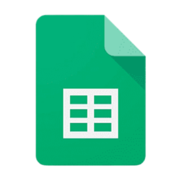
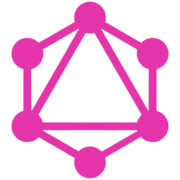
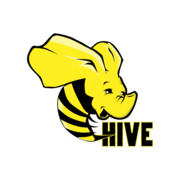
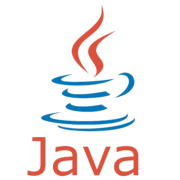
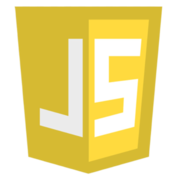
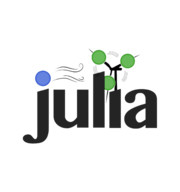
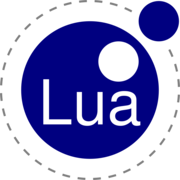
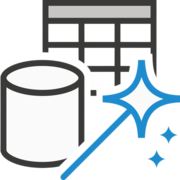
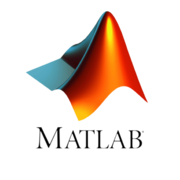
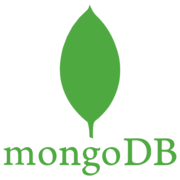
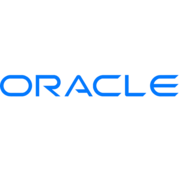
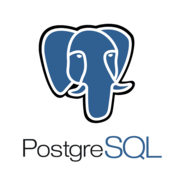
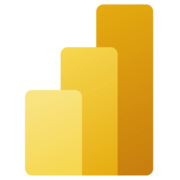
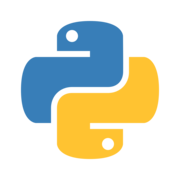
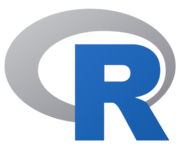
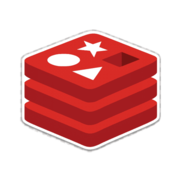
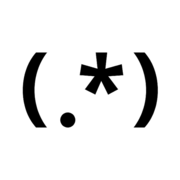
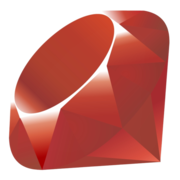
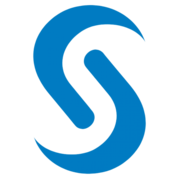
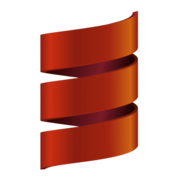
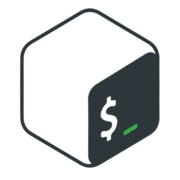
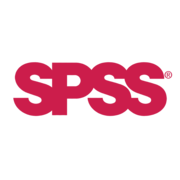
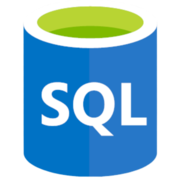
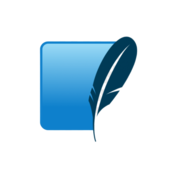
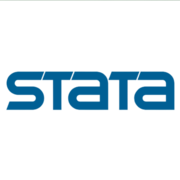
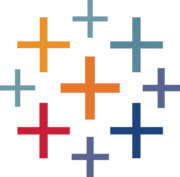
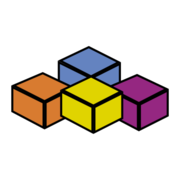